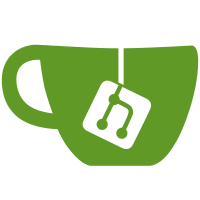
7 changed files with 331 additions and 6 deletions
@ -0,0 +1,139 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<ui version="4.0"> |
|||
<class>MigrationDialog</class> |
|||
<widget class="QDialog" name="MigrationDialog"> |
|||
<property name="geometry"> |
|||
<rect> |
|||
<x>0</x> |
|||
<y>0</y> |
|||
<width>511</width> |
|||
<height>498</height> |
|||
</rect> |
|||
</property> |
|||
<property name="windowTitle"> |
|||
<string>Migration Turnstile</string> |
|||
</property> |
|||
<layout class="QGridLayout" name="gridLayout"> |
|||
<item row="9" column="0" colspan="2"> |
|||
<widget class="QDialogButtonBox" name="buttonBox"> |
|||
<property name="orientation"> |
|||
<enum>Qt::Horizontal</enum> |
|||
</property> |
|||
<property name="standardButtons"> |
|||
<set>QDialogButtonBox::Cancel|QDialogButtonBox::Ok</set> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="7" column="0" colspan="2"> |
|||
<widget class="QGroupBox" name="groupBox"> |
|||
<property name="title"> |
|||
<string>Migration History</string> |
|||
</property> |
|||
<layout class="QGridLayout" name="gridLayout_2"> |
|||
<item row="2" column="1"> |
|||
<widget class="QLabel" name="label_4"> |
|||
<property name="text"> |
|||
<string>Migrated Amount</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="2" column="2"> |
|||
<widget class="QLabel" name="lblUnMigrated"> |
|||
<property name="text"> |
|||
<string notr="true">TextLabel</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="4" column="1" colspan="2"> |
|||
<widget class="QTableView" name="tblTxids"> |
|||
<attribute name="horizontalHeaderStretchLastSection"> |
|||
<bool>true</bool> |
|||
</attribute> |
|||
</widget> |
|||
</item> |
|||
<item row="0" column="2"> |
|||
<widget class="QLabel" name="lblMigrated"> |
|||
<property name="text"> |
|||
<string notr="true">TextLabel</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="0" column="1"> |
|||
<widget class="QLabel" name="label_2"> |
|||
<property name="text"> |
|||
<string>Unmigrated Amount</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
</layout> |
|||
</widget> |
|||
</item> |
|||
<item row="0" column="0" colspan="2"> |
|||
<widget class="QCheckBox" name="chkEnabled"> |
|||
<property name="text"> |
|||
<string>Sprout -> Sapling migration enabled</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="1" column="0" colspan="2"> |
|||
<widget class="QLabel" name="label"> |
|||
<property name="text"> |
|||
<string>If enabled, zcashd will slowly migrate your Sprout shielded funds to your Sapling address. </string> |
|||
</property> |
|||
<property name="wordWrap"> |
|||
<bool>true</bool> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="4" column="0" colspan="2"> |
|||
<widget class="Line" name="line"> |
|||
<property name="orientation"> |
|||
<enum>Qt::Horizontal</enum> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="2" column="0" colspan="2"> |
|||
<widget class="QLabel" name="lblSaplingAddress"> |
|||
<property name="text"> |
|||
<string notr="true"/> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
</layout> |
|||
</widget> |
|||
<resources/> |
|||
<connections> |
|||
<connection> |
|||
<sender>buttonBox</sender> |
|||
<signal>accepted()</signal> |
|||
<receiver>MigrationDialog</receiver> |
|||
<slot>accept()</slot> |
|||
<hints> |
|||
<hint type="sourcelabel"> |
|||
<x>248</x> |
|||
<y>254</y> |
|||
</hint> |
|||
<hint type="destinationlabel"> |
|||
<x>157</x> |
|||
<y>274</y> |
|||
</hint> |
|||
</hints> |
|||
</connection> |
|||
<connection> |
|||
<sender>buttonBox</sender> |
|||
<signal>rejected()</signal> |
|||
<receiver>MigrationDialog</receiver> |
|||
<slot>reject()</slot> |
|||
<hints> |
|||
<hint type="sourcelabel"> |
|||
<x>316</x> |
|||
<y>260</y> |
|||
</hint> |
|||
<hint type="destinationlabel"> |
|||
<x>286</x> |
|||
<y>274</y> |
|||
</hint> |
|||
</hints> |
|||
</connection> |
|||
</connections> |
|||
</ui> |
Loading…
Reference in new issue