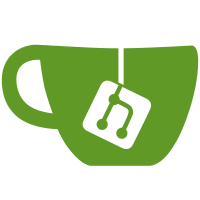
14 changed files with 382 additions and 681 deletions
@ -1,137 +1,137 @@ |
|||
// Copyright John W. Wilkinson 2007 - 2013
|
|||
// Copyright John W. Wilkinson 2007 - 2009.
|
|||
// Distributed under the MIT License, see accompanying file LICENSE.txt
|
|||
|
|||
// json spirit version 4.06
|
|||
// json spirit version 4.03
|
|||
|
|||
#include "json_spirit_reader.h" |
|||
#include "json_spirit_reader_template.h" |
|||
|
|||
using namespace json_spirit; |
|||
|
|||
#ifdef JSON_SPIRIT_VALUE_ENABLED |
|||
bool json_spirit::read( const std::string& s, Value& value ) |
|||
{ |
|||
return read_string( s, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( const std::string& s, Value& value ) |
|||
{ |
|||
read_string_or_throw( s, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::istream& is, Value& value ) |
|||
{ |
|||
return read_stream( is, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::istream& is, Value& value ) |
|||
{ |
|||
read_stream_or_throw( is, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::string::const_iterator& begin, std::string::const_iterator end, Value& value ) |
|||
{ |
|||
return read_range( begin, end, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::string::const_iterator& begin, std::string::const_iterator end, Value& value ) |
|||
{ |
|||
begin = read_range_or_throw( begin, end, value ); |
|||
} |
|||
#endif |
|||
bool json_spirit::read( const std::string& s, Value& value ) |
|||
{ |
|||
return read_string( s, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( const std::string& s, Value& value ) |
|||
{ |
|||
read_string_or_throw( s, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::istream& is, Value& value ) |
|||
{ |
|||
return read_stream( is, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::istream& is, Value& value ) |
|||
{ |
|||
read_stream_or_throw( is, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::string::const_iterator& begin, std::string::const_iterator end, Value& value ) |
|||
{ |
|||
return read_range( begin, end, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::string::const_iterator& begin, std::string::const_iterator end, Value& value ) |
|||
{ |
|||
begin = read_range_or_throw( begin, end, value ); |
|||
} |
|||
|
|||
#ifndef BOOST_NO_STD_WSTRING |
|||
|
|||
bool json_spirit::read( const std::wstring& s, wValue& value ) |
|||
{ |
|||
return read_string( s, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( const std::wstring& s, wValue& value ) |
|||
{ |
|||
read_string_or_throw( s, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::wistream& is, wValue& value ) |
|||
{ |
|||
return read_stream( is, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::wistream& is, wValue& value ) |
|||
{ |
|||
read_stream_or_throw( is, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::wstring::const_iterator& begin, std::wstring::const_iterator end, wValue& value ) |
|||
{ |
|||
return read_range( begin, end, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::wstring::const_iterator& begin, std::wstring::const_iterator end, wValue& value ) |
|||
{ |
|||
begin = read_range_or_throw( begin, end, value ); |
|||
} |
|||
|
|||
#if defined( JSON_SPIRIT_WVALUE_ENABLED ) && !defined( BOOST_NO_STD_WSTRING ) |
|||
bool json_spirit::read( const std::wstring& s, wValue& value ) |
|||
{ |
|||
return read_string( s, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( const std::wstring& s, wValue& value ) |
|||
{ |
|||
read_string_or_throw( s, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::wistream& is, wValue& value ) |
|||
{ |
|||
return read_stream( is, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::wistream& is, wValue& value ) |
|||
{ |
|||
read_stream_or_throw( is, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::wstring::const_iterator& begin, std::wstring::const_iterator end, wValue& value ) |
|||
{ |
|||
return read_range( begin, end, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::wstring::const_iterator& begin, std::wstring::const_iterator end, wValue& value ) |
|||
{ |
|||
begin = read_range_or_throw( begin, end, value ); |
|||
} |
|||
#endif |
|||
|
|||
#ifdef JSON_SPIRIT_MVALUE_ENABLED |
|||
bool json_spirit::read( const std::string& s, mValue& value ) |
|||
{ |
|||
return read_string( s, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( const std::string& s, mValue& value ) |
|||
{ |
|||
read_string_or_throw( s, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::istream& is, mValue& value ) |
|||
{ |
|||
return read_stream( is, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::istream& is, mValue& value ) |
|||
{ |
|||
read_stream_or_throw( is, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::string::const_iterator& begin, std::string::const_iterator end, mValue& value ) |
|||
{ |
|||
return read_range( begin, end, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::string::const_iterator& begin, std::string::const_iterator end, mValue& value ) |
|||
{ |
|||
begin = read_range_or_throw( begin, end, value ); |
|||
} |
|||
#endif |
|||
bool json_spirit::read( const std::string& s, mValue& value ) |
|||
{ |
|||
return read_string( s, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( const std::string& s, mValue& value ) |
|||
{ |
|||
read_string_or_throw( s, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::istream& is, mValue& value ) |
|||
{ |
|||
return read_stream( is, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::istream& is, mValue& value ) |
|||
{ |
|||
read_stream_or_throw( is, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::string::const_iterator& begin, std::string::const_iterator end, mValue& value ) |
|||
{ |
|||
return read_range( begin, end, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::string::const_iterator& begin, std::string::const_iterator end, mValue& value ) |
|||
{ |
|||
begin = read_range_or_throw( begin, end, value ); |
|||
} |
|||
|
|||
#ifndef BOOST_NO_STD_WSTRING |
|||
|
|||
bool json_spirit::read( const std::wstring& s, wmValue& value ) |
|||
{ |
|||
return read_string( s, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( const std::wstring& s, wmValue& value ) |
|||
{ |
|||
read_string_or_throw( s, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::wistream& is, wmValue& value ) |
|||
{ |
|||
return read_stream( is, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::wistream& is, wmValue& value ) |
|||
{ |
|||
read_stream_or_throw( is, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::wstring::const_iterator& begin, std::wstring::const_iterator end, wmValue& value ) |
|||
{ |
|||
return read_range( begin, end, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::wstring::const_iterator& begin, std::wstring::const_iterator end, wmValue& value ) |
|||
{ |
|||
begin = read_range_or_throw( begin, end, value ); |
|||
} |
|||
|
|||
#if defined( JSON_SPIRIT_WMVALUE_ENABLED ) && !defined( BOOST_NO_STD_WSTRING ) |
|||
bool json_spirit::read( const std::wstring& s, wmValue& value ) |
|||
{ |
|||
return read_string( s, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( const std::wstring& s, wmValue& value ) |
|||
{ |
|||
read_string_or_throw( s, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::wistream& is, wmValue& value ) |
|||
{ |
|||
return read_stream( is, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::wistream& is, wmValue& value ) |
|||
{ |
|||
read_stream_or_throw( is, value ); |
|||
} |
|||
|
|||
bool json_spirit::read( std::wstring::const_iterator& begin, std::wstring::const_iterator end, wmValue& value ) |
|||
{ |
|||
return read_range( begin, end, value ); |
|||
} |
|||
|
|||
void json_spirit::read_or_throw( std::wstring::const_iterator& begin, std::wstring::const_iterator end, wmValue& value ) |
|||
{ |
|||
begin = read_range_or_throw( begin, end, value ); |
|||
} |
|||
#endif |
|||
|
@ -1,96 +1,95 @@ |
|||
// Copyright John W. Wilkinson 2007 - 2013
|
|||
// Copyright John W. Wilkinson 2007 - 2009.
|
|||
// Distributed under the MIT License, see accompanying file LICENSE.txt
|
|||
|
|||
// json spirit version 4.06
|
|||
// json spirit version 4.03
|
|||
|
|||
#include "json_spirit_writer.h" |
|||
#include "json_spirit_writer_template.h" |
|||
|
|||
using namespace json_spirit; |
|||
|
|||
#ifdef JSON_SPIRIT_VALUE_ENABLED |
|||
void json_spirit::write( const Value& value, std::ostream& os, unsigned int options ) |
|||
{ |
|||
write_stream( value, os, options ); |
|||
} |
|||
std::string json_spirit::write( const Value& value, unsigned int options ) |
|||
{ |
|||
return write_string( value, options ); |
|||
} |
|||
|
|||
void json_spirit::write_formatted( const Value& value, std::ostream& os ) |
|||
{ |
|||
write_stream( value, os, pretty_print ); |
|||
} |
|||
|
|||
std::string json_spirit::write_formatted( const Value& value ) |
|||
{ |
|||
return write_string( value, pretty_print ); |
|||
} |
|||
#endif |
|||
void json_spirit::write( const Value& value, std::ostream& os ) |
|||
{ |
|||
write_stream( value, os, false ); |
|||
} |
|||
|
|||
#ifdef JSON_SPIRIT_MVALUE_ENABLED |
|||
void json_spirit::write( const mValue& value, std::ostream& os, unsigned int options ) |
|||
{ |
|||
write_stream( value, os, options ); |
|||
} |
|||
|
|||
std::string json_spirit::write( const mValue& value, unsigned int options ) |
|||
{ |
|||
return write_string( value, options ); |
|||
} |
|||
|
|||
void json_spirit::write_formatted( const mValue& value, std::ostream& os ) |
|||
{ |
|||
write_stream( value, os, pretty_print ); |
|||
} |
|||
|
|||
std::string json_spirit::write_formatted( const mValue& value ) |
|||
{ |
|||
return write_string( value, pretty_print ); |
|||
} |
|||
#endif |
|||
void json_spirit::write_formatted( const Value& value, std::ostream& os ) |
|||
{ |
|||
write_stream( value, os, true ); |
|||
} |
|||
|
|||
std::string json_spirit::write( const Value& value ) |
|||
{ |
|||
return write_string( value, false ); |
|||
} |
|||
|
|||
std::string json_spirit::write_formatted( const Value& value ) |
|||
{ |
|||
return write_string( value, true ); |
|||
} |
|||
|
|||
#ifndef BOOST_NO_STD_WSTRING |
|||
|
|||
void json_spirit::write( const wValue& value, std::wostream& os ) |
|||
{ |
|||
write_stream( value, os, false ); |
|||
} |
|||
|
|||
void json_spirit::write_formatted( const wValue& value, std::wostream& os ) |
|||
{ |
|||
write_stream( value, os, true ); |
|||
} |
|||
|
|||
std::wstring json_spirit::write( const wValue& value ) |
|||
{ |
|||
return write_string( value, false ); |
|||
} |
|||
|
|||
std::wstring json_spirit::write_formatted( const wValue& value ) |
|||
{ |
|||
return write_string( value, true ); |
|||
} |
|||
|
|||
#if defined( JSON_SPIRIT_WVALUE_ENABLED ) && !defined( BOOST_NO_STD_WSTRING ) |
|||
void json_spirit::write( const wValue& value, std::wostream& os, unsigned int options ) |
|||
{ |
|||
write_stream( value, os, options ); |
|||
} |
|||
|
|||
std::wstring json_spirit::write( const wValue& value, unsigned int options ) |
|||
{ |
|||
return write_string( value, options ); |
|||
} |
|||
|
|||
void json_spirit::write_formatted( const wValue& value, std::wostream& os ) |
|||
{ |
|||
write_stream( value, os, pretty_print ); |
|||
} |
|||
|
|||
std::wstring json_spirit::write_formatted( const wValue& value ) |
|||
{ |
|||
return write_string( value, pretty_print ); |
|||
} |
|||
#endif |
|||
|
|||
#if defined( JSON_SPIRIT_WMVALUE_ENABLED ) && !defined( BOOST_NO_STD_WSTRING ) |
|||
void json_spirit::write_formatted( const wmValue& value, std::wostream& os ) |
|||
{ |
|||
write_stream( value, os, pretty_print ); |
|||
} |
|||
|
|||
std::wstring json_spirit::write_formatted( const wmValue& value ) |
|||
{ |
|||
return write_string( value, pretty_print ); |
|||
} |
|||
|
|||
void json_spirit::write( const wmValue& value, std::wostream& os, unsigned int options ) |
|||
{ |
|||
write_stream( value, os, options ); |
|||
} |
|||
|
|||
std::wstring json_spirit::write( const wmValue& value, unsigned int options ) |
|||
{ |
|||
return write_string( value, options ); |
|||
} |
|||
void json_spirit::write( const mValue& value, std::ostream& os ) |
|||
{ |
|||
write_stream( value, os, false ); |
|||
} |
|||
|
|||
void json_spirit::write_formatted( const mValue& value, std::ostream& os ) |
|||
{ |
|||
write_stream( value, os, true ); |
|||
} |
|||
|
|||
std::string json_spirit::write( const mValue& value ) |
|||
{ |
|||
return write_string( value, false ); |
|||
} |
|||
|
|||
std::string json_spirit::write_formatted( const mValue& value ) |
|||
{ |
|||
return write_string( value, true ); |
|||
} |
|||
|
|||
#ifndef BOOST_NO_STD_WSTRING |
|||
|
|||
void json_spirit::write( const wmValue& value, std::wostream& os ) |
|||
{ |
|||
write_stream( value, os, false ); |
|||
} |
|||
|
|||
void json_spirit::write_formatted( const wmValue& value, std::wostream& os ) |
|||
{ |
|||
write_stream( value, os, true ); |
|||
} |
|||
|
|||
std::wstring json_spirit::write( const wmValue& value ) |
|||
{ |
|||
return write_string( value, false ); |
|||
} |
|||
|
|||
std::wstring json_spirit::write_formatted( const wmValue& value ) |
|||
{ |
|||
return write_string( value, true ); |
|||
} |
|||
|
|||
#endif |
|||
|
@ -1,33 +0,0 @@ |
|||
#ifndef JSON_SPIRIT_WRITER_OPTIONS |
|||
#define JSON_SPIRIT_WRITER_OPTIONS |
|||
|
|||
// Copyright John W. Wilkinson 2007 - 2013
|
|||
// Distributed under the MIT License, see accompanying file LICENSE.txt
|
|||
|
|||
// json spirit version 4.06
|
|||
|
|||
#if defined(_MSC_VER) && (_MSC_VER >= 1020) |
|||
# pragma once |
|||
#endif |
|||
|
|||
namespace json_spirit |
|||
{ |
|||
enum Output_options{ pretty_print = 0x01, // Add whitespace to format the output nicely.
|
|||
|
|||
raw_utf8 = 0x02, // This prevents non-printable characters from being escapted using "\uNNNN" notation.
|
|||
// Note, this is an extension to the JSON standard. It disables the escaping of
|
|||
// non-printable characters allowing UTF-8 sequences held in 8 bit char strings
|
|||
// to pass through unaltered.
|
|||
|
|||
remove_trailing_zeros = 0x04, |
|||
// outputs e.g. "1.200000000000000" as "1.2"
|
|||
single_line_arrays = 0x08, |
|||
// pretty printing except that arrays printed on single lines unless they contain
|
|||
// composite elements, i.e. objects or arrays
|
|||
always_escape_nonascii = 0x10, |
|||
// all unicode wide characters are escaped, i.e. outputed as "\uXXXX", even if they are
|
|||
// printable under the current locale, ascii printable chars are not escaped
|
|||
}; |
|||
} |
|||
|
|||
#endif |
Loading…
Reference in new issue