Browse Source
While CBloomFilter is usually used with an explicitly set nTweak, CRollingBloomFilter is only used internally. Requiring every caller to set nTweak is error-prone and redundant; better to have the class handle that for you with a high-quality randomness source. Additionally when clearing the filter it makes sense to change nTweak as well to recover from a bad setting, e.g. due to insufficient randomness at initialization, so the clear() method is replaced by a reset() method that sets a new, random, nTweak value. (cherry picked from commit d2d7ee0e863b286e1c9f9c54659d494fb0a7712d)pull/145/head
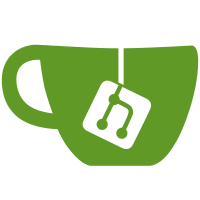
5 changed files with 29 additions and 12 deletions
Loading…
Reference in new issue