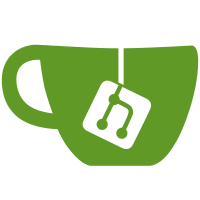
14 changed files with 183 additions and 1 deletions
@ -0,0 +1,35 @@ |
|||
b ContactDataStore::dump() |
|||
r |
|||
n |
|||
q |
|||
b ContactDataStore::dump() |
|||
r |
|||
n |
|||
c |
|||
./build.sh |
|||
$(./build.sh) |
|||
$(./build.sh) |
|||
q |
|||
r |
|||
q |
|||
r |
|||
b ContactDataStore::dump() |
|||
r |
|||
n |
|||
b ContactItem::toJson() |
|||
r |
|||
c |
|||
n |
|||
q |
|||
b ContactItem::toJson() |
|||
r |
|||
n |
|||
c |
|||
c |
|||
c |
|||
c |
|||
c |
|||
c |
|||
c |
|||
c |
|||
q |
@ -0,0 +1,2 @@ |
|||
break ContactItem::toJson() |
|||
|
@ -0,0 +1,53 @@ |
|||
// Copyright 2019-2020 The Hush developers
|
|||
// GPLv3
|
|||
|
|||
#include "ContactDataStore.h" |
|||
#include <string> |
|||
|
|||
ContactDataStore* ContactDataStore::getInstance() |
|||
{ |
|||
if(!ContactDataStore::instanced) |
|||
{ |
|||
ContactDataStore::instanced = true; |
|||
ContactDataStore::instance = new ContactDataStore(); |
|||
} |
|||
|
|||
return ContactDataStore::instance; |
|||
} |
|||
|
|||
void ContactDataStore::clear() |
|||
{ |
|||
this->data.clear(); |
|||
} |
|||
|
|||
|
|||
void ContactDataStore::setData(QString key, ContactItem value) |
|||
{ |
|||
this->data[key] = value; |
|||
} |
|||
|
|||
ContactItem ContactDataStore::getData(QString key) |
|||
{ |
|||
return this->data[key]; |
|||
} |
|||
|
|||
QString ContactDataStore::dump() |
|||
{ |
|||
json contacts; |
|||
contacts["count"] = this->data.size(); |
|||
json j = {}; |
|||
for (auto &c: this->data) |
|||
{ |
|||
qDebug() << c.second.toQTString(); |
|||
c.second.toJson(); |
|||
j.push_back(c.second.toJson()); |
|||
} |
|||
contacts["contacts"] = j; |
|||
|
|||
std::string dump = contacts.dump(4); |
|||
qDebug() << dump.c_str(); |
|||
return ""; |
|||
} |
|||
|
|||
ContactDataStore* ContactDataStore::instance = nullptr; |
|||
bool ContactDataStore::instanced = false; |
@ -0,0 +1,34 @@ |
|||
#ifndef CONTACTDATASTORE_H |
|||
#define CONTACTDATASTORE_H |
|||
#include "../Model/ContactItem.h" |
|||
#include <string> |
|||
using json = nlohmann::json; |
|||
|
|||
class ContactDataStore |
|||
{ |
|||
private: |
|||
static bool instanced; |
|||
static ContactDataStore* instance; |
|||
std::map<QString, ContactItem> data; |
|||
ContactDataStore() |
|||
{ |
|||
|
|||
} |
|||
|
|||
public: |
|||
static ContactDataStore* getInstance(); |
|||
void clear(); |
|||
void setData(QString key, ContactItem value); |
|||
ContactItem getData(QString key); |
|||
QString dump(); |
|||
|
|||
~ContactDataStore() |
|||
{ |
|||
ContactDataStore::instanced = false; |
|||
ContactDataStore::instance = nullptr; |
|||
} |
|||
}; |
|||
|
|||
|
|||
|
|||
#endif |
Loading…
Reference in new issue