Browse Source
Move the txid duplicates check into BuildMerkleTree, where it can be done much more efficiently (without needing to build a full txid set to detect duplicates). The previous version (using the std::set<uint256> to detect duplicates) was also slightly too weak. A block mined with actual duplicate transactions (which is invalid, due to the inputs of the duplicated transactions being seen as double spends) would trigger the duplicates logic, resulting in the block not being stored on disk, and rerequested. This change fixes that by only triggering in the case of duplicated transactions that can actually result in an identical merkle root.pull/145/head
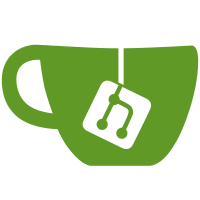
3 changed files with 57 additions and 17 deletions
Loading…
Reference in new issue