Browse Source
* Save created recurring info * Add to confirm dialog * Update RPI at confirm * Make singleton * Add history to recurring payments * USD/ZEC switch * Fix check state * recurring item serialization * Add recurring payments to file * Refactor * Address view model * Wire up dialog * Update windows installer logos * Store all payments in the store * Save table geometry * Add recurring payments view * Add deletion * Add recurring payment execution * Add donation address to address book * Add multiple payment handling * Disable recurring for multiple payments * Handle pay last * Handle pay all * Reomve frequency * Enable recurring payments only for testnet * For testing, allow payments in 5 min intervals * Fix request money amounts0.6.10
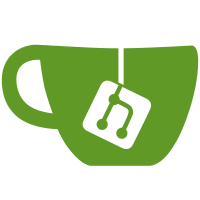
committed by
GitHub
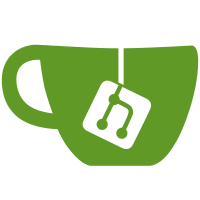
21 changed files with 1439 additions and 201 deletions
@ -0,0 +1,178 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<ui version="4.0"> |
|||
<class>RecurringPending</class> |
|||
<widget class="QDialog" name="RecurringPending"> |
|||
<property name="geometry"> |
|||
<rect> |
|||
<x>0</x> |
|||
<y>0</y> |
|||
<width>883</width> |
|||
<height>801</height> |
|||
</rect> |
|||
</property> |
|||
<property name="windowTitle"> |
|||
<string>Dialog</string> |
|||
</property> |
|||
<layout class="QGridLayout" name="gridLayout"> |
|||
<item row="14" column="0" colspan="2"> |
|||
<widget class="QLabel" name="label_5"> |
|||
<property name="text"> |
|||
<string>No payments will be processed. You can manually pay them from the Recurring Payments Dialog box</string> |
|||
</property> |
|||
<property name="wordWrap"> |
|||
<bool>true</bool> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="15" column="0" colspan="2"> |
|||
<widget class="Line" name="line_2"> |
|||
<property name="orientation"> |
|||
<enum>Qt::Horizontal</enum> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="4" column="0" colspan="2"> |
|||
<widget class="QLabel" name="lblSchedule"> |
|||
<property name="text"> |
|||
<string>Schedule</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="8" column="0" colspan="2"> |
|||
<widget class="QLabel" name="label_2"> |
|||
<property name="text"> |
|||
<string>How should ZecWallet proceed?</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="9" column="0" colspan="2"> |
|||
<widget class="QRadioButton" name="rAll"> |
|||
<property name="text"> |
|||
<string>Pay All in 1 Tx</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="12" column="0" colspan="2"> |
|||
<widget class="QLabel" name="label_4"> |
|||
<property name="text"> |
|||
<string>Only the latest pending payment will be processed. All previous pending payments will be skipped</string> |
|||
</property> |
|||
<property name="wordWrap"> |
|||
<bool>true</bool> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="11" column="0" colspan="2"> |
|||
<widget class="QRadioButton" name="rLast"> |
|||
<property name="text"> |
|||
<string>Pay Latest Only</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="13" column="0" colspan="2"> |
|||
<widget class="QRadioButton" name="rNone"> |
|||
<property name="text"> |
|||
<string>Pay None</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="6" column="0" colspan="2"> |
|||
<widget class="QTableView" name="tblPending"> |
|||
<attribute name="horizontalHeaderStretchLastSection"> |
|||
<bool>true</bool> |
|||
</attribute> |
|||
</widget> |
|||
</item> |
|||
<item row="7" column="0" colspan="2"> |
|||
<widget class="Line" name="line"> |
|||
<property name="orientation"> |
|||
<enum>Qt::Horizontal</enum> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="10" column="0" colspan="2"> |
|||
<widget class="QLabel" name="label_3"> |
|||
<property name="text"> |
|||
<string>All pending payments collected, added up and paid in a single transaction</string> |
|||
</property> |
|||
<property name="wordWrap"> |
|||
<bool>true</bool> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="16" column="0" colspan="2"> |
|||
<widget class="QDialogButtonBox" name="buttonBox"> |
|||
<property name="orientation"> |
|||
<enum>Qt::Horizontal</enum> |
|||
</property> |
|||
<property name="standardButtons"> |
|||
<set>QDialogButtonBox::Cancel|QDialogButtonBox::Ok</set> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="2" column="0" colspan="2"> |
|||
<widget class="QLabel" name="lblDesc"> |
|||
<property name="text"> |
|||
<string>Description</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="3" column="0" colspan="2"> |
|||
<widget class="QLabel" name="lblTo"> |
|||
<property name="text"> |
|||
<string>To</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="1" column="0" colspan="2"> |
|||
<widget class="Line" name="line_3"> |
|||
<property name="orientation"> |
|||
<enum>Qt::Horizontal</enum> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="0" column="0" colspan="2"> |
|||
<widget class="QLabel" name="label"> |
|||
<property name="text"> |
|||
<string>The following recurring payment has multiple payments pending</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
</layout> |
|||
</widget> |
|||
<resources/> |
|||
<connections> |
|||
<connection> |
|||
<sender>buttonBox</sender> |
|||
<signal>accepted()</signal> |
|||
<receiver>RecurringPending</receiver> |
|||
<slot>accept()</slot> |
|||
<hints> |
|||
<hint type="sourcelabel"> |
|||
<x>248</x> |
|||
<y>254</y> |
|||
</hint> |
|||
<hint type="destinationlabel"> |
|||
<x>157</x> |
|||
<y>274</y> |
|||
</hint> |
|||
</hints> |
|||
</connection> |
|||
<connection> |
|||
<sender>buttonBox</sender> |
|||
<signal>rejected()</signal> |
|||
<receiver>RecurringPending</receiver> |
|||
<slot>reject()</slot> |
|||
<hints> |
|||
<hint type="sourcelabel"> |
|||
<x>316</x> |
|||
<y>260</y> |
|||
</hint> |
|||
<hint type="destinationlabel"> |
|||
<x>286</x> |
|||
<y>274</y> |
|||
</hint> |
|||
</hints> |
|||
</connection> |
|||
</connections> |
|||
</ui> |
@ -0,0 +1,80 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<ui version="4.0"> |
|||
<class>RecurringPayments</class> |
|||
<widget class="QDialog" name="RecurringPayments"> |
|||
<property name="geometry"> |
|||
<rect> |
|||
<x>0</x> |
|||
<y>0</y> |
|||
<width>577</width> |
|||
<height>704</height> |
|||
</rect> |
|||
</property> |
|||
<property name="windowTitle"> |
|||
<string>Payments</string> |
|||
</property> |
|||
<layout class="QGridLayout" name="gridLayout"> |
|||
<item row="0" column="1"> |
|||
<widget class="QDialogButtonBox" name="buttonBox"> |
|||
<property name="orientation"> |
|||
<enum>Qt::Vertical</enum> |
|||
</property> |
|||
<property name="standardButtons"> |
|||
<set>QDialogButtonBox::Cancel|QDialogButtonBox::Ok</set> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="0" column="0"> |
|||
<widget class="QTableView" name="tableView"> |
|||
<property name="selectionMode"> |
|||
<enum>QAbstractItemView::SingleSelection</enum> |
|||
</property> |
|||
<property name="selectionBehavior"> |
|||
<enum>QAbstractItemView::SelectRows</enum> |
|||
</property> |
|||
<attribute name="horizontalHeaderStretchLastSection"> |
|||
<bool>true</bool> |
|||
</attribute> |
|||
<attribute name="verticalHeaderStretchLastSection"> |
|||
<bool>false</bool> |
|||
</attribute> |
|||
</widget> |
|||
</item> |
|||
</layout> |
|||
</widget> |
|||
<resources/> |
|||
<connections> |
|||
<connection> |
|||
<sender>buttonBox</sender> |
|||
<signal>accepted()</signal> |
|||
<receiver>RecurringPayments</receiver> |
|||
<slot>accept()</slot> |
|||
<hints> |
|||
<hint type="sourcelabel"> |
|||
<x>248</x> |
|||
<y>254</y> |
|||
</hint> |
|||
<hint type="destinationlabel"> |
|||
<x>157</x> |
|||
<y>274</y> |
|||
</hint> |
|||
</hints> |
|||
</connection> |
|||
<connection> |
|||
<sender>buttonBox</sender> |
|||
<signal>rejected()</signal> |
|||
<receiver>RecurringPayments</receiver> |
|||
<slot>reject()</slot> |
|||
<hints> |
|||
<hint type="sourcelabel"> |
|||
<x>316</x> |
|||
<y>260</y> |
|||
</hint> |
|||
<hint type="destinationlabel"> |
|||
<x>286</x> |
|||
<y>274</y> |
|||
</hint> |
|||
</hints> |
|||
</connection> |
|||
</connections> |
|||
</ui> |
Loading…
Reference in new issue