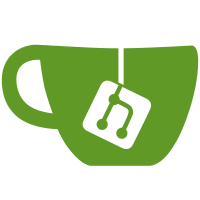
11 changed files with 104 additions and 88 deletions
@ -0,0 +1,42 @@ |
|||
#include "ChatDataStore.h" |
|||
|
|||
ChatDataStore* ChatDataStore::getInstance() |
|||
{ |
|||
if(!ChatDataStore::instanced) |
|||
{ |
|||
ChatDataStore::instanced = true; |
|||
ChatDataStore::instance = new ChatDataStore(); |
|||
} |
|||
|
|||
return ChatDataStore::instance; |
|||
} |
|||
|
|||
void ChatDataStore::clear() |
|||
{ |
|||
this->data.clear(); |
|||
} |
|||
|
|||
|
|||
void ChatDataStore::setData(QString key, ChatItem value) |
|||
{ |
|||
this->data[key] = value; |
|||
} |
|||
|
|||
ChatItem ChatDataStore::getData(QString key) |
|||
{ |
|||
return this->data[key]; |
|||
} |
|||
|
|||
QString ChatDataStore::dump() |
|||
{ |
|||
return ""; |
|||
} |
|||
|
|||
std::map<QString, ChatItem> ChatDataStore::getAllRawChatItems() |
|||
{ |
|||
return this->data; |
|||
} |
|||
|
|||
|
|||
ChatDataStore* ChatDataStore::instance = nullptr; |
|||
bool ChatDataStore::instanced = false; |
@ -0,0 +1,11 @@ |
|||
#include "DataStore.h" |
|||
|
|||
SietchDataStore* DataStore::getSietchDataStore() |
|||
{ |
|||
return SietchDataStore::getInstance(); |
|||
} |
|||
|
|||
ChatDataStore* DataStore::getChatDataStore() |
|||
{ |
|||
return ChatDataStore::getInstance(); |
|||
} |
@ -0,0 +1,35 @@ |
|||
#include "SietchDataStore.h" |
|||
|
|||
SietchDataStore* SietchDataStore::getInstance() |
|||
{ |
|||
if(!SietchDataStore::instanced) |
|||
{ |
|||
SietchDataStore::instanced = true; |
|||
SietchDataStore::instance = new SietchDataStore(); |
|||
} |
|||
|
|||
return SietchDataStore::instance; |
|||
} |
|||
|
|||
void SietchDataStore::clear() |
|||
{ |
|||
this->data.clear(); |
|||
} |
|||
|
|||
void SietchDataStore::setData(QString key, QString value) |
|||
{ |
|||
this->data[key] = value; |
|||
} |
|||
|
|||
QString SietchDataStore::getData(QString key) |
|||
{ |
|||
return this->data[key]; |
|||
} |
|||
|
|||
QString SietchDataStore::dump() |
|||
{ |
|||
return ""; |
|||
} |
|||
|
|||
SietchDataStore* SietchDataStore::instance = nullptr; |
|||
bool SietchDataStore::instanced = false; |
Loading…
Reference in new issue