Browse Source
-checklevel gets a new meaning: 0: verify blocks can be read from disk (like before) 1: verify (contextless) block validity (like before) 2: verify undo files can be read and have good checksums 3: verify coin database is consistent with the last few blocks (close to level 6 before) 4: verify all validity rules of the last few blocks Level 3 is the new default, as it's reasonably fast. As level 3 and 4 are implemented using an in-memory rollback of the database, they are limited to as many blocks as possible without exceeding the limits set by -dbcache. The default of -dbcache=25 allows for some 150-200 blocks to be rolled back. In case an error is found, the application quits with a message instructing the user to restart with -reindex. Better instructions, and automatic recovery (when possible) or automatic reindexing are left as future work.pull/145/head
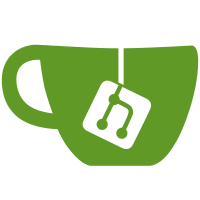
3 changed files with 60 additions and 14 deletions
Loading…
Reference in new issue