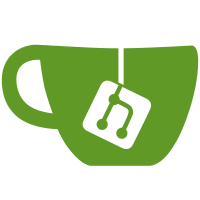
committed by
GitHub
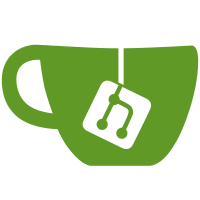
18 changed files with 1964 additions and 18 deletions
@ -0,0 +1,31 @@ |
|||
#!/usr/bin/env python2 |
|||
# Copyright (c) 2018 The Hush developers |
|||
# Distributed under the MIT software license, see the accompanying |
|||
# file COPYING or http://www.opensource.org/licenses/mit-license.php. |
|||
|
|||
from test_framework.test_framework import BitcoinTestFramework |
|||
from test_framework.util import assert_equal, initialize_chain_clean, \ |
|||
start_node, stop_node, wait_bitcoinds |
|||
|
|||
|
|||
class DPoWTest(BitcoinTestFramework): |
|||
|
|||
def setup_chain(self): |
|||
print("Initializing test directory "+self.options.tmpdir) |
|||
initialize_chain_clean(self.options.tmpdir, 1) |
|||
|
|||
def setup_network(self): |
|||
self.nodes = [] |
|||
self.is_network_split = False |
|||
self.nodes.append(start_node(0, self.options.tmpdir)) |
|||
|
|||
def run_test(self): |
|||
self.nodes[0].generate(3) |
|||
rpc = self.nodes[0] |
|||
|
|||
# Verify that basic RPC functions exist and work |
|||
result = rpc.calc_MoM(2,20) |
|||
print result |
|||
|
|||
if __name__ == '__main__': |
|||
DPoWTest().main() |
@ -0,0 +1,227 @@ |
|||
// Copyright (c) 2014-2016 The Bitcoin Core developers
|
|||
// Distributed under the MIT software license, see the accompanying
|
|||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|||
|
|||
#include <key_io.h> |
|||
|
|||
#include <base58.h> |
|||
#include <bech32.h> |
|||
#include <script/script.h> |
|||
#include <utilstrencodings.h> |
|||
|
|||
#include <boost/variant/apply_visitor.hpp> |
|||
#include <boost/variant/static_visitor.hpp> |
|||
|
|||
#include <assert.h> |
|||
#include <string.h> |
|||
#include <algorithm> |
|||
|
|||
namespace |
|||
{ |
|||
class DestinationEncoder : public boost::static_visitor<std::string> |
|||
{ |
|||
private: |
|||
const CChainParams& m_params; |
|||
|
|||
public: |
|||
DestinationEncoder(const CChainParams& params) : m_params(params) {} |
|||
|
|||
std::string operator()(const CKeyID& id) const |
|||
{ |
|||
std::vector<unsigned char> data = m_params.Base58Prefix(CChainParams::PUBKEY_ADDRESS); |
|||
data.insert(data.end(), id.begin(), id.end()); |
|||
return EncodeBase58Check(data); |
|||
} |
|||
|
|||
std::string operator()(const CScriptID& id) const |
|||
{ |
|||
std::vector<unsigned char> data = m_params.Base58Prefix(CChainParams::SCRIPT_ADDRESS); |
|||
data.insert(data.end(), id.begin(), id.end()); |
|||
return EncodeBase58Check(data); |
|||
} |
|||
|
|||
std::string operator()(const WitnessV0KeyHash& id) const |
|||
{ |
|||
std::vector<unsigned char> data = {0}; |
|||
data.reserve(33); |
|||
ConvertBits<8, 5, true>([&](unsigned char c) { data.push_back(c); }, id.begin(), id.end()); |
|||
return bech32::Encode(m_params.Bech32HRP(), data); |
|||
} |
|||
|
|||
std::string operator()(const WitnessV0ScriptHash& id) const |
|||
{ |
|||
std::vector<unsigned char> data = {0}; |
|||
data.reserve(53); |
|||
ConvertBits<8, 5, true>([&](unsigned char c) { data.push_back(c); }, id.begin(), id.end()); |
|||
return bech32::Encode(m_params.Bech32HRP(), data); |
|||
} |
|||
|
|||
std::string operator()(const WitnessUnknown& id) const |
|||
{ |
|||
if (id.version < 1 || id.version > 16 || id.length < 2 || id.length > 40) { |
|||
return {}; |
|||
} |
|||
std::vector<unsigned char> data = {(unsigned char)id.version}; |
|||
data.reserve(1 + (id.length * 8 + 4) / 5); |
|||
ConvertBits<8, 5, true>([&](unsigned char c) { data.push_back(c); }, id.program, id.program + id.length); |
|||
return bech32::Encode(m_params.Bech32HRP(), data); |
|||
} |
|||
|
|||
std::string operator()(const CNoDestination& no) const { return {}; } |
|||
}; |
|||
|
|||
CTxDestination DecodeDestination(const std::string& str, const CChainParams& params) |
|||
{ |
|||
std::vector<unsigned char> data; |
|||
uint160 hash; |
|||
if (DecodeBase58Check(str, data)) { |
|||
// base58-encoded Bitcoin addresses.
|
|||
// Public-key-hash-addresses have version 0 (or 111 testnet).
|
|||
// The data vector contains RIPEMD160(SHA256(pubkey)), where pubkey is the serialized public key.
|
|||
const std::vector<unsigned char>& pubkey_prefix = params.Base58Prefix(CChainParams::PUBKEY_ADDRESS); |
|||
if (data.size() == hash.size() + pubkey_prefix.size() && std::equal(pubkey_prefix.begin(), pubkey_prefix.end(), data.begin())) { |
|||
std::copy(data.begin() + pubkey_prefix.size(), data.end(), hash.begin()); |
|||
return CKeyID(hash); |
|||
} |
|||
// Script-hash-addresses have version 5 (or 196 testnet).
|
|||
// The data vector contains RIPEMD160(SHA256(cscript)), where cscript is the serialized redemption script.
|
|||
const std::vector<unsigned char>& script_prefix = params.Base58Prefix(CChainParams::SCRIPT_ADDRESS); |
|||
if (data.size() == hash.size() + script_prefix.size() && std::equal(script_prefix.begin(), script_prefix.end(), data.begin())) { |
|||
std::copy(data.begin() + script_prefix.size(), data.end(), hash.begin()); |
|||
return CScriptID(hash); |
|||
} |
|||
} |
|||
data.clear(); |
|||
auto bech = bech32::Decode(str); |
|||
if (bech.second.size() > 0 && bech.first == params.Bech32HRP()) { |
|||
// Bech32 decoding
|
|||
int version = bech.second[0]; // The first 5 bit symbol is the witness version (0-16)
|
|||
// The rest of the symbols are converted witness program bytes.
|
|||
data.reserve(((bech.second.size() - 1) * 5) / 8); |
|||
if (ConvertBits<5, 8, false>([&](unsigned char c) { data.push_back(c); }, bech.second.begin() + 1, bech.second.end())) { |
|||
if (version == 0) { |
|||
{ |
|||
WitnessV0KeyHash keyid; |
|||
if (data.size() == keyid.size()) { |
|||
std::copy(data.begin(), data.end(), keyid.begin()); |
|||
return keyid; |
|||
} |
|||
} |
|||
{ |
|||
WitnessV0ScriptHash scriptid; |
|||
if (data.size() == scriptid.size()) { |
|||
std::copy(data.begin(), data.end(), scriptid.begin()); |
|||
return scriptid; |
|||
} |
|||
} |
|||
return CNoDestination(); |
|||
} |
|||
if (version > 16 || data.size() < 2 || data.size() > 40) { |
|||
return CNoDestination(); |
|||
} |
|||
WitnessUnknown unk; |
|||
unk.version = version; |
|||
std::copy(data.begin(), data.end(), unk.program); |
|||
unk.length = data.size(); |
|||
return unk; |
|||
} |
|||
} |
|||
return CNoDestination(); |
|||
} |
|||
} // namespace
|
|||
|
|||
CKey DecodeSecret(const std::string& str) |
|||
{ |
|||
CKey key; |
|||
std::vector<unsigned char> data; |
|||
if (DecodeBase58Check(str, data)) { |
|||
const std::vector<unsigned char>& privkey_prefix = Params().Base58Prefix(CChainParams::SECRET_KEY); |
|||
if ((data.size() == 32 + privkey_prefix.size() || (data.size() == 33 + privkey_prefix.size() && data.back() == 1)) && |
|||
std::equal(privkey_prefix.begin(), privkey_prefix.end(), data.begin())) { |
|||
bool compressed = data.size() == 33 + privkey_prefix.size(); |
|||
key.Set(data.begin() + privkey_prefix.size(), data.begin() + privkey_prefix.size() + 32, compressed); |
|||
} |
|||
} |
|||
memory_cleanse(data.data(), data.size()); |
|||
return key; |
|||
} |
|||
|
|||
std::string EncodeSecret(const CKey& key) |
|||
{ |
|||
assert(key.IsValid()); |
|||
std::vector<unsigned char> data = Params().Base58Prefix(CChainParams::SECRET_KEY); |
|||
data.insert(data.end(), key.begin(), key.end()); |
|||
if (key.IsCompressed()) { |
|||
data.push_back(1); |
|||
} |
|||
std::string ret = EncodeBase58Check(data); |
|||
memory_cleanse(data.data(), data.size()); |
|||
return ret; |
|||
} |
|||
|
|||
CExtPubKey DecodeExtPubKey(const std::string& str) |
|||
{ |
|||
CExtPubKey key; |
|||
std::vector<unsigned char> data; |
|||
if (DecodeBase58Check(str, data)) { |
|||
const std::vector<unsigned char>& prefix = Params().Base58Prefix(CChainParams::EXT_PUBLIC_KEY); |
|||
if (data.size() == BIP32_EXTKEY_SIZE + prefix.size() && std::equal(prefix.begin(), prefix.end(), data.begin())) { |
|||
key.Decode(data.data() + prefix.size()); |
|||
} |
|||
} |
|||
return key; |
|||
} |
|||
|
|||
std::string EncodeExtPubKey(const CExtPubKey& key) |
|||
{ |
|||
std::vector<unsigned char> data = Params().Base58Prefix(CChainParams::EXT_PUBLIC_KEY); |
|||
size_t size = data.size(); |
|||
data.resize(size + BIP32_EXTKEY_SIZE); |
|||
key.Encode(data.data() + size); |
|||
std::string ret = EncodeBase58Check(data); |
|||
return ret; |
|||
} |
|||
|
|||
CExtKey DecodeExtKey(const std::string& str) |
|||
{ |
|||
CExtKey key; |
|||
std::vector<unsigned char> data; |
|||
if (DecodeBase58Check(str, data)) { |
|||
const std::vector<unsigned char>& prefix = Params().Base58Prefix(CChainParams::EXT_SECRET_KEY); |
|||
if (data.size() == BIP32_EXTKEY_SIZE + prefix.size() && std::equal(prefix.begin(), prefix.end(), data.begin())) { |
|||
key.Decode(data.data() + prefix.size()); |
|||
} |
|||
} |
|||
return key; |
|||
} |
|||
|
|||
std::string EncodeExtKey(const CExtKey& key) |
|||
{ |
|||
std::vector<unsigned char> data = Params().Base58Prefix(CChainParams::EXT_SECRET_KEY); |
|||
size_t size = data.size(); |
|||
data.resize(size + BIP32_EXTKEY_SIZE); |
|||
key.Encode(data.data() + size); |
|||
std::string ret = EncodeBase58Check(data); |
|||
memory_cleanse(data.data(), data.size()); |
|||
return ret; |
|||
} |
|||
|
|||
std::string EncodeDestination(const CTxDestination& dest) |
|||
{ |
|||
return boost::apply_visitor(DestinationEncoder(Params()), dest); |
|||
} |
|||
|
|||
CTxDestination DecodeDestination(const std::string& str) |
|||
{ |
|||
return DecodeDestination(str, Params()); |
|||
} |
|||
|
|||
bool IsValidDestinationString(const std::string& str, const CChainParams& params) |
|||
{ |
|||
return IsValidDestination(DecodeDestination(str, params)); |
|||
} |
|||
|
|||
bool IsValidDestinationString(const std::string& str) |
|||
{ |
|||
return IsValidDestinationString(str, Params()); |
|||
} |
@ -0,0 +1,29 @@ |
|||
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|||
// Copyright (c) 2009-2015 The Bitcoin Core developers
|
|||
// Distributed under the MIT software license, see the accompanying
|
|||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|||
|
|||
#ifndef BITCOIN_KEYIO_H |
|||
#define BITCOIN_KEYIO_H |
|||
|
|||
#include <chainparams.h> |
|||
#include <key.h> |
|||
#include <pubkey.h> |
|||
#include <script/standard.h> |
|||
|
|||
#include <string> |
|||
|
|||
CKey DecodeSecret(const std::string& str); |
|||
std::string EncodeSecret(const CKey& key); |
|||
|
|||
CExtKey DecodeExtKey(const std::string& str); |
|||
std::string EncodeExtKey(const CExtKey& extkey); |
|||
CExtPubKey DecodeExtPubKey(const std::string& str); |
|||
std::string EncodeExtPubKey(const CExtPubKey& extpubkey); |
|||
|
|||
std::string EncodeDestination(const CTxDestination& dest); |
|||
CTxDestination DecodeDestination(const std::string& str); |
|||
bool IsValidDestinationString(const std::string& str); |
|||
bool IsValidDestinationString(const std::string& str, const CChainParams& params); |
|||
|
|||
#endif // BITCOIN_KEYIO_H
|
@ -0,0 +1,103 @@ |
|||
/******************************************************************************
|
|||
* Copyright © 2014-2018 The SuperNET Developers. * |
|||
* * |
|||
* See the AUTHORS, DEVELOPER-AGREEMENT and LICENSE files at * |
|||
* the top-level directory of this distribution for the individual copyright * |
|||
* holder information and the developer policies on copyright and licensing. * |
|||
* * |
|||
* Unless otherwise agreed in a custom licensing agreement, no part of the * |
|||
* SuperNET software, including this file may be copied, modified, propagated * |
|||
* or distributed except according to the terms contained in the LICENSE file * |
|||
* * |
|||
* Removal or modification of this copyright notice is prohibited. * |
|||
* * |
|||
******************************************************************************/ |
|||
|
|||
#ifndef komodo_rpcblockchain_h |
|||
#define komodo_rpcblockchain_h |
|||
|
|||
#include "main.h" |
|||
|
|||
int32_t komodo_MoMdata(int32_t *notarized_htp,uint256 *MoMp,uint256 *kmdtxidp,int32_t height,uint256 *MoMoMp,int32_t *MoMoMoffsetp,int32_t *MoMoMdepthp,int32_t *kmdstartip,int32_t *kmdendip); |
|||
uint256 komodo_calcMoM(int32_t height,int32_t MoMdepth); |
|||
extern char ASSETCHAINS_SYMBOL[65]; |
|||
|
|||
int32_t komodo_MoM(int32_t *notarized_heightp,uint256 *MoMp,uint256 *kmdtxidp,int32_t nHeight,uint256 *MoMoMp,int32_t *MoMoMoffsetp,int32_t *MoMoMdepthp,int32_t *kmdstartip,int32_t *kmdendip) |
|||
{ |
|||
int32_t depth,notarized_ht; uint256 MoM,kmdtxid; |
|||
depth = komodo_MoMdata(¬arized_ht,&MoM,&kmdtxid,nHeight,MoMoMp,MoMoMoffsetp,MoMoMdepthp,kmdstartip,kmdendip); |
|||
memset(MoMp,0,sizeof(*MoMp)); |
|||
memset(kmdtxidp,0,sizeof(*kmdtxidp)); |
|||
*notarized_heightp = 0; |
|||
if ( depth > 0 && notarized_ht > 0 && nHeight > notarized_ht-depth && nHeight <= notarized_ht ) |
|||
{ |
|||
*MoMp = MoM; |
|||
*notarized_heightp = notarized_ht; |
|||
*kmdtxidp = kmdtxid; |
|||
} |
|||
return(depth); |
|||
} |
|||
|
|||
UniValue calc_MoM(const UniValue& params, bool fHelp) |
|||
{ |
|||
int32_t height,MoMdepth; uint256 MoM; UniValue ret(UniValue::VOBJ); UniValue a(UniValue::VARR); |
|||
if ( fHelp || params.size() != 2 ) |
|||
throw runtime_error("calc_MoM height MoMdepth\n"); |
|||
LOCK(cs_main); |
|||
height = atoi(params[0].get_str().c_str()); |
|||
MoMdepth = atoi(params[1].get_str().c_str()); |
|||
if ( height <= 0 ) |
|||
throw runtime_error("calc_MoM illegal height, must be positive\n"); |
|||
if ( MoMdepth <= 0 || MoMdepth >= height ) |
|||
throw runtime_error("calc_MoM illegal MoMdepth, must be positive and less than height\n"); |
|||
|
|||
//fprintf(stderr,"height_MoM height.%d\n",height);
|
|||
MoM = komodo_calcMoM(height,MoMdepth); |
|||
ret.push_back(Pair("coin",(char *)(ASSETCHAINS_SYMBOL[0] == 0 ? "KMD" : ASSETCHAINS_SYMBOL))); |
|||
ret.push_back(Pair("height",height)); |
|||
ret.push_back(Pair("MoMdepth",MoMdepth)); |
|||
ret.push_back(Pair("MoM",MoM.GetHex())); |
|||
return ret; |
|||
} |
|||
|
|||
UniValue height_MoM(const UniValue& params, bool fHelp) |
|||
{ |
|||
int32_t height,depth,notarized_height,MoMoMdepth,MoMoMoffset,kmdstarti,kmdendi; uint256 MoM,MoMoM,kmdtxid; uint32_t timestamp = 0; UniValue ret(UniValue::VOBJ); UniValue a(UniValue::VARR); |
|||
if ( fHelp || params.size() != 1 ) |
|||
throw runtime_error("height_MoM height\n"); |
|||
LOCK(cs_main); |
|||
height = atoi(params[0].get_str().c_str()); |
|||
if ( height <= 0 ) |
|||
{ |
|||
if ( chainActive.Tip() == 0 ) |
|||
{ |
|||
ret.push_back(Pair("error",(char *)"no active chain yet")); |
|||
return(ret); |
|||
} |
|||
height = chainActive.Tip()->nHeight; |
|||
} |
|||
//fprintf(stderr,"height_MoM height.%d\n",height);
|
|||
depth = komodo_MoM(¬arized_height,&MoM,&kmdtxid,height,&MoMoM,&MoMoMoffset,&MoMoMdepth,&kmdstarti,&kmdendi); |
|||
ret.push_back(Pair("coin",(char *)(ASSETCHAINS_SYMBOL[0] == 0 ? "KMD" : ASSETCHAINS_SYMBOL))); |
|||
ret.push_back(Pair("height",height)); |
|||
ret.push_back(Pair("timestamp",(uint64_t)timestamp)); |
|||
if ( depth > 0 ) |
|||
{ |
|||
ret.push_back(Pair("depth",depth)); |
|||
ret.push_back(Pair("notarized_height",notarized_height)); |
|||
ret.push_back(Pair("MoM",MoM.GetHex())); |
|||
ret.push_back(Pair("kmdtxid",kmdtxid.GetHex())); |
|||
if ( ASSETCHAINS_SYMBOL[0] != 0 ) |
|||
{ |
|||
ret.push_back(Pair("MoMoM",MoMoM.GetHex())); |
|||
ret.push_back(Pair("MoMoMoffset",MoMoMoffset)); |
|||
ret.push_back(Pair("MoMoMdepth",MoMoMdepth)); |
|||
ret.push_back(Pair("kmdstarti",kmdstarti)); |
|||
ret.push_back(Pair("kmdendi",kmdendi)); |
|||
} |
|||
} else ret.push_back(Pair("error",(char *)"no MoM for height")); |
|||
|
|||
return ret; |
|||
} |
|||
|
|||
#endif /* komodo_rpcblockchain_h */ |
File diff suppressed because it is too large
Loading…
Reference in new issue