Browse Source
Create a monitoring task that counts how many blocks have been found in the last four hours. If very few or too many have been found, an alert is triggered. "Very few" and "too many" are set based on a false positive rate of once every fifty years of constant running with constant hashing power, which works out to getting 5 or fewer or 48 or more blocks in four hours (instead of the average of 24). Only one alert per day is triggered, so if you get disconnected from the network (or are being Sybil'ed) -alertnotify will be triggered after 3.5 hours but you won't get another -alertnotify for 24 hours. Tested with a new unit test and by running on the main network with -debug=partitioncheck Run test/test_bitcoin --log_level=message to see the alert messages: WARNING: check your network connection, 3 blocks received in the last 4 hours (24 expected) WARNING: abnormally high number of blocks generated, 60 blocks received in the last 4 hours (24 expected) The -debug=partitioncheck debug.log messages look like: ThreadPartitionCheck : Found 22 blocks in the last 4 hours ThreadPartitionCheck : likelihood: 0.0777702pull/145/head
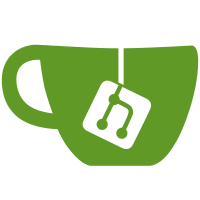
4 changed files with 133 additions and 0 deletions
Loading…
Reference in new issue