Browse Source
This makes it possible for a node with `-onlynet=tor` to bootstrap itself. It also adds the base infrastructure for adding IPv6 seed nodes. Also represent IPv4 fixed seed addresses in 16-byte format.pull/145/head
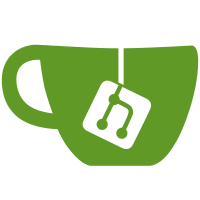
6 changed files with 1432 additions and 91 deletions
@ -0,0 +1,132 @@ |
|||
#!/usr/bin/python |
|||
# Copyright (c) 2014 Wladmir J. van der Laan |
|||
# Distributed under the MIT/X11 software license, see the accompanying |
|||
# file COPYING or http://www.opensource.org/licenses/mit-license.php. |
|||
''' |
|||
Script to generate list of seed nodes for chainparams.cpp. |
|||
|
|||
This script expects two text files in the directory that is passed as an |
|||
argument: |
|||
|
|||
nodes_main.txt |
|||
nodes_test.txt |
|||
|
|||
These files must consist of lines in the format |
|||
|
|||
<ip> |
|||
<ip>:<port> |
|||
[<ipv6>] |
|||
[<ipv6>]:<port> |
|||
<onion>.onion |
|||
0xDDBBCCAA (IPv4 little-endian old pnSeeds format) |
|||
|
|||
The output will be two data structures with the peers in binary format: |
|||
|
|||
static SeedSpec6 pnSeed6_main[]={ |
|||
... |
|||
} |
|||
static SeedSpec6 pnSeed6_test[]={ |
|||
... |
|||
} |
|||
|
|||
These should be pasted into `src/chainparamsseeds.h`. |
|||
''' |
|||
from __future__ import print_function, division |
|||
from base64 import b32decode |
|||
from binascii import a2b_hex |
|||
import sys, os |
|||
import re |
|||
|
|||
# ipv4 in ipv6 prefix |
|||
pchIPv4 = bytearray([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0xff, 0xff]) |
|||
# tor-specific ipv6 prefix |
|||
pchOnionCat = bytearray([0xFD,0x87,0xD8,0x7E,0xEB,0x43]) |
|||
|
|||
def name_to_ipv6(addr): |
|||
if len(addr)>6 and addr.endswith('.onion'): |
|||
vchAddr = b32decode(addr[0:-6], True) |
|||
if len(vchAddr) != 16-len(pchOnionCat): |
|||
raise ValueError('Invalid onion %s' % s) |
|||
return pchOnionCat + vchAddr |
|||
elif '.' in addr: # IPv4 |
|||
return pchIPv4 + bytearray((int(x) for x in addr.split('.'))) |
|||
elif ':' in addr: # IPv6 |
|||
sub = [[], []] # prefix, suffix |
|||
x = 0 |
|||
addr = addr.split(':') |
|||
for i,comp in enumerate(addr): |
|||
if comp == '': |
|||
if i == 0 or i == (len(addr)-1): # skip empty component at beginning or end |
|||
continue |
|||
x += 1 # :: skips to suffix |
|||
assert(x < 2) |
|||
else: # two bytes per component |
|||
val = int(comp, 16) |
|||
sub[x].append(val >> 8) |
|||
sub[x].append(val & 0xff) |
|||
nullbytes = 16 - len(sub[0]) - len(sub[1]) |
|||
assert((x == 0 and nullbytes == 0) or (x == 1 and nullbytes > 0)) |
|||
return bytearray(sub[0] + ([0] * nullbytes) + sub[1]) |
|||
elif addr.startswith('0x'): # IPv4-in-little-endian |
|||
return pchIPv4 + bytearray(reversed(a2b_hex(addr[2:]))) |
|||
else: |
|||
raise ValueError('Could not parse address %s' % addr) |
|||
|
|||
def parse_spec(s, defaultport): |
|||
match = re.match('\[([0-9a-fA-F:]+)\](?::([0-9]+))?$', s) |
|||
if match: # ipv6 |
|||
host = match.group(1) |
|||
port = match.group(2) |
|||
else: |
|||
(host,_,port) = s.partition(':') |
|||
|
|||
if not port: |
|||
port = defaultport |
|||
else: |
|||
port = int(port) |
|||
|
|||
host = name_to_ipv6(host) |
|||
|
|||
return (host,port) |
|||
|
|||
def process_nodes(g, f, structname, defaultport): |
|||
g.write('static SeedSpec6 %s[] = {\n' % structname) |
|||
first = True |
|||
for line in f: |
|||
comment = line.find('#') |
|||
if comment != -1: |
|||
line = line[0:comment] |
|||
line = line.strip() |
|||
if not line: |
|||
continue |
|||
if not first: |
|||
g.write(',\n') |
|||
first = False |
|||
|
|||
(host,port) = parse_spec(line, defaultport) |
|||
hoststr = ','.join(('0x%02x' % b) for b in host) |
|||
g.write(' {{%s}, %i}' % (hoststr, port)) |
|||
g.write('\n};\n') |
|||
|
|||
def main(): |
|||
if len(sys.argv)<2: |
|||
print(('Usage: %s <path_to_nodes_txt>' % sys.argv[0]), file=sys.stderr) |
|||
exit(1) |
|||
g = sys.stdout |
|||
indir = sys.argv[1] |
|||
g.write('#ifndef H_CHAINPARAMSSEEDS\n') |
|||
g.write('#define H_CHAINPARAMSSEEDS\n') |
|||
g.write('// List of fixed seed nodes for the bitcoin network\n') |
|||
g.write('// AUTOGENERATED by contrib/devtools/generate-seeds.py\n\n') |
|||
g.write('// Each line contains a 16-byte IPv6 address and a port.\n') |
|||
g.write('// IPv4 as well as onion addresses are wrapped inside a IPv6 address accordingly.\n') |
|||
with open(os.path.join(indir,'nodes_main.txt'),'r') as f: |
|||
process_nodes(g, f, 'pnSeed6_main', 8333) |
|||
g.write('\n') |
|||
with open(os.path.join(indir,'nodes_test.txt'),'r') as f: |
|||
process_nodes(g, f, 'pnSeed6_test', 18333) |
|||
g.write('#endif\n') |
|||
|
|||
if __name__ == '__main__': |
|||
main() |
|||
|
@ -0,0 +1,629 @@ |
|||
# List of fixed seed nodes for main network |
|||
|
|||
# IPv4 nodes (in old chainparams.cpp 0xDDCCBBAA format) |
|||
# n.b. when importing a new list, there is no need to use this format, just use IPv4 dotted addresses directly |
|||
0x7e6a692e # 46.105.106.126 |
|||
0x7d04d1a2 # 162.209.4.125 |
|||
0x6c0c17d9 # 217.23.12.108 |
|||
0xdb330ab9 # 185.10.51.219 |
|||
0xc649c7c6 # 198.199.73.198 |
|||
0x7895484d # 77.72.149.120 |
|||
0x047109b0 # 176.9.113.4 |
|||
0xb90ca5bc # 188.165.12.185 |
|||
0xd130805f # 95.128.48.209 |
|||
0xbd074ea6 # 166.78.7.189 |
|||
0x578ff1c0 # 192.241.143.87 |
|||
0x286e09b0 # 176.9.110.40 |
|||
0xd4dcaf42 # 66.175.220.212 |
|||
0x529b6bb8 # 184.107.155.82 |
|||
0x635cc6c0 # 192.198.92.99 |
|||
0xedde892e # 46.137.222.237 |
|||
0xa976d9c7 # 199.217.118.169 |
|||
0xea91a4b8 # 184.164.145.234 |
|||
0x03fa4eb2 # 178.78.250.3 |
|||
0x6ca9008d # 141.0.169.108 |
|||
0xaf62c825 # 37.200.98.175 |
|||
0x93f3ba51 # 81.186.243.147 |
|||
0xc2c9efd5 # 213.239.201.194 |
|||
0x0ed5175e # 94.23.213.14 |
|||
0x487028bc # 188.40.112.72 |
|||
0x7297c225 # 37.194.151.114 |
|||
0x8af0c658 # 88.198.240.138 |
|||
0x2e57ba1f # 31.186.87.46 |
|||
0xd0098abc # 188.138.9.208 |
|||
0x46a8853e # 62.133.168.70 |
|||
0xcc92dc3e # 62.220.146.204 |
|||
0xeb6f1955 # 85.25.111.235 |
|||
0x8cce175e # 94.23.206.140 |
|||
0x237281ae # 174.129.114.35 |
|||
0x9d42795b # 91.121.66.157 |
|||
0x4f4f0905 # 5.9.79.79 |
|||
0xc50151d0 # 208.81.1.197 |
|||
0xb1ba90c6 # 198.144.186.177 |
|||
0xaed7175e # 94.23.215.174 |
|||
0x204de55b # 91.229.77.32 |
|||
0x4bb03245 # 69.50.176.75 |
|||
0x932b28bc # 188.40.43.147 |
|||
0x2dcce65b # 91.230.204.45 |
|||
0xe2708abc # 188.138.112.226 |
|||
0x1b08b8d5 # 213.184.8.27 |
|||
0x12a3dc5b # 91.220.163.18 |
|||
0x8a884c90 # 144.76.136.138 |
|||
0xa386a8b8 # 184.168.134.163 |
|||
0x18e417c6 # 198.23.228.24 |
|||
0x2e709ac3 # 195.154.112.46 |
|||
0xeb62e925 # 37.233.98.235 |
|||
0x6f6503ae # 174.3.101.111 |
|||
0x05d0814e # 78.129.208.5 |
|||
0x8a9ac545 # 69.197.154.138 |
|||
0x946fd65e # 94.214.111.148 |
|||
0x3f57495d # 93.73.87.63 |
|||
0x4a29c658 # 88.198.41.74 |
|||
0xad454c90 # 144.76.69.173 |
|||
0x15340905 # 5.9.52.21 |
|||
0x4c3f3b25 # 37.59.63.76 |
|||
0x01fe19b9 # 185.25.254.1 |
|||
0x5620595b # 91.89.32.86 |
|||
0x443c795b # 91.121.60.68 |
|||
0x44f24ac8 # 200.74.242.68 |
|||
0x0442464e # 78.70.66.4 |
|||
0xc8665882 # 130.88.102.200 |
|||
0xed3f3ec3 # 195.62.63.237 |
|||
0xf585bf5d # 93.191.133.245 |
|||
0x5dd141da # 218.65.209.93 |
|||
0xf93a084e # 78.8.58.249 |
|||
0x1264dd52 # 82.221.100.18 |
|||
0x0711c658 # 88.198.17.7 |
|||
0xf12e7bbe # 190.123.46.241 |
|||
0x5b02b740 # 64.183.2.91 |
|||
0x7d526dd5 # 213.109.82.125 |
|||
0x0cb04c90 # 144.76.176.12 |
|||
0x2abe1132 # 50.17.190.42 |
|||
0x61a39f58 # 88.159.163.97 |
|||
0x044a0618 # 24.6.74.4 |
|||
0xf3af7dce # 206.125.175.243 |
|||
0xb994c96d # 109.201.148.185 |
|||
0x361c5058 # 88.80.28.54 |
|||
0xca735d53 # 83.93.115.202 |
|||
0xeca743b0 # 176.67.167.236 |
|||
0xec790905 # 5.9.121.236 |
|||
0xc4d37845 # 69.120.211.196 |
|||
0xa1c4a2b2 # 178.162.196.161 |
|||
0x726fd453 # 83.212.111.114 |
|||
0x625cc6c0 # 192.198.92.98 |
|||
0x6c20132e # 46.19.32.108 |
|||
0xb7aa0c79 # 121.12.170.183 |
|||
0xc6ed983d # 61.152.237.198 |
|||
0x47e4cbc0 # 192.203.228.71 |
|||
0xa4ac75d4 # 212.117.172.164 |
|||
0xe2e59345 # 69.147.229.226 |
|||
0x4d784ad0 # 208.74.120.77 |
|||
0x18a5ec5e # 94.236.165.24 |
|||
0x481cc85b # 91.200.28.72 |
|||
0x7c6c2fd5 # 213.47.108.124 |
|||
0x5e4d6018 # 24.96.77.94 |
|||
0x5b4b6c18 # 24.108.75.91 |
|||
0xd99b4c90 # 144.76.155.217 |
|||
0xe63987dc # 220.135.57.230 |
|||
0xb817bb25 # 37.187.23.184 |
|||
0x141cfeb2 # 178.254.28.20 |
|||
0x5f005058 # 88.80.0.95 |
|||
0x0d987f47 # 71.127.152.13 |
|||
0x242a496d # 109.73.42.36 |
|||
0x3e519bc0 # 192.155.81.62 |
|||
0x02b2454b # 75.69.178.2 |
|||
0xdfaf3dc6 # 198.61.175.223 |
|||
0x888128bc # 188.40.129.136 |
|||
0x1165bb25 # 37.187.101.17 |
|||
0xabfeca5b # 91.202.254.171 |
|||
0x2ef63540 # 64.53.246.46 |
|||
0x5773c7c6 # 198.199.115.87 |
|||
0x1280dd52 # 82.221.128.18 |
|||
0x8ebcacd9 # 217.172.188.142 |
|||
0x81c439c6 # 198.57.196.129 |
|||
0x39fcfa45 # 69.250.252.57 |
|||
0x62177d41 # 65.125.23.98 |
|||
0xc975ed62 # 98.237.117.201 |
|||
0x05cff476 # 118.244.207.5 |
|||
0xdabda743 # 67.167.189.218 |
|||
0xaa1ac24e # 78.194.26.170 |
|||
0xe255a22e # 46.162.85.226 |
|||
0x88aac705 # 5.199.170.136 |
|||
0xe707c658 # 88.198.7.231 |
|||
0xa9e94b5e # 94.75.233.169 |
|||
0x2893484b # 75.72.147.40 |
|||
0x99512705 # 5.39.81.153 |
|||
0xd63970ca # 202.112.57.214 |
|||
0x45994f32 # 50.79.153.69 |
|||
0xe519a8ad # 173.168.25.229 |
|||
0x92e25f5d # 93.95.226.146 |
|||
0x8b84a9c1 # 193.169.132.139 |
|||
0x5eaa0a05 # 5.10.170.94 |
|||
0xa74de55b # 91.229.77.167 |
|||
0xb090ff62 # 98.255.144.176 |
|||
0x5eee326c # 108.50.238.94 |
|||
0xc331a679 # 121.166.49.195 |
|||
0xc1d9b72e # 46.183.217.193 |
|||
0x0c6ab982 # 130.185.106.12 |
|||
0x7362bb25 # 37.187.98.115 |
|||
0x4cfedd42 # 66.221.254.76 |
|||
0x1e09a032 # 50.160.9.30 |
|||
0xa4c34c5e # 94.76.195.164 |
|||
0x3777d9c7 # 199.217.119.55 |
|||
0x5edcf260 # 96.242.220.94 |
|||
0x3ce2b548 # 72.181.226.60 |
|||
0xd2ac0360 # 96.3.172.210 |
|||
0x2f80b992 # 146.185.128.47 |
|||
0x3e4cbb25 # 37.187.76.62 |
|||
0x3995e236 # 54.226.149.57 |
|||
0xd03977ae # 174.119.57.208 |
|||
0x953cf054 # 84.240.60.149 |
|||
0x3c654ed0 # 208.78.101.60 |
|||
0x74024c90 # 144.76.2.116 |
|||
0xa14f1155 # 85.17.79.161 |
|||
0x14ce0125 # 37.1.206.20 |
|||
0xc15ebb6a # 106.187.94.193 |
|||
0x2c08c452 # 82.196.8.44 |
|||
0xc7fd0652 # 82.6.253.199 |
|||
0x7604f8ce # 206.248.4.118 |
|||
0xffb38332 # 50.131.179.255 |
|||
0xa4c2efd5 # 213.239.194.164 |
|||
0xe9614018 # 24.64.97.233 |
|||
0xab49e557 # 87.229.73.171 |
|||
0x1648c052 # 82.192.72.22 |
|||
0x36024047 # 71.64.2.54 |
|||
0x0e8cffad # 173.255.140.14 |
|||
0x21918953 # 83.137.145.33 |
|||
0xb61f50ad # 173.80.31.182 |
|||
0x9b406b59 # 89.107.64.155 |
|||
0xaf282218 # 24.34.40.175 |
|||
0x7f1d164e # 78.22.29.127 |
|||
0x1f560da2 # 162.13.86.31 |
|||
0xe237be58 # 88.190.55.226 |
|||
0xbdeb1955 # 85.25.235.189 |
|||
0x6c0717d9 # 217.23.7.108 |
|||
0xdaf8ce62 # 98.206.248.218 |
|||
0x0f74246c # 108.36.116.15 |
|||
0xdee95243 # 67.82.233.222 |
|||
0xf23f1a56 # 86.26.63.242 |
|||
0x61bdf867 # 103.248.189.97 |
|||
0xd254c854 # 84.200.84.210 |
|||
0xc4422e4e # 78.46.66.196 |
|||
0xae0563c0 # 192.99.5.174 |
|||
0xbdb9a95f # 95.169.185.189 |
|||
0xa9eb32c6 # 198.50.235.169 |
|||
0xd9943950 # 80.57.148.217 |
|||
0x116add52 # 82.221.106.17 |
|||
0x73a54c90 # 144.76.165.115 |
|||
0xb36b525e # 94.82.107.179 |
|||
0xd734175e # 94.23.52.215 |
|||
0x333d7f76 # 118.127.61.51 |
|||
0x51431bc6 # 198.27.67.81 |
|||
0x084ae5cf # 207.229.74.8 |
|||
0xa60a236c # 108.35.10.166 |
|||
0x5c67692e # 46.105.103.92 |
|||
0x0177cf45 # 69.207.119.1 |
|||
0xa6683ac6 # 198.58.104.166 |
|||
0x7ff4ea47 # 71.234.244.127 |
|||
0x2192fab2 # 178.250.146.33 |
|||
0xa03a0f46 # 70.15.58.160 |
|||
0xfe3e39ae # 174.57.62.254 |
|||
0x2cce5fc1 # 193.95.206.44 |
|||
0xc8a6c148 # 72.193.166.200 |
|||
0x96fb7e4c # 76.126.251.150 |
|||
0x0a66c752 # 82.199.102.10 |
|||
0x6b4d2705 # 5.39.77.107 |
|||
0xeba0c118 # 24.193.160.235 |
|||
0x3ba0795b # 91.121.160.59 |
|||
0x1dccd23e # 62.210.204.29 |
|||
0x6912f3a2 # 162.243.18.105 |
|||
0x22f23c41 # 65.60.242.34 |
|||
0x65646b4a # 74.107.100.101 |
|||
0x8b9f8705 # 5.135.159.139 |
|||
0xeb9b9a95 # 149.154.155.235 |
|||
0x79fe6b4e # 78.107.254.121 |
|||
0x0536f447 # 71.244.54.5 |
|||
0x23224d61 # 97.77.34.35 |
|||
0x5d952ec6 # 198.46.149.93 |
|||
0x0cb4f736 # 54.247.180.12 |
|||
0xdc14be6d # 109.190.20.220 |
|||
0xb24609b0 # 176.9.70.178 |
|||
0xd3f79b62 # 98.155.247.211 |
|||
0x6518c836 # 54.200.24.101 |
|||
0x83a3cf42 # 66.207.163.131 |
|||
0x9b641fb0 # 176.31.100.155 |
|||
0x17fef1c0 # 192.241.254.23 |
|||
0xd508cc82 # 130.204.8.213 |
|||
0x91a4369b # 155.54.164.145 |
|||
0x39cb4a4c # 76.74.203.57 |
|||
0xbbc9536c # 108.83.201.187 |
|||
0xaf64c44a # 74.196.100.175 |
|||
0x605eca50 # 80.202.94.96 |
|||
0x0c6a6805 # 5.104.106.12 |
|||
0xd07e9d4e # 78.157.126.208 |
|||
0x78e6d3a2 # 162.211.230.120 |
|||
0x1b31eb6d # 109.235.49.27 |
|||
0xaa01feb2 # 178.254.1.170 |
|||
0x4603c236 # 54.194.3.70 |
|||
0x1ecba3b6 # 182.163.203.30 |
|||
0x0effe336 # 54.227.255.14 |
|||
0xc3fdcb36 # 54.203.253.195 |
|||
0xc290036f # 111.3.144.194 |
|||
0x4464692e # 46.105.100.68 |
|||
0x1aca7589 # 137.117.202.26 |
|||
0x59a9e52e # 46.229.169.89 |
|||
0x19aa7489 # 137.116.170.25 |
|||
0x2622c85e # 94.200.34.38 |
|||
0xa598d318 # 24.211.152.165 |
|||
0x438ec345 # 69.195.142.67 |
|||
0xc79619b9 # 185.25.150.199 |
|||
0xaf570360 # 96.3.87.175 |
|||
0x5098e289 # 137.226.152.80 |
|||
0x36add862 # 98.216.173.54 |
|||
0x83c1a2b2 # 178.162.193.131 |
|||
0x969d0905 # 5.9.157.150 |
|||
0xcf3d156c # 108.21.61.207 |
|||
0x49c1a445 # 69.164.193.73 |
|||
0xbd0b7562 # 98.117.11.189 |
|||
0x8fff1955 # 85.25.255.143 |
|||
0x1e51fe53 # 83.254.81.30 |
|||
0x28d6efd5 # 213.239.214.40 |
|||
0x2837cc62 # 98.204.55.40 |
|||
0x02f42d42 # 66.45.244.2 |
|||
0x070e3fb2 # 178.63.14.7 |
|||
0xbcb18705 # 5.135.177.188 |
|||
0x14a4e15b # 91.225.164.20 |
|||
0x82096844 # 68.104.9.130 |
|||
0xcfcb1c2e # 46.28.203.207 |
|||
0x37e27fc7 # 199.127.226.55 |
|||
0x07923748 # 72.55.146.7 |
|||
0x0c14bc2e # 46.188.20.12 |
|||
0x26100905 # 5.9.16.38 |
|||
0xcb7cd93e # 62.217.124.203 |
|||
0x3bc0d2c0 # 192.210.192.59 |
|||
0x97131b4c # 76.27.19.151 |
|||
0x6f1e5c17 # 23.92.30.111 |
|||
0xa7939f43 # 67.159.147.167 |
|||
0xb7a0bf58 # 88.191.160.183 |
|||
0xafa83a47 # 71.58.168.175 |
|||
0xcbb83f32 # 50.63.184.203 |
|||
0x5f321cb0 # 176.28.50.95 |
|||
0x52d6c3c7 # 199.195.214.82 |
|||
0xdeac5bc7 # 199.91.172.222 |
|||
0x2cf310cc # 204.16.243.44 |
|||
0x108a2bc3 # 195.43.138.16 |
|||
0x726fa14f # 79.161.111.114 |
|||
0x85bad2cc # 204.210.186.133 |
|||
0x459e4c90 # 144.76.158.69 |
|||
0x1a08b8d8 # 216.184.8.26 |
|||
0xcd7048c6 # 198.72.112.205 |
|||
0x6d5b4c90 # 144.76.91.109 |
|||
0xa66cfe7b # 123.254.108.166 |
|||
0xad730905 # 5.9.115.173 |
|||
0xdaac5bc7 # 199.91.172.218 |
|||
0x8417fd9f # 159.253.23.132 |
|||
0x41377432 # 50.116.55.65 |
|||
0x1f138632 # 50.134.19.31 |
|||
0x295a12b2 # 178.18.90.41 |
|||
0x7ac031b2 # 178.49.192.122 |
|||
0x3a87d295 # 149.210.135.58 |
|||
0xe219bc2e # 46.188.25.226 |
|||
0xf485d295 # 149.210.133.244 |
|||
0x137b6405 # 5.100.123.19 |
|||
0xcfffd9ad # 173.217.255.207 |
|||
0xafe20844 # 68.8.226.175 |
|||
0x32679a5f # 95.154.103.50 |
|||
0xa431c644 # 68.198.49.164 |
|||
0x0e5fce8c # 140.206.95.14 |
|||
0x305ef853 # 83.248.94.48 |
|||
0xad26ca32 # 50.202.38.173 |
|||
0xd9d21a54 # 84.26.210.217 |
|||
0xddd0d736 # 54.215.208.221 |
|||
0xc24ec0c7 # 199.192.78.194 |
|||
0x4aadcd5b # 91.205.173.74 |
|||
0x49109852 # 82.152.16.73 |
|||
0x9d6b3ac6 # 198.58.107.157 |
|||
0xf0aa1e8b # 139.30.170.240 |
|||
0xf1bfa343 # 67.163.191.241 |
|||
0x8a30c0ad # 173.192.48.138 |
|||
0x260f93d4 # 212.147.15.38 |
|||
0x2339e760 # 96.231.57.35 |
|||
0x8869959f # 159.149.105.136 |
|||
0xc207216c # 108.33.7.194 |
|||
0x29453448 # 72.52.69.41 |
|||
0xb651ec36 # 54.236.81.182 |
|||
0x45496259 # 89.98.73.69 |
|||
0xa23d1bcc # 204.27.61.162 |
|||
0xb39bcf43 # 67.207.155.179 |
|||
0xa1d29432 # 50.148.210.161 |
|||
0x3507c658 # 88.198.7.53 |
|||
0x4a88dd62 # 98.221.136.74 |
|||
0x27aff363 # 99.243.175.39 |
|||
0x7498ea6d # 109.234.152.116 |
|||
0x4a6785d5 # 213.133.103.74 |
|||
0x5e6d47c2 # 194.71.109.94 |
|||
0x3baba542 # 66.165.171.59 |
|||
0x045a37ae # 174.55.90.4 |
|||
0xa24dc0c7 # 199.192.77.162 |
|||
0xe981ea4d # 77.234.129.233 |
|||
0xed6ce217 # 23.226.108.237 |
|||
0x857214c6 # 198.20.114.133 |
|||
0x6b6c0464 # 100.4.108.107 |
|||
0x5a4945b8 # 184.69.73.90 |
|||
0x12f24742 # 66.71.242.18 |
|||
0xf35f42ad # 173.66.95.243 |
|||
0xfd0f5a4e # 78.90.15.253 |
|||
0xfb081556 # 86.21.8.251 |
|||
0xb24b5861 # 97.88.75.178 |
|||
0x2e114146 # 70.65.17.46 |
|||
0xb7780905 # 5.9.120.183 |
|||
0x33bb0e48 # 72.14.187.51 |
|||
0x39e26556 # 86.101.226.57 |
|||
0xa794484d # 77.72.148.167 |
|||
0x4225424d # 77.66.37.66 |
|||
0x3003795b # 91.121.3.48 |
|||
0x31c8cf44 # 68.207.200.49 |
|||
0xd65bad59 # 89.173.91.214 |
|||
0x127bc648 # 72.198.123.18 |
|||
0xf2bc4d4c # 76.77.188.242 |
|||
0x0273dc50 # 80.220.115.2 |
|||
0x4572d736 # 54.215.114.69 |
|||
0x064bf653 # 83.246.75.6 |
|||
0xcdcd126c # 108.18.205.205 |
|||
0x608281ae # 174.129.130.96 |
|||
0x4d130087 # 135.0.19.77 |
|||
0x1016f725 # 37.247.22.16 |
|||
0xba185fc0 # 192.95.24.186 |
|||
0x16c1a84f # 79.168.193.22 |
|||
0xfb697252 # 82.114.105.251 |
|||
0xa2942360 # 96.35.148.162 |
|||
0x53083b6c # 108.59.8.83 |
|||
0x0583f1c0 # 192.241.131.5 |
|||
0x2d5a2441 # 65.36.90.45 |
|||
0xc172aa43 # 67.170.114.193 |
|||
0xcd11cf36 # 54.207.17.205 |
|||
0x7b14ed62 # 98.237.20.123 |
|||
0x5c94f1c0 # 192.241.148.92 |
|||
0x7c23132e # 46.19.35.124 |
|||
0x39965a6f # 111.90.150.57 |
|||
0x7890e24e # 78.226.144.120 |
|||
0xa38ec447 # 71.196.142.163 |
|||
0xc187f1c0 # 192.241.135.193 |
|||
0xef80b647 # 71.182.128.239 |
|||
0xf20a7432 # 50.116.10.242 |
|||
0x7ad1d8d2 # 210.216.209.122 |
|||
0x869e2ec6 # 198.46.158.134 |
|||
0xccdb5c5d # 93.92.219.204 |
|||
0x9d11f636 # 54.246.17.157 |
|||
0x2161bb25 # 37.187.97.33 |
|||
0x7599f889 # 137.248.153.117 |
|||
0x2265ecad # 173.236.101.34 |
|||
0x0f4f0e55 # 85.14.79.15 |
|||
0x7d25854a # 74.133.37.125 |
|||
0xf857e360 # 96.227.87.248 |
|||
0xf83f3d6c # 108.61.63.248 |
|||
0x9cc93bb8 # 184.59.201.156 |
|||
0x02716857 # 87.104.113.2 |
|||
0x5dd8a177 # 119.161.216.93 |
|||
0x8adc6cd4 # 212.108.220.138 |
|||
0xe5613d46 # 70.61.97.229 |
|||
0x6a734f50 # 80.79.115.106 |
|||
0x2a5c3bae # 174.59.92.42 |
|||
0x4a04c3d1 # 209.195.4.74 |
|||
0xe4613d46 # 70.61.97.228 |
|||
0x8426f4bc # 188.244.38.132 |
|||
0x3e1b5fc0 # 192.95.27.62 |
|||
0x0d5a3c18 # 24.60.90.13 |
|||
0xd0f6d154 # 84.209.246.208 |
|||
0x21c7ff5e # 94.255.199.33 |
|||
0xeb3f3d6c # 108.61.63.235 |
|||
0x9da5edc0 # 192.237.165.157 |
|||
0x5d753b81 # 129.59.117.93 |
|||
0x0d8d53d4 # 212.83.141.13 |
|||
0x2613f018 # 24.240.19.38 |
|||
0x4443698d # 141.105.67.68 |
|||
0x8ca1edcd # 205.237.161.140 |
|||
0x10ed3f4e # 78.63.237.16 |
|||
0x789b403a # 58.64.155.120 |
|||
0x7b984a4b # 75.74.152.123 |
|||
0x964ebc25 # 37.188.78.150 |
|||
0x7520ee60 # 96.238.32.117 |
|||
0x4f4828bc # 188.40.72.79 |
|||
0x115c407d # 125.64.92.17 |
|||
0x32dd0667 # 103.6.221.50 |
|||
0xa741715e # 94.113.65.167 |
|||
0x1d3f3532 # 50.53.63.29 |
|||
0x817d1f56 # 86.31.125.129 |
|||
0x2f99a552 # 82.165.153.47 |
|||
0x6b2a5956 # 86.89.42.107 |
|||
0x8d4f4f05 # 5.79.79.141 |
|||
0xd23c1e17 # 23.30.60.210 |
|||
0x98993748 # 72.55.153.152 |
|||
0x2c92e536 # 54.229.146.44 |
|||
0x237ebdc3 # 195.189.126.35 |
|||
0xa762fb43 # 67.251.98.167 |
|||
0x32016b71 # 113.107.1.50 |
|||
0xd0e7cf79 # 121.207.231.208 |
|||
0x7d35bdd5 # 213.189.53.125 |
|||
0x53dac3d2 # 210.195.218.83 |
|||
0x31016b71 # 113.107.1.49 |
|||
0x7fb8f8ce # 206.248.184.127 |
|||
0x9a38c232 # 50.194.56.154 |
|||
0xefaa42ad # 173.66.170.239 |
|||
0x876b823d # 61.130.107.135 |
|||
0x18175347 # 71.83.23.24 |
|||
0xdb46597d # 125.89.70.219 |
|||
0xd2c168da # 218.104.193.210 |
|||
0xcd6fe9dc # 220.233.111.205 |
|||
0x45272e4e # 78.46.39.69 |
|||
0x8d4bca5b # 91.202.75.141 |
|||
0xa4043d47 # 71.61.4.164 |
|||
0xaab7aa47 # 71.170.183.170 |
|||
0x202881ae # 174.129.40.32 |
|||
0xa4aef160 # 96.241.174.164 |
|||
0xecd7e6bc # 188.230.215.236 |
|||
0x391359ad # 173.89.19.57 |
|||
0xd8cc9318 # 24.147.204.216 |
|||
0xbbeee52e # 46.229.238.187 |
|||
0x077067b0 # 176.103.112.7 |
|||
0xebd39d62 # 98.157.211.235 |
|||
0x0cedc547 # 71.197.237.12 |
|||
0x23d3e15e # 94.225.211.35 |
|||
0xa5a81318 # 24.19.168.165 |
|||
0x179a32c6 # 198.50.154.23 |
|||
0xe4d3483d # 61.72.211.228 |
|||
0x03680905 # 5.9.104.3 |
|||
0xe8018abc # 188.138.1.232 |
|||
0xdde9ef5b # 91.239.233.221 |
|||
0x438b8705 # 5.135.139.67 |
|||
0xb48224a0 # 160.36.130.180 |
|||
0xcbd69218 # 24.146.214.203 |
|||
0x9075795b # 91.121.117.144 |
|||
0xc6411c3e # 62.28.65.198 |
|||
0x03833f5c # 92.63.131.3 |
|||
0xf33f8b5e # 94.139.63.243 |
|||
0x495e464b # 75.70.94.73 |
|||
0x83c8e65b # 91.230.200.131 |
|||
0xac09cd25 # 37.205.9.172 |
|||
0xdaabc547 # 71.197.171.218 |
|||
0x7665a553 # 83.165.101.118 |
|||
0xc5263718 # 24.55.38.197 |
|||
0x2fd0c5cd # 205.197.208.47 |
|||
0x22224d61 # 97.77.34.34 |
|||
0x3e954048 # 72.64.149.62 |
|||
0xfaa37557 # 87.117.163.250 |
|||
0x36dbc658 # 88.198.219.54 |
|||
0xa81453d0 # 208.83.20.168 |
|||
0x5a941f5d # 93.31.148.90 |
|||
0xa598ea60 # 96.234.152.165 |
|||
0x65384ac6 # 198.74.56.101 |
|||
0x10aaa545 # 69.165.170.16 |
|||
0xaaab795b # 91.121.171.170 |
|||
0xdda7024c # 76.2.167.221 |
|||
0x0966f4c6 # 198.244.102.9 |
|||
0x68571c08 # 8.28.87.104 |
|||
0x8b40ee59 # 89.238.64.139 |
|||
0x33ac096c # 108.9.172.51 |
|||
0x844b4c4b # 75.76.75.132 |
|||
0xd392254d # 77.37.146.211 |
|||
0xba4d5a46 # 70.90.77.186 |
|||
0x63029653 # 83.150.2.99 |
|||
0xf655f636 # 54.246.85.246 |
|||
0xbe4c4bb1 # 177.75.76.190 |
|||
0x45dad036 # 54.208.218.69 |
|||
0x204bc052 # 82.192.75.32 |
|||
0x06c3a2b2 # 178.162.195.6 |
|||
0xf31fba6a # 106.186.31.243 |
|||
0xb21f09b0 # 176.9.31.178 |
|||
0x540d0751 # 81.7.13.84 |
|||
0xc7b46a57 # 87.106.180.199 |
|||
0x6a11795b # 91.121.17.106 |
|||
0x3d514045 # 69.64.81.61 |
|||
0x0318aa6d # 109.170.24.3 |
|||
0x30306ec3 # 195.110.48.48 |
|||
0x5c077432 # 50.116.7.92 |
|||
0x259ae46d # 109.228.154.37 |
|||
0x82bbd35f # 95.211.187.130 |
|||
0xae4222c0 # 192.34.66.174 |
|||
0x254415d4 # 212.21.68.37 |
|||
0xbd5f574b # 75.87.95.189 |
|||
0xd8fd175e # 94.23.253.216 |
|||
0x0a3f38c3 # 195.56.63.10 |
|||
0x2dce6bb8 # 184.107.206.45 |
|||
0xc201d058 # 88.208.1.194 |
|||
0x17fca5bc # 188.165.252.23 |
|||
0xe8453cca # 202.60.69.232 |
|||
0xd361f636 # 54.246.97.211 |
|||
0xa0d9edc0 # 192.237.217.160 |
|||
0x2f232e4e # 78.46.35.47 |
|||
0x134e116c # 108.17.78.19 |
|||
0x61ddc058 # 88.192.221.97 |
|||
0x05ba7283 # 131.114.186.5 |
|||
0xe1f7ed5b # 91.237.247.225 |
|||
0x040ec452 # 82.196.14.4 |
|||
0x4b672e4e # 78.46.103.75 |
|||
0xe4efa36d # 109.163.239.228 |
|||
0x47dca52e # 46.165.220.71 |
|||
0xe9332e4e # 78.46.51.233 |
|||
0xa3acb992 # 146.185.172.163 |
|||
0x24714c90 # 144.76.113.36 |
|||
0xa8cc8632 # 50.134.204.168 |
|||
0x26b1ce6d # 109.206.177.38 |
|||
0x264e53d4 # 212.83.78.38 |
|||
0xd3d2718c # 140.113.210.211 |
|||
0x225534ad # 173.52.85.34 |
|||
0xe289f3a2 # 162.243.137.226 |
|||
0x87341717 # 23.23.52.135 |
|||
0x9255ad4f # 79.173.85.146 |
|||
0x184bbb25 # 37.187.75.24 |
|||
0x885c7abc # 188.122.92.136 |
|||
0x3a6e9ac6 # 198.154.110.58 |
|||
0x1924185e # 94.24.36.25 |
|||
0xb73d4c90 # 144.76.61.183 |
|||
0x946d807a # 122.128.109.148 |
|||
0xa0d78e3f # 63.142.215.160 |
|||
0x5a16bb25 # 37.187.22.90 |
|||
0xcb09795b # 91.121.9.203 |
|||
0x8d0de657 # 87.230.13.141 |
|||
0x630b8b25 # 37.139.11.99 |
|||
0xe572c6cf # 207.198.114.229 |
|||
0x2b3f1118 # 24.17.63.43 |
|||
0x4242a91f # 31.169.66.66 |
|||
0x32990905 # 5.9.153.50 |
|||
0x058b0905 # 5.9.139.5 |
|||
0xe266fc60 # 96.252.102.226 |
|||
0xbe66c5b0 # 176.197.102.190 |
|||
0xcc98e46d # 109.228.152.204 |
|||
0x698c943e # 62.148.140.105 |
|||
0x44bd0cc3 # 195.12.189.68 |
|||
0x865c7abc # 188.122.92.134 |
|||
0x771764d3 # 211.100.23.119 |
|||
0x4675d655 # 85.214.117.70 |
|||
0x354e4826 # 38.72.78.53 |
|||
0xb67ac152 # 82.193.122.182 |
|||
0xaeccf285 # 133.242.204.174 |
|||
0xea625b4e # 78.91.98.234 |
|||
0xbcd6031f # 31.3.214.188 |
|||
0x5e81eb18 # 24.235.129.94 |
|||
0x74b347ce # 206.71.179.116 |
|||
0x3ca56ac1 # 193.106.165.60 |
|||
0x54ee4546 # 70.69.238.84 |
|||
0x38a8175e # 94.23.168.56 |
|||
0xa3c21155 # 85.17.194.163 |
|||
0x2f01576d # 109.87.1.47 |
|||
0x5d7ade50 # 80.222.122.93 |
|||
0xa003ae48 # 72.174.3.160 |
|||
0x2bc1d31f # 31.211.193.43 |
|||
0x13f5094c # 76.9.245.19 |
|||
0x7ab32648 # 72.38.179.122 |
|||
0x542e9fd5 # 213.159.46.84 |
|||
0x53136bc1 # 193.107.19.83 |
|||
0x7fdf51c0 # 192.81.223.127 |
|||
0x802197b2 # 178.151.33.128 |
|||
0xa2d2cc5b # 91.204.210.162 |
|||
0x6b5f4bc0 # 192.75.95.107 |
|||
|
|||
# Onion nodes |
|||
bitcoinostk4e4re.onion:8333 |
|||
5k4vwyy5stro33fb.onion:8333 |
|||
zy3kdqowmrb7xm7h.onion:8333 |
|||
e3tn727fywnioxrc.onion:8333 |
|||
kjy2eqzk4zwi5zd3.onion:8333 |
|||
pt2awtcs2ulm75ig.onion:8333 |
|||
td7tgof3imei3fm6.onion:8333 |
|||
czsbwh4pq4mh3izl.onion:8333 |
|||
xdnigz4qn5dbbw2t.onion:8333 |
|||
ymnsfdejmc74vcfb.onion:7033 |
|||
jxrvw5iqizppbgml.onion:8333 |
|||
bk5ejfe56xakvtkk.onion:8333 |
|||
szsm43ou7otwwyfv.onion:8333 |
|||
5ghqw4wj6hpgfvdg.onion:8333 |
|||
evolynhit7shzeet.onion:8333 |
|||
4crhf372poejlc44.onion:8333 |
|||
tfu4kqfhsw5slqp2.onion:8333 |
|||
i2r5tbaizb75h26f.onion:8333 |
|||
btcnet3utgzyz2bf.onion:8333 |
|||
vso3r6cmjoomhhgg.onion:8333 |
|||
pqosrh6wfaucet32.onion:8333 |
|||
zy3kdqowmrb7xm7h.onion:8333 |
|||
r4de4zf4lyniu4mx.onion:8444 |
@ -0,0 +1,5 @@ |
|||
# List of fixed seed nodes for testnet |
|||
|
|||
# Onion nodes |
|||
thfsmmn2jbitcoin.onion |
|||
it2pj4f7657g3rhi.onion |
@ -0,0 +1,638 @@ |
|||
#ifndef H_CHAINPARAMSSEEDS |
|||
#define H_CHAINPARAMSSEEDS |
|||
// List of fixed seed nodes for the bitcoin network
|
|||
// AUTOGENERATED by contrib/devtools/generate-seeds.py
|
|||
|
|||
// Each line contains a 16-byte IPv6 address and a port.
|
|||
// IPv4 as well as onion addresses are wrapped inside a IPv6 address accordingly.
|
|||
static SeedSpec6 pnSeed6_main[] = { |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0x69,0x6a,0x7e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xa2,0xd1,0x04,0x7d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd9,0x17,0x0c,0x6c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb9,0x0a,0x33,0xdb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0xc7,0x49,0xc6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4d,0x48,0x95,0x78}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb0,0x09,0x71,0x04}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0xa5,0x0c,0xb9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5f,0x80,0x30,0xd1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xa6,0x4e,0x07,0xbd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0xf1,0x8f,0x57}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb0,0x09,0x6e,0x28}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x42,0xaf,0xdc,0xd4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb8,0x6b,0x9b,0x52}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0xc6,0x5c,0x63}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0x89,0xde,0xed}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc7,0xd9,0x76,0xa9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb8,0xa4,0x91,0xea}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb2,0x4e,0xfa,0x03}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x8d,0x00,0xa9,0x6c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xc8,0x62,0xaf}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x51,0xba,0xf3,0x93}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd5,0xef,0xc9,0xc2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x17,0xd5,0x0e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0x28,0x70,0x48}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xc2,0x97,0x72}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0xc6,0xf0,0x8a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x1f,0xba,0x57,0x2e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0x8a,0x09,0xd0}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x3e,0x85,0xa8,0x46}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x3e,0xdc,0x92,0xcc}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x55,0x19,0x6f,0xeb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x17,0xce,0x8c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xae,0x81,0x72,0x23}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0x79,0x42,0x9d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x09,0x4f,0x4f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd0,0x51,0x01,0xc5}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x90,0xba,0xb1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x17,0xd7,0xae}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xe5,0x4d,0x20}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x45,0x32,0xb0,0x4b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0x28,0x2b,0x93}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xe6,0xcc,0x2d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0x8a,0x70,0xe2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd5,0xb8,0x08,0x1b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xdc,0xa3,0x12}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x90,0x4c,0x88,0x8a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb8,0xa8,0x86,0xa3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x17,0xe4,0x18}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc3,0x9a,0x70,0x2e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xe9,0x62,0xeb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xae,0x03,0x65,0x6f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x81,0xd0,0x05}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x45,0xc5,0x9a,0x8a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0xd6,0x6f,0x94}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5d,0x49,0x57,0x3f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0xc6,0x29,0x4a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x90,0x4c,0x45,0xad}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x09,0x34,0x15}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0x3b,0x3f,0x4c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb9,0x19,0xfe,0x01}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0x59,0x20,0x56}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0x79,0x3c,0x44}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc8,0x4a,0xf2,0x44}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x46,0x42,0x04}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x82,0x58,0x66,0xc8}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc3,0x3e,0x3f,0xed}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5d,0xbf,0x85,0xf5}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xda,0x41,0xd1,0x5d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x08,0x3a,0xf9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0xdd,0x64,0x12}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0xc6,0x11,0x07}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbe,0x7b,0x2e,0xf1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x40,0xb7,0x02,0x5b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd5,0x6d,0x52,0x7d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x90,0x4c,0xb0,0x0c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0x11,0xbe,0x2a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0x9f,0xa3,0x61}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0x06,0x4a,0x04}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xce,0x7d,0xaf,0xf3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6d,0xc9,0x94,0xb9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0x50,0x1c,0x36}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x53,0x5d,0x73,0xca}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb0,0x43,0xa7,0xec}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x09,0x79,0xec}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x45,0x78,0xd3,0xc4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb2,0xa2,0xc4,0xa1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x53,0xd4,0x6f,0x72}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0xc6,0x5c,0x62}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0x13,0x20,0x6c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x79,0x0c,0xaa,0xb7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x3d,0x98,0xed,0xc6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0xcb,0xe4,0x47}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd4,0x75,0xac,0xa4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x45,0x93,0xe5,0xe2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd0,0x4a,0x78,0x4d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0xec,0xa5,0x18}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xc8,0x1c,0x48}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd5,0x2f,0x6c,0x7c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0x60,0x4d,0x5e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0x6c,0x4b,0x5b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x90,0x4c,0x9b,0xd9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xdc,0x87,0x39,0xe6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xbb,0x17,0xb8}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb2,0xfe,0x1c,0x14}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0x50,0x00,0x5f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0x7f,0x98,0x0d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6d,0x49,0x2a,0x24}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0x9b,0x51,0x3e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4b,0x45,0xb2,0x02}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x3d,0xaf,0xdf}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0x28,0x81,0x88}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xbb,0x65,0x11}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xca,0xfe,0xab}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x40,0x35,0xf6,0x2e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0xc7,0x73,0x57}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0xdd,0x80,0x12}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd9,0xac,0xbc,0x8e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x39,0xc4,0x81}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x45,0xfa,0xfc,0x39}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x41,0x7d,0x17,0x62}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x62,0xed,0x75,0xc9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x76,0xf4,0xcf,0x05}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x43,0xa7,0xbd,0xda}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0xc2,0x1a,0xaa}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0xa2,0x55,0xe2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0xc7,0xaa,0x88}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0xc6,0x07,0xe7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x4b,0xe9,0xa9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4b,0x48,0x93,0x28}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x27,0x51,0x99}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xca,0x70,0x39,0xd6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0x4f,0x99,0x45}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xad,0xa8,0x19,0xe5}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5d,0x5f,0xe2,0x92}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc1,0xa9,0x84,0x8b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x0a,0xaa,0x5e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xe5,0x4d,0xa7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x62,0xff,0x90,0xb0}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x32,0xee,0x5e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x79,0xa6,0x31,0xc3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0xb7,0xd9,0xc1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x82,0xb9,0x6a,0x0c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xbb,0x62,0x73}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x42,0xdd,0xfe,0x4c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0xa0,0x09,0x1e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x4c,0xc3,0xa4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc7,0xd9,0x77,0x37}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x60,0xf2,0xdc,0x5e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x48,0xb5,0xe2,0x3c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x60,0x03,0xac,0xd2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x92,0xb9,0x80,0x2f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xbb,0x4c,0x3e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xe2,0x95,0x39}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xae,0x77,0x39,0xd0}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x54,0xf0,0x3c,0x95}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd0,0x4e,0x65,0x3c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x90,0x4c,0x02,0x74}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x55,0x11,0x4f,0xa1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0x01,0xce,0x14}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6a,0xbb,0x5e,0xc1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0xc4,0x08,0x2c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0x06,0xfd,0xc7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xce,0xf8,0x04,0x76}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0x83,0xb3,0xff}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd5,0xef,0xc2,0xa4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0x40,0x61,0xe9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x57,0xe5,0x49,0xab}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0xc0,0x48,0x16}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0x40,0x02,0x36}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xad,0xff,0x8c,0x0e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x53,0x89,0x91,0x21}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xad,0x50,0x1f,0xb6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x59,0x6b,0x40,0x9b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0x22,0x28,0xaf}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x16,0x1d,0x7f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xa2,0x0d,0x56,0x1f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0xbe,0x37,0xe2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x55,0x19,0xeb,0xbd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd9,0x17,0x07,0x6c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x62,0xce,0xf8,0xda}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x24,0x74,0x0f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x43,0x52,0xe9,0xde}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x56,0x1a,0x3f,0xf2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x67,0xf8,0xbd,0x61}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x54,0xc8,0x54,0xd2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x2e,0x42,0xc4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0x63,0x05,0xae}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5f,0xa9,0xb9,0xbd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x32,0xeb,0xa9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x50,0x39,0x94,0xd9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0xdd,0x6a,0x11}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x90,0x4c,0xa5,0x73}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x52,0x6b,0xb3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x17,0x34,0xd7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x76,0x7f,0x3d,0x33}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x1b,0x43,0x51}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xcf,0xe5,0x4a,0x08}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x23,0x0a,0xa6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0x69,0x67,0x5c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x45,0xcf,0x77,0x01}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x3a,0x68,0xa6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0xea,0xf4,0x7f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb2,0xfa,0x92,0x21}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x46,0x0f,0x3a,0xa0}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xae,0x39,0x3e,0xfe}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc1,0x5f,0xce,0x2c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x48,0xc1,0xa6,0xc8}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4c,0x7e,0xfb,0x96}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0xc7,0x66,0x0a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x27,0x4d,0x6b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0xc1,0xa0,0xeb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0x79,0xa0,0x3b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x3e,0xd2,0xcc,0x1d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xa2,0xf3,0x12,0x69}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x41,0x3c,0xf2,0x22}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4a,0x6b,0x64,0x65}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x87,0x9f,0x8b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x95,0x9a,0x9b,0xeb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x6b,0xfe,0x79}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0xf4,0x36,0x05}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x61,0x4d,0x22,0x23}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x2e,0x95,0x5d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xf7,0xb4,0x0c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6d,0xbe,0x14,0xdc}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb0,0x09,0x46,0xb2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x62,0x9b,0xf7,0xd3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xc8,0x18,0x65}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x42,0xcf,0xa3,0x83}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb0,0x1f,0x64,0x9b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0xf1,0xfe,0x17}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x82,0xcc,0x08,0xd5}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x9b,0x36,0xa4,0x91}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4c,0x4a,0xcb,0x39}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x53,0xc9,0xbb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4a,0xc4,0x64,0xaf}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x50,0xca,0x5e,0x60}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x68,0x6a,0x0c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x9d,0x7e,0xd0}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xa2,0xd3,0xe6,0x78}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6d,0xeb,0x31,0x1b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb2,0xfe,0x01,0xaa}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xc2,0x03,0x46}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb6,0xa3,0xcb,0x1e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xe3,0xff,0x0e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xcb,0xfd,0xc3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6f,0x03,0x90,0xc2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0x69,0x64,0x44}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x89,0x75,0xca,0x1a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0xe5,0xa9,0x59}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x89,0x74,0xaa,0x19}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0xc8,0x22,0x26}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0xd3,0x98,0xa5}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x45,0xc3,0x8e,0x43}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb9,0x19,0x96,0xc7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x60,0x03,0x57,0xaf}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x89,0xe2,0x98,0x50}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x62,0xd8,0xad,0x36}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb2,0xa2,0xc1,0x83}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x09,0x9d,0x96}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x15,0x3d,0xcf}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x45,0xa4,0xc1,0x49}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x62,0x75,0x0b,0xbd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x55,0x19,0xff,0x8f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x53,0xfe,0x51,0x1e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd5,0xef,0xd6,0x28}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x62,0xcc,0x37,0x28}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x42,0x2d,0xf4,0x02}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb2,0x3f,0x0e,0x07}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x87,0xb1,0xbc}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xe1,0xa4,0x14}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x44,0x68,0x09,0x82}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0x1c,0xcb,0xcf}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc7,0x7f,0xe2,0x37}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x48,0x37,0x92,0x07}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0xbc,0x14,0x0c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x09,0x10,0x26}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x3e,0xd9,0x7c,0xcb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0xd2,0xc0,0x3b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4c,0x1b,0x13,0x97}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x17,0x5c,0x1e,0x6f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x43,0x9f,0x93,0xa7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0xbf,0xa0,0xb7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0x3a,0xa8,0xaf}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0x3f,0xb8,0xcb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb0,0x1c,0x32,0x5f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc7,0xc3,0xd6,0x52}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc7,0x5b,0xac,0xde}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xcc,0x10,0xf3,0x2c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc3,0x2b,0x8a,0x10}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4f,0xa1,0x6f,0x72}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xcc,0xd2,0xba,0x85}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x90,0x4c,0x9e,0x45}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd8,0xb8,0x08,0x1a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x48,0x70,0xcd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x90,0x4c,0x5b,0x6d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x7b,0xfe,0x6c,0xa6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x09,0x73,0xad}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc7,0x5b,0xac,0xda}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x9f,0xfd,0x17,0x84}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0x74,0x37,0x41}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0x86,0x13,0x1f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb2,0x12,0x5a,0x29}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb2,0x31,0xc0,0x7a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x95,0xd2,0x87,0x3a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0xbc,0x19,0xe2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x95,0xd2,0x85,0xf4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x64,0x7b,0x13}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xad,0xd9,0xff,0xcf}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x44,0x08,0xe2,0xaf}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5f,0x9a,0x67,0x32}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x44,0xc6,0x31,0xa4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x8c,0xce,0x5f,0x0e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x53,0xf8,0x5e,0x30}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0xca,0x26,0xad}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x54,0x1a,0xd2,0xd9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xd7,0xd0,0xdd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc7,0xc0,0x4e,0xc2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xcd,0xad,0x4a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0x98,0x10,0x49}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x3a,0x6b,0x9d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x8b,0x1e,0xaa,0xf0}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x43,0xa3,0xbf,0xf1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xad,0xc0,0x30,0x8a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd4,0x93,0x0f,0x26}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x60,0xe7,0x39,0x23}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x9f,0x95,0x69,0x88}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x21,0x07,0xc2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x48,0x34,0x45,0x29}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xec,0x51,0xb6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x59,0x62,0x49,0x45}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xcc,0x1b,0x3d,0xa2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x43,0xcf,0x9b,0xb3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0x94,0xd2,0xa1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0xc6,0x07,0x35}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x62,0xdd,0x88,0x4a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x63,0xf3,0xaf,0x27}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6d,0xea,0x98,0x74}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd5,0x85,0x67,0x4a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc2,0x47,0x6d,0x5e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x42,0xa5,0xab,0x3b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xae,0x37,0x5a,0x04}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc7,0xc0,0x4d,0xa2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4d,0xea,0x81,0xe9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x17,0xe2,0x6c,0xed}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x14,0x72,0x85}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x64,0x04,0x6c,0x6b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb8,0x45,0x49,0x5a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x42,0x47,0xf2,0x12}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xad,0x42,0x5f,0xf3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x5a,0x0f,0xfd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x56,0x15,0x08,0xfb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x61,0x58,0x4b,0xb2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x46,0x41,0x11,0x2e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x09,0x78,0xb7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x48,0x0e,0xbb,0x33}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x56,0x65,0xe2,0x39}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4d,0x48,0x94,0xa7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4d,0x42,0x25,0x42}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0x79,0x03,0x30}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x44,0xcf,0xc8,0x31}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x59,0xad,0x5b,0xd6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x48,0xc6,0x7b,0x12}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4c,0x4d,0xbc,0xf2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x50,0xdc,0x73,0x02}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xd7,0x72,0x45}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x53,0xf6,0x4b,0x06}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x12,0xcd,0xcd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xae,0x81,0x82,0x60}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x87,0x00,0x13,0x4d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xf7,0x16,0x10}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0x5f,0x18,0xba}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4f,0xa8,0xc1,0x16}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0x72,0x69,0xfb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x60,0x23,0x94,0xa2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x3b,0x08,0x53}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0xf1,0x83,0x05}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x41,0x24,0x5a,0x2d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x43,0xaa,0x72,0xc1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xcf,0x11,0xcd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x62,0xed,0x14,0x7b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0xf1,0x94,0x5c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0x13,0x23,0x7c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6f,0x5a,0x96,0x39}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0xe2,0x90,0x78}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0xc4,0x8e,0xa3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0xf1,0x87,0xc1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0xb6,0x80,0xef}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0x74,0x0a,0xf2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd2,0xd8,0xd1,0x7a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x2e,0x9e,0x86}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5d,0x5c,0xdb,0xcc}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xf6,0x11,0x9d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xbb,0x61,0x21}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x89,0xf8,0x99,0x75}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xad,0xec,0x65,0x22}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x55,0x0e,0x4f,0x0f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4a,0x85,0x25,0x7d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x60,0xe3,0x57,0xf8}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x3d,0x3f,0xf8}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb8,0x3b,0xc9,0x9c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x57,0x68,0x71,0x02}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x77,0xa1,0xd8,0x5d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd4,0x6c,0xdc,0x8a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x46,0x3d,0x61,0xe5}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x50,0x4f,0x73,0x6a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xae,0x3b,0x5c,0x2a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd1,0xc3,0x04,0x4a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x46,0x3d,0x61,0xe4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0xf4,0x26,0x84}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0x5f,0x1b,0x3e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0x3c,0x5a,0x0d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x54,0xd1,0xf6,0xd0}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0xff,0xc7,0x21}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x3d,0x3f,0xeb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0xed,0xa5,0x9d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x81,0x3b,0x75,0x5d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd4,0x53,0x8d,0x0d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0xf0,0x13,0x26}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x8d,0x69,0x43,0x44}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xcd,0xed,0xa1,0x8c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x3f,0xed,0x10}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x3a,0x40,0x9b,0x78}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4b,0x4a,0x98,0x7b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xbc,0x4e,0x96}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x60,0xee,0x20,0x75}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0x28,0x48,0x4f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x7d,0x40,0x5c,0x11}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x67,0x06,0xdd,0x32}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x71,0x41,0xa7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0x35,0x3f,0x1d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x56,0x1f,0x7d,0x81}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0xa5,0x99,0x2f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x56,0x59,0x2a,0x6b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x4f,0x4f,0x8d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x17,0x1e,0x3c,0xd2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x48,0x37,0x99,0x98}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xe5,0x92,0x2c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc3,0xbd,0x7e,0x23}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x43,0xfb,0x62,0xa7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x71,0x6b,0x01,0x32}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x79,0xcf,0xe7,0xd0}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd5,0xbd,0x35,0x7d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd2,0xc3,0xda,0x53}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x71,0x6b,0x01,0x31}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xce,0xf8,0xb8,0x7f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0xc2,0x38,0x9a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xad,0x42,0xaa,0xef}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x3d,0x82,0x6b,0x87}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0x53,0x17,0x18}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x7d,0x59,0x46,0xdb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xda,0x68,0xc1,0xd2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xdc,0xe9,0x6f,0xcd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x2e,0x27,0x45}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xca,0x4b,0x8d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0x3d,0x04,0xa4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0xaa,0xb7,0xaa}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xae,0x81,0x28,0x20}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x60,0xf1,0xae,0xa4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0xe6,0xd7,0xec}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xad,0x59,0x13,0x39}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0x93,0xcc,0xd8}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0xe5,0xee,0xbb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb0,0x67,0x70,0x07}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x62,0x9d,0xd3,0xeb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0xc5,0xed,0x0c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0xe1,0xd3,0x23}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0x13,0xa8,0xa5}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x32,0x9a,0x17}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x3d,0x48,0xd3,0xe4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x09,0x68,0x03}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0x8a,0x01,0xe8}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xef,0xe9,0xdd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x87,0x8b,0x43}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xa0,0x24,0x82,0xb4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0x92,0xd6,0xcb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0x79,0x75,0x90}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x3e,0x1c,0x41,0xc6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5c,0x3f,0x83,0x03}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x8b,0x3f,0xf3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4b,0x46,0x5e,0x49}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xe6,0xc8,0x83}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xcd,0x09,0xac}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x47,0xc5,0xab,0xda}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x53,0xa5,0x65,0x76}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0x37,0x26,0xc5}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xcd,0xc5,0xd0,0x2f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x61,0x4d,0x22,0x22}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x48,0x40,0x95,0x3e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x57,0x75,0xa3,0xfa}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0xc6,0xdb,0x36}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd0,0x53,0x14,0xa8}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5d,0x1f,0x94,0x5a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x60,0xea,0x98,0xa5}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x4a,0x38,0x65}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x45,0xa5,0xaa,0x10}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0x79,0xab,0xaa}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4c,0x02,0xa7,0xdd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0xf4,0x66,0x09}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x08,0x1c,0x57,0x68}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x59,0xee,0x40,0x8b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x09,0xac,0x33}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4b,0x4c,0x4b,0x84}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4d,0x25,0x92,0xd3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x46,0x5a,0x4d,0xba}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x53,0x96,0x02,0x63}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xf6,0x55,0xf6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb1,0x4b,0x4c,0xbe}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xd0,0xda,0x45}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0xc0,0x4b,0x20}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb2,0xa2,0xc3,0x06}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6a,0xba,0x1f,0xf3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb0,0x09,0x1f,0xb2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x51,0x07,0x0d,0x54}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x57,0x6a,0xb4,0xc7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0x79,0x11,0x6a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x45,0x40,0x51,0x3d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6d,0xaa,0x18,0x03}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc3,0x6e,0x30,0x30}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0x74,0x07,0x5c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6d,0xe4,0x9a,0x25}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5f,0xd3,0xbb,0x82}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0x22,0x42,0xae}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd4,0x15,0x44,0x25}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4b,0x57,0x5f,0xbd}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x17,0xfd,0xd8}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc3,0x38,0x3f,0x0a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb8,0x6b,0xce,0x2d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0xd0,0x01,0xc2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0xa5,0xfc,0x17}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xca,0x3c,0x45,0xe8}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x36,0xf6,0x61,0xd3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0xed,0xd9,0xa0}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x2e,0x23,0x2f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6c,0x11,0x4e,0x13}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x58,0xc0,0xdd,0x61}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x83,0x72,0xba,0x05}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xed,0xf7,0xe1}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0xc4,0x0e,0x04}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x2e,0x67,0x4b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6d,0xa3,0xef,0xe4}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x2e,0xa5,0xdc,0x47}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x2e,0x33,0xe9}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x92,0xb9,0xac,0xa3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x90,0x4c,0x71,0x24}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x32,0x86,0xcc,0xa8}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6d,0xce,0xb1,0x26}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd4,0x53,0x4e,0x26}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x8c,0x71,0xd2,0xd3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xad,0x34,0x55,0x22}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xa2,0xf3,0x89,0xe2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x17,0x17,0x34,0x87}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4f,0xad,0x55,0x92}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xbb,0x4b,0x18}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0x7a,0x5c,0x88}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc6,0x9a,0x6e,0x3a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x18,0x24,0x19}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x90,0x4c,0x3d,0xb7}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x7a,0x80,0x6d,0x94}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x3f,0x8e,0xd7,0xa0}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0xbb,0x16,0x5a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0x79,0x09,0xcb}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x57,0xe6,0x0d,0x8d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x25,0x8b,0x0b,0x63}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xcf,0xc6,0x72,0xe5}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0x11,0x3f,0x2b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x1f,0xa9,0x42,0x42}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x09,0x99,0x32}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x05,0x09,0x8b,0x05}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x60,0xfc,0x66,0xe2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb0,0xc5,0x66,0xbe}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6d,0xe4,0x98,0xcc}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x3e,0x94,0x8c,0x69}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc3,0x0c,0xbd,0x44}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xbc,0x7a,0x5c,0x86}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd3,0x64,0x17,0x77}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x55,0xd6,0x75,0x46}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x26,0x48,0x4e,0x35}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x52,0xc1,0x7a,0xb6}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x85,0xf2,0xcc,0xae}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4e,0x5b,0x62,0xea}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x1f,0x03,0xd6,0xbc}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x18,0xeb,0x81,0x5e}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xce,0x47,0xb3,0x74}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc1,0x6a,0xa5,0x3c}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x46,0x45,0xee,0x54}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5e,0x17,0xa8,0x38}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x55,0x11,0xc2,0xa3}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x6d,0x57,0x01,0x2f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x50,0xde,0x7a,0x5d}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x48,0xae,0x03,0xa0}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x1f,0xd3,0xc1,0x2b}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x4c,0x09,0xf5,0x13}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x48,0x26,0xb3,0x7a}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xd5,0x9f,0x2e,0x54}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc1,0x6b,0x13,0x53}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0x51,0xdf,0x7f}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xb2,0x97,0x21,0x80}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0x5b,0xcc,0xd2,0xa2}, 8333}, |
|||
{{0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xff,0xff,0xc0,0x4b,0x5f,0x6b}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x0a,0x26,0x27,0x21,0xae,0x94,0xd5,0xc2,0x72,0x24}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0xea,0xb9,0x5b,0x63,0x1d,0x94,0xe2,0xed,0xec,0xa1}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0xce,0x36,0xa1,0xc1,0xd6,0x64,0x43,0xfb,0xb3,0xe7}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x26,0xe6,0xdf,0xeb,0xe5,0xc5,0x9a,0x87,0x5e,0x22}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x52,0x71,0xa2,0x43,0x2a,0xe6,0x6c,0x8e,0xe4,0x7b}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x7c,0xf4,0x0b,0x4c,0x52,0xd5,0x16,0xcf,0xf5,0x06}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x98,0xff,0x33,0x38,0xbb,0x43,0x08,0x8d,0x95,0x9e}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x16,0x64,0x1b,0x1f,0x8f,0x87,0x18,0x7d,0xa3,0x2b}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0xb8,0xda,0x83,0x67,0x90,0x6f,0x46,0x10,0xdb,0x53}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0xc3,0x1b,0x22,0x8c,0x89,0x60,0xbf,0xca,0x88,0xa1}, 7033}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x4d,0xe3,0x5b,0x75,0x10,0x46,0x5e,0xf0,0x99,0x8b}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x0a,0xba,0x44,0x94,0x9d,0xf5,0xc0,0xaa,0xcd,0x4a}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x96,0x64,0xce,0x6d,0xd4,0xfb,0xa7,0x6b,0x60,0xb5}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0xe9,0x8f,0x0b,0x72,0xc9,0xf1,0xde,0x62,0xd4,0x66}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x25,0x5c,0xbc,0x34,0xe8,0x9f,0xe4,0x7c,0x90,0x93}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0xe0,0xa2,0x72,0xef,0xfa,0x7b,0x88,0x95,0x8b,0x9c}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x99,0x69,0xc5,0x40,0xa7,0x95,0xbb,0x25,0xc1,0xfa}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x46,0xa3,0xd9,0x84,0x08,0xc8,0x7f,0xd3,0xeb,0xc5}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x0c,0xc4,0xd2,0x4f,0x74,0x99,0xb3,0x8c,0xe8,0x25}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0xac,0x9d,0xb8,0xf8,0x4c,0x4b,0x9c,0xc3,0x9c,0xc6}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x7c,0x1d,0x28,0x9f,0xd6,0x28,0x28,0x22,0x4f,0x7a}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0xce,0x36,0xa1,0xc1,0xd6,0x64,0x43,0xfb,0xb3,0xe7}, 8333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x8f,0x06,0x4e,0x64,0xbc,0x5e,0x1a,0x8a,0x71,0x97}, 8444} |
|||
}; |
|||
|
|||
static SeedSpec6 pnSeed6_test[] = { |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x99,0xcb,0x26,0x31,0xba,0x48,0x51,0x31,0x39,0x0d}, 18333}, |
|||
{{0xfd,0x87,0xd8,0x7e,0xeb,0x43,0x44,0xf4,0xf4,0xf0,0xbf,0xf7,0x7e,0x6d,0xc4,0xe8}, 18333} |
|||
}; |
|||
#endif |
Loading…
Reference in new issue