Browse Source
* hacked up labels * Custom widget for addresses with labels * turnstile to use labelsrecurring
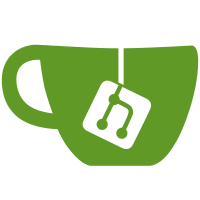
committed by
GitHub
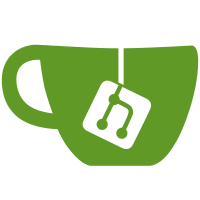
16 changed files with 144 additions and 48 deletions
@ -0,0 +1,41 @@ |
|||
#include "addresscombo.h" |
|||
|
|||
#include "addressbook.h" |
|||
#include "settings.h" |
|||
|
|||
AddressCombo::AddressCombo(QWidget* parent) : |
|||
QComboBox(parent) { |
|||
|
|||
} |
|||
|
|||
QString AddressCombo::itemText(int i) { |
|||
QString txt = QComboBox::itemText(i); |
|||
return AddressBook::addressFromAddressLabel(txt.split("(")[0].trimmed()); |
|||
} |
|||
|
|||
QString AddressCombo::currentText() { |
|||
QString txt = QComboBox::currentText(); |
|||
return AddressBook::addressFromAddressLabel(txt.split("(")[0].trimmed()); |
|||
} |
|||
|
|||
void AddressCombo::setCurrentText(const QString& text) { |
|||
for (int i=0; i < count(); i++) { |
|||
if (itemText(i) == text) { |
|||
QComboBox::setCurrentIndex(i); |
|||
} |
|||
} |
|||
} |
|||
|
|||
void AddressCombo::addItem(const QString& text, double bal) { |
|||
QString txt = AddressBook::addLabelToAddress(text); |
|||
if (bal > 0) |
|||
txt = txt % "(" % Settings::getZECDisplayFormat(bal) % ")"; |
|||
|
|||
QComboBox::addItem(txt); |
|||
} |
|||
|
|||
void AddressCombo::insertItem(int index, const QString& text, double bal) { |
|||
QString txt = AddressBook::addLabelToAddress(text) % |
|||
"(" % Settings::getZECDisplayFormat(bal) % ")"; |
|||
QComboBox::insertItem(index, txt); |
|||
} |
@ -0,0 +1,24 @@ |
|||
#ifndef ADDRESSCOMBO_H |
|||
#define ADDRESSCOMBO_H |
|||
|
|||
#include "precompiled.h" |
|||
|
|||
class AddressCombo : public QComboBox |
|||
{ |
|||
Q_OBJECT; |
|||
public: |
|||
explicit AddressCombo(QWidget* parent = nullptr); |
|||
|
|||
QString itemText(int i); |
|||
QString currentText(); |
|||
|
|||
void addItem(const QString& itemText, double bal); |
|||
void insertItem(int index, const QString& text, double bal = 0.0); |
|||
|
|||
public slots: |
|||
void setCurrentText(const QString& itemText); |
|||
|
|||
private: |
|||
}; |
|||
|
|||
#endif // ADDRESSCOMBO_H
|
Loading…
Reference in new issue