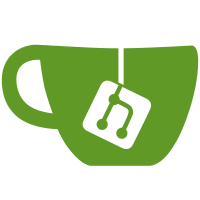
16 changed files with 220 additions and 185 deletions
@ -0,0 +1,140 @@ |
|||
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|||
// Copyright (c) 2009-2014 The Bitcoin developers
|
|||
// Distributed under the MIT/X11 software license, see the accompanying
|
|||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|||
|
|||
#include "random.h" |
|||
|
|||
#ifdef WIN32 |
|||
#include "compat.h" // for Windows API |
|||
#endif |
|||
#include "util.h" // for LogPrint() |
|||
|
|||
#ifndef WIN32 |
|||
#include <sys/time.h> |
|||
#endif |
|||
#include <cstring> // for memset() |
|||
|
|||
#include <openssl/crypto.h> |
|||
#include <openssl/err.h> |
|||
#include <openssl/rand.h> |
|||
|
|||
static inline int64_t GetPerformanceCounter() |
|||
{ |
|||
int64_t nCounter = 0; |
|||
#ifdef WIN32 |
|||
QueryPerformanceCounter((LARGE_INTEGER*)&nCounter); |
|||
#else |
|||
timeval t; |
|||
gettimeofday(&t, NULL); |
|||
nCounter = (int64_t)(t.tv_sec * 1000000 + t.tv_usec); |
|||
#endif |
|||
return nCounter; |
|||
} |
|||
|
|||
void RandAddSeed() |
|||
{ |
|||
// Seed with CPU performance counter
|
|||
int64_t nCounter = GetPerformanceCounter(); |
|||
RAND_add(&nCounter, sizeof(nCounter), 1.5); |
|||
memset(&nCounter, 0, sizeof(nCounter)); |
|||
} |
|||
|
|||
void RandAddSeedPerfmon() |
|||
{ |
|||
RandAddSeed(); |
|||
|
|||
// This can take up to 2 seconds, so only do it every 10 minutes
|
|||
static int64_t nLastPerfmon; |
|||
if (GetTime() < nLastPerfmon + 10 * 60) |
|||
return; |
|||
nLastPerfmon = GetTime(); |
|||
|
|||
#ifdef WIN32 |
|||
// Don't need this on Linux, OpenSSL automatically uses /dev/urandom
|
|||
// Seed with the entire set of perfmon data
|
|||
std::vector <unsigned char> vData(250000,0); |
|||
long ret = 0; |
|||
unsigned long nSize = 0; |
|||
const size_t nMaxSize = 10000000; // Bail out at more than 10MB of performance data
|
|||
while (true) |
|||
{ |
|||
nSize = vData.size(); |
|||
ret = RegQueryValueExA(HKEY_PERFORMANCE_DATA, "Global", NULL, NULL, begin_ptr(vData), &nSize); |
|||
if (ret != ERROR_MORE_DATA || vData.size() >= nMaxSize) |
|||
break; |
|||
vData.resize(std::max((vData.size()*3)/2, nMaxSize)); // Grow size of buffer exponentially
|
|||
} |
|||
RegCloseKey(HKEY_PERFORMANCE_DATA); |
|||
if (ret == ERROR_SUCCESS) |
|||
{ |
|||
RAND_add(begin_ptr(vData), nSize, nSize/100.0); |
|||
OPENSSL_cleanse(begin_ptr(vData), nSize); |
|||
LogPrint("rand", "%s: %lu bytes\n", __func__, nSize); |
|||
} else { |
|||
static bool warned = false; // Warn only once
|
|||
if (!warned) |
|||
{ |
|||
LogPrintf("%s: Warning: RegQueryValueExA(HKEY_PERFORMANCE_DATA) failed with code %i\n", __func__, ret); |
|||
warned = true; |
|||
} |
|||
} |
|||
#endif |
|||
} |
|||
|
|||
bool GetRandBytes(unsigned char *buf, int num) |
|||
{ |
|||
if (RAND_bytes(buf, num) != 1) { |
|||
LogPrintf("%s: OpenSSL RAND_bytes() failed with error: %s\n", __func__, ERR_error_string(ERR_get_error(), NULL)); |
|||
return false; |
|||
} |
|||
return true; |
|||
} |
|||
|
|||
uint64_t GetRand(uint64_t nMax) |
|||
{ |
|||
if (nMax == 0) |
|||
return 0; |
|||
|
|||
// The range of the random source must be a multiple of the modulus
|
|||
// to give every possible output value an equal possibility
|
|||
uint64_t nRange = (std::numeric_limits<uint64_t>::max() / nMax) * nMax; |
|||
uint64_t nRand = 0; |
|||
do { |
|||
GetRandBytes((unsigned char*)&nRand, sizeof(nRand)); |
|||
} while (nRand >= nRange); |
|||
return (nRand % nMax); |
|||
} |
|||
|
|||
int GetRandInt(int nMax) |
|||
{ |
|||
return GetRand(nMax); |
|||
} |
|||
|
|||
uint256 GetRandHash() |
|||
{ |
|||
uint256 hash; |
|||
GetRandBytes((unsigned char*)&hash, sizeof(hash)); |
|||
return hash; |
|||
} |
|||
|
|||
uint32_t insecure_rand_Rz = 11; |
|||
uint32_t insecure_rand_Rw = 11; |
|||
void seed_insecure_rand(bool fDeterministic) |
|||
{ |
|||
// The seed values have some unlikely fixed points which we avoid.
|
|||
if(fDeterministic) |
|||
{ |
|||
insecure_rand_Rz = insecure_rand_Rw = 11; |
|||
} else { |
|||
uint32_t tmp; |
|||
do { |
|||
GetRandBytes((unsigned char*)&tmp, 4); |
|||
} while(tmp == 0 || tmp == 0x9068ffffU); |
|||
insecure_rand_Rz = tmp; |
|||
do { |
|||
GetRandBytes((unsigned char*)&tmp, 4); |
|||
} while(tmp == 0 || tmp == 0x464fffffU); |
|||
insecure_rand_Rw = tmp; |
|||
} |
|||
} |
@ -0,0 +1,49 @@ |
|||
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
|||
// Copyright (c) 2009-2014 The Bitcoin developers
|
|||
// Distributed under the MIT/X11 software license, see the accompanying
|
|||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|||
|
|||
#ifndef BITCOIN_RANDOM_H |
|||
#define BITCOIN_RANDOM_H |
|||
|
|||
#include "uint256.h" |
|||
|
|||
#include <stdint.h> |
|||
|
|||
/**
|
|||
* Seed OpenSSL PRNG with additional entropy data |
|||
*/ |
|||
void RandAddSeed(); |
|||
void RandAddSeedPerfmon(); |
|||
|
|||
/**
|
|||
* Functions to gather random data via the OpenSSL PRNG |
|||
*/ |
|||
bool GetRandBytes(unsigned char *buf, int num); |
|||
uint64_t GetRand(uint64_t nMax); |
|||
int GetRandInt(int nMax); |
|||
uint256 GetRandHash(); |
|||
|
|||
/**
|
|||
* Seed insecure_rand using the random pool. |
|||
* @param Deterministic Use a determinstic seed |
|||
*/ |
|||
void seed_insecure_rand(bool fDeterministic = false); |
|||
|
|||
/**
|
|||
* MWC RNG of George Marsaglia |
|||
* This is intended to be fast. It has a period of 2^59.3, though the |
|||
* least significant 16 bits only have a period of about 2^30.1. |
|||
* |
|||
* @return random value |
|||
*/ |
|||
extern uint32_t insecure_rand_Rz; |
|||
extern uint32_t insecure_rand_Rw; |
|||
static inline uint32_t insecure_rand(void) |
|||
{ |
|||
insecure_rand_Rz = 36969 * (insecure_rand_Rz & 65535) + (insecure_rand_Rz >> 16); |
|||
insecure_rand_Rw = 18000 * (insecure_rand_Rw & 65535) + (insecure_rand_Rw >> 16); |
|||
return (insecure_rand_Rw << 16) + insecure_rand_Rz; |
|||
} |
|||
|
|||
#endif // BITCOIN_RANDOM_H
|
Loading…
Reference in new issue