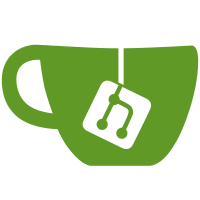
committed by
Daniel Cousens
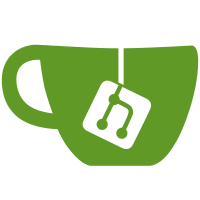
5 changed files with 74 additions and 19 deletions
@ -0,0 +1,17 @@ |
|||
var typeforce = require('./') |
|||
|
|||
// async wrapper
|
|||
function tfAsync (type, value, strict, callback) { |
|||
// default to falsy strict if using shorthand overload
|
|||
if (typeof strict === 'function') return tfAsync(type, value, false, strict) |
|||
|
|||
try { |
|||
typeforce(type, value, strict) |
|||
} catch (e) { |
|||
return callback(e) |
|||
} |
|||
|
|||
callback() |
|||
} |
|||
|
|||
module.exports = Object.assign(tfAsync, typeforce) |
@ -0,0 +1,12 @@ |
|||
var typeforce = require('./') |
|||
|
|||
function tfNoThrow (type, value, strict) { |
|||
try { |
|||
return typeforce(type, value, strict) |
|||
} catch (e) { |
|||
tfNoThrow.error = e |
|||
return false |
|||
} |
|||
} |
|||
|
|||
module.exports = Object.assign(tfNoThrow, typeforce) |
Loading…
Reference in new issue