Browse Source
Eventually (after 0.10) these files will hold the logic for crypto verification routines, and CKey/CPubKey will call into them.pull/4/head
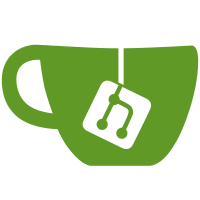
5 changed files with 88 additions and 52 deletions
@ -0,0 +1,63 @@ |
|||
#include "eccryptoverify.h" |
|||
|
|||
namespace { |
|||
|
|||
int CompareBigEndian(const unsigned char *c1, size_t c1len, const unsigned char *c2, size_t c2len) { |
|||
while (c1len > c2len) { |
|||
if (*c1) |
|||
return 1; |
|||
c1++; |
|||
c1len--; |
|||
} |
|||
while (c2len > c1len) { |
|||
if (*c2) |
|||
return -1; |
|||
c2++; |
|||
c2len--; |
|||
} |
|||
while (c1len > 0) { |
|||
if (*c1 > *c2) |
|||
return 1; |
|||
if (*c2 > *c1) |
|||
return -1; |
|||
c1++; |
|||
c2++; |
|||
c1len--; |
|||
} |
|||
return 0; |
|||
} |
|||
|
|||
/** Order of secp256k1's generator minus 1. */ |
|||
const unsigned char vchMaxModOrder[32] = { |
|||
0xFF,0xFF,0xFF,0xFF,0xFF,0xFF,0xFF,0xFF, |
|||
0xFF,0xFF,0xFF,0xFF,0xFF,0xFF,0xFF,0xFE, |
|||
0xBA,0xAE,0xDC,0xE6,0xAF,0x48,0xA0,0x3B, |
|||
0xBF,0xD2,0x5E,0x8C,0xD0,0x36,0x41,0x40 |
|||
}; |
|||
|
|||
/** Half of the order of secp256k1's generator minus 1. */ |
|||
const unsigned char vchMaxModHalfOrder[32] = { |
|||
0x7F,0xFF,0xFF,0xFF,0xFF,0xFF,0xFF,0xFF, |
|||
0xFF,0xFF,0xFF,0xFF,0xFF,0xFF,0xFF,0xFF, |
|||
0x5D,0x57,0x6E,0x73,0x57,0xA4,0x50,0x1D, |
|||
0xDF,0xE9,0x2F,0x46,0x68,0x1B,0x20,0xA0 |
|||
}; |
|||
|
|||
const unsigned char vchZero[1] = {0}; |
|||
} // anon namespace
|
|||
|
|||
namespace eccrypto { |
|||
|
|||
bool Check(const unsigned char *vch) { |
|||
return vch && |
|||
CompareBigEndian(vch, 32, vchZero, 0) > 0 && |
|||
CompareBigEndian(vch, 32, vchMaxModOrder, 32) <= 0; |
|||
} |
|||
|
|||
bool CheckSignatureElement(const unsigned char *vch, int len, bool half) { |
|||
return vch && |
|||
CompareBigEndian(vch, len, vchZero, 0) > 0 && |
|||
CompareBigEndian(vch, len, half ? vchMaxModHalfOrder : vchMaxModOrder, 32) <= 0; |
|||
} |
|||
|
|||
} // namespace eccrypto
|
@ -0,0 +1,19 @@ |
|||
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|||
// Copyright (c) 2009-2013 The Bitcoin developers
|
|||
// Distributed under the MIT/X11 software license, see the accompanying
|
|||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|||
|
|||
#ifndef BITCOIN_EC_CRYPTO_VERIFY_H |
|||
#define BITCOIN_EC_CRYPTO_VERIFY_H |
|||
|
|||
#include <vector> |
|||
#include <cstdlib> |
|||
class uint256; |
|||
|
|||
namespace eccrypto { |
|||
|
|||
bool Check(const unsigned char *vch); |
|||
bool CheckSignatureElement(const unsigned char *vch, int len, bool half); |
|||
|
|||
} // eccrypto namespace
|
|||
#endif |
Loading…
Reference in new issue