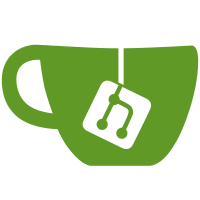
14 changed files with 9 additions and 502 deletions
@ -1,163 +0,0 @@ |
|||
// Copyright (c) 2010 Satoshi Nakamoto
|
|||
// Copyright (c) 2009-2014 The Bitcoin Core developers
|
|||
// Copyright (c) 2016-2022 The Hush developers
|
|||
// Distributed under the GPLv3 software license, see the accompanying
|
|||
// file COPYING or https://www.gnu.org/licenses/gpl-3.0.en.html
|
|||
/******************************************************************************
|
|||
* Copyright © 2014-2019 The SuperNET Developers. * |
|||
* * |
|||
* See the AUTHORS, DEVELOPER-AGREEMENT and LICENSE files at * |
|||
* the top-level directory of this distribution for the individual copyright * |
|||
* holder information and the developer policies on copyright and licensing. * |
|||
* * |
|||
* Unless otherwise agreed in a custom licensing agreement, no part of the * |
|||
* SuperNET software, including this file may be copied, modified, propagated * |
|||
* or distributed except according to the terms contained in the LICENSE file * |
|||
* * |
|||
* Removal or modification of this copyright notice is prohibited. * |
|||
* * |
|||
******************************************************************************/ |
|||
#include "alert.h" |
|||
#include "clientversion.h" |
|||
#include "net.h" |
|||
#include "pubkey.h" |
|||
#include "timedata.h" |
|||
#include "ui_interface.h" |
|||
#include "util.h" |
|||
#include <stdint.h> |
|||
#include <algorithm> |
|||
#include <map> |
|||
#include <boost/algorithm/string/classification.hpp> |
|||
#include <boost/algorithm/string/replace.hpp> |
|||
#include <boost/foreach.hpp> |
|||
#include <boost/thread.hpp> |
|||
|
|||
using namespace std; |
|||
|
|||
map<uint256, CAlert> mapAlerts; |
|||
CCriticalSection cs_mapAlerts; |
|||
|
|||
void CUnsignedAlert::SetNull() |
|||
{ |
|||
nVersion = 1; |
|||
nRelayUntil = 0; |
|||
nExpiration = 0; |
|||
nID = 0; |
|||
nCancel = 0; |
|||
setCancel.clear(); |
|||
nMinVer = 0; |
|||
nMaxVer = 0; |
|||
setSubVer.clear(); |
|||
nPriority = 0; |
|||
|
|||
strComment.clear(); |
|||
strStatusBar.clear(); |
|||
strRPCError.clear(); |
|||
} |
|||
|
|||
std::string CUnsignedAlert::ToString() const |
|||
{ |
|||
std::string strSetCancel; |
|||
BOOST_FOREACH(int n, setCancel) |
|||
strSetCancel += strprintf("%d ", n); |
|||
std::string strSetSubVer; |
|||
BOOST_FOREACH(const std::string& str, setSubVer) |
|||
strSetSubVer += "\"" + str + "\" "; |
|||
return strprintf( |
|||
"CAlert(\n" |
|||
" nVersion = %d\n" |
|||
" nRelayUntil = %d\n" |
|||
" nExpiration = %d\n" |
|||
" nID = %d\n" |
|||
" nCancel = %d\n" |
|||
" setCancel = %s\n" |
|||
" nMinVer = %d\n" |
|||
" nMaxVer = %d\n" |
|||
" setSubVer = %s\n" |
|||
" nPriority = %d\n" |
|||
" strComment = \"%s\"\n" |
|||
" strStatusBar = \"%s\"\n" |
|||
" strRPCError = \"%s\"\n" |
|||
")\n", |
|||
nVersion, |
|||
nRelayUntil, |
|||
nExpiration, |
|||
nID, |
|||
nCancel, |
|||
strSetCancel, |
|||
nMinVer, |
|||
nMaxVer, |
|||
strSetSubVer, |
|||
nPriority, |
|||
strComment, |
|||
strStatusBar, |
|||
strRPCError); |
|||
} |
|||
|
|||
void CAlert::SetNull() |
|||
{ |
|||
CUnsignedAlert::SetNull(); |
|||
vchMsg.clear(); |
|||
vchSig.clear(); |
|||
} |
|||
|
|||
bool CAlert::IsNull() const |
|||
{ |
|||
return (nExpiration == 0); |
|||
} |
|||
|
|||
uint256 CAlert::GetHash() const |
|||
{ |
|||
return Hash(this->vchMsg.begin(), this->vchMsg.end()); |
|||
} |
|||
|
|||
bool CAlert::IsInEffect() const |
|||
{ |
|||
return false; |
|||
} |
|||
|
|||
bool CAlert::Cancels(const CAlert& alert) const |
|||
{ |
|||
if (!IsInEffect()) |
|||
return false; // this was a no-op before 31403
|
|||
return (alert.nID <= nCancel || setCancel.count(alert.nID)); |
|||
} |
|||
|
|||
bool CAlert::AppliesTo(int nVersion, const std::string& strSubVerIn) const |
|||
{ |
|||
// TODO: rework for client-version-embedded-in-strSubVer ?
|
|||
return (IsInEffect() && |
|||
nMinVer <= nVersion && nVersion <= nMaxVer && |
|||
(setSubVer.empty() || setSubVer.count(strSubVerIn))); |
|||
} |
|||
|
|||
bool CAlert::AppliesToMe() const |
|||
{ |
|||
return false; |
|||
} |
|||
|
|||
bool CAlert::RelayTo(CNode* pnode) const |
|||
{ |
|||
return false; |
|||
} |
|||
|
|||
bool CAlert::CheckSignature(const std::vector<unsigned char>& alertKey) const |
|||
{ |
|||
return false; |
|||
} |
|||
|
|||
CAlert CAlert::getAlertByHash(const uint256 &hash) |
|||
{ |
|||
CAlert retval; |
|||
return retval; |
|||
} |
|||
|
|||
bool CAlert::ProcessAlert(const std::vector<unsigned char>& alertKey, bool fThread) |
|||
{ |
|||
return true; |
|||
} |
|||
|
|||
void CAlert::Notify(const std::string& strMessage, bool fThread) |
|||
{ |
|||
return; |
|||
} |
@ -1,127 +0,0 @@ |
|||
// Copyright (c) 2010 Satoshi Nakamoto
|
|||
// Copyright (c) 2009-2013 The Bitcoin Core developers
|
|||
// Copyright (c) 2016-2022 The Hush developers
|
|||
// Distributed under the GPLv3 software license, see the accompanying
|
|||
// file COPYING or https://www.gnu.org/licenses/gpl-3.0.en.html
|
|||
|
|||
/******************************************************************************
|
|||
* Copyright © 2014-2019 The SuperNET Developers. * |
|||
* * |
|||
* See the AUTHORS, DEVELOPER-AGREEMENT and LICENSE files at * |
|||
* the top-level directory of this distribution for the individual copyright * |
|||
* holder information and the developer policies on copyright and licensing. * |
|||
* * |
|||
* Unless otherwise agreed in a custom licensing agreement, no part of the * |
|||
* SuperNET software, including this file may be copied, modified, propagated * |
|||
* or distributed except according to the terms contained in the LICENSE file * |
|||
* * |
|||
* Removal or modification of this copyright notice is prohibited. * |
|||
* * |
|||
******************************************************************************/ |
|||
|
|||
#ifndef HUSH_ALERT_H |
|||
#define HUSH_ALERT_H |
|||
|
|||
#include "serialize.h" |
|||
#include "sync.h" |
|||
#include <map> |
|||
#include <set> |
|||
#include <stdint.h> |
|||
#include <string> |
|||
|
|||
class CAlert; |
|||
class CNode; |
|||
class uint256; |
|||
|
|||
extern std::map<uint256, CAlert> mapAlerts; |
|||
extern CCriticalSection cs_mapAlerts; |
|||
|
|||
/** Alerts are for notifying old versions if they become too obsolete and
|
|||
* need to upgrade. The message is displayed in the status bar. |
|||
* Alert messages are broadcast as a vector of signed data. Unserializing may |
|||
* not read the entire buffer if the alert is for a newer version, but older |
|||
* versions can still relay the original data. |
|||
*/ |
|||
class CUnsignedAlert |
|||
{ |
|||
public: |
|||
int nVersion; |
|||
int64_t nRelayUntil; // when newer nodes stop relaying to newer nodes
|
|||
int64_t nExpiration; |
|||
int nID; |
|||
int nCancel; |
|||
std::set<int> setCancel; |
|||
int nMinVer; // lowest version inclusive
|
|||
int nMaxVer; // highest version inclusive
|
|||
std::set<std::string> setSubVer; // empty matches all
|
|||
int nPriority; |
|||
|
|||
// Actions
|
|||
std::string strComment; |
|||
std::string strStatusBar; |
|||
std::string strRPCError; |
|||
|
|||
ADD_SERIALIZE_METHODS; |
|||
|
|||
template <typename Stream, typename Operation> |
|||
inline void SerializationOp(Stream& s, Operation ser_action) { |
|||
READWRITE(this->nVersion); |
|||
READWRITE(nRelayUntil); |
|||
READWRITE(nExpiration); |
|||
READWRITE(nID); |
|||
READWRITE(nCancel); |
|||
READWRITE(setCancel); |
|||
READWRITE(nMinVer); |
|||
READWRITE(nMaxVer); |
|||
READWRITE(setSubVer); |
|||
READWRITE(nPriority); |
|||
|
|||
READWRITE(LIMITED_STRING(strComment, 65536)); |
|||
READWRITE(LIMITED_STRING(strStatusBar, 256)); |
|||
READWRITE(LIMITED_STRING(strRPCError, 256)); |
|||
} |
|||
|
|||
void SetNull(); |
|||
|
|||
std::string ToString() const; |
|||
}; |
|||
|
|||
/** An alert is a combination of a serialized CUnsignedAlert and a signature. */ |
|||
class CAlert : public CUnsignedAlert |
|||
{ |
|||
public: |
|||
std::vector<unsigned char> vchMsg; |
|||
std::vector<unsigned char> vchSig; |
|||
|
|||
CAlert() |
|||
{ |
|||
SetNull(); |
|||
} |
|||
|
|||
ADD_SERIALIZE_METHODS; |
|||
|
|||
template <typename Stream, typename Operation> |
|||
inline void SerializationOp(Stream& s, Operation ser_action) { |
|||
READWRITE(vchMsg); |
|||
READWRITE(vchSig); |
|||
} |
|||
|
|||
void SetNull(); |
|||
bool IsNull() const; |
|||
uint256 GetHash() const; |
|||
bool IsInEffect() const; |
|||
bool Cancels(const CAlert& alert) const; |
|||
bool AppliesTo(int nVersion, const std::string& strSubVerIn) const; |
|||
bool AppliesToMe() const; |
|||
bool RelayTo(CNode* pnode) const; |
|||
bool CheckSignature(const std::vector<unsigned char>& alertKey) const; |
|||
bool ProcessAlert(const std::vector<unsigned char>& alertKey, bool fThread = true); // fThread means run -alertnotify in a free-running thread
|
|||
static void Notify(const std::string& strMessage, bool fThread); |
|||
|
|||
/*
|
|||
* Get copy of (active) alert object by hash. Returns a null alert if it is not found. |
|||
*/ |
|||
static CAlert getAlertByHash(const uint256 &hash); |
|||
}; |
|||
|
|||
#endif // HUSH_ALERT_H
|
@ -1,28 +0,0 @@ |
|||
// Copyright (c) 2016-2022 The Hush developers
|
|||
// Distributed under the GPLv3 software license, see the accompanying
|
|||
// file COPYING or https://www.gnu.org/licenses/gpl-3.0.en.html
|
|||
/******************************************************************************
|
|||
* Copyright © 2014-2019 The SuperNET Developers. * |
|||
* * |
|||
* See the AUTHORS, DEVELOPER-AGREEMENT and LICENSE files at * |
|||
* the top-level directory of this distribution for the individual copyright * |
|||
* holder information and the developer policies on copyright and licensing. * |
|||
* * |
|||
* Unless otherwise agreed in a custom licensing agreement, no part of the * |
|||
* SuperNET software, including this file may be copied, modified, propagated * |
|||
* or distributed except according to the terms contained in the LICENSE file * |
|||
* * |
|||
* Removal or modification of this copyright notice is prohibited. * |
|||
* * |
|||
******************************************************************************/ |
|||
|
|||
#ifndef HUSH_ALERTKEYS_H |
|||
#define HUSH_ALERTKEYS_H |
|||
|
|||
// REMINDER: DO NOT COMMIT YOUR PRIVATE KEYS TO THE GIT REPOSITORY, lulz
|
|||
|
|||
const char* pszPrivKey = "000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000"; |
|||
const char* pszTestNetPrivKey = "000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000"; |
|||
|
|||
#endif |
|||
|
@ -1,83 +0,0 @@ |
|||
// Copyright (c) 2016-2022 The Hush developers
|
|||
// Copyright (c) 2016 The Zcash developers
|
|||
// Original code from: https://gist.github.com/laanwj/0e689cfa37b52bcbbb44
|
|||
// Distributed under the GPLv3 software license, see the accompanying
|
|||
// file COPYING or https://www.gnu.org/licenses/gpl-3.0.en.html
|
|||
|
|||
/******************************************************************************
|
|||
* Copyright © 2014-2019 The SuperNET Developers. * |
|||
* * |
|||
* See the AUTHORS, DEVELOPER-AGREEMENT and LICENSE files at * |
|||
* the top-level directory of this distribution for the individual copyright * |
|||
* holder information and the developer policies on copyright and licensing. * |
|||
* * |
|||
* Unless otherwise agreed in a custom licensing agreement, no part of the * |
|||
* SuperNET software, including this file may be copied, modified, propagated * |
|||
* or distributed except according to the terms contained in the LICENSE file * |
|||
* * |
|||
* Removal or modification of this copyright notice is prohibited. * |
|||
* * |
|||
******************************************************************************/ |
|||
|
|||
/*
|
|||
|
|||
To set up a new alert system |
|||
---------------------------- |
|||
|
|||
Create a new alert key pair: |
|||
openssl ecparam -name secp256k1 -genkey -param_enc explicit -outform PEM -out data.pem |
|||
|
|||
Get the private key in hex: |
|||
openssl ec -in data.pem -outform DER | tail -c 279 | xxd -p -c 279 |
|||
|
|||
Get the public key in hex: |
|||
openssl ec -in data.pem -pubout -outform DER | tail -c 65 | xxd -p -c 65 |
|||
|
|||
Update the public keys found in chainparams.cpp. |
|||
|
|||
|
|||
To send an alert message |
|||
------------------------ |
|||
|
|||
Copy the private keys into alertkeys.h. |
|||
|
|||
Modify the alert parameters, id and message found in this file. |
|||
|
|||
Build and run with -sendalert or -printalert. |
|||
|
|||
./hushd -printtoconsole -sendalert |
|||
|
|||
One minute after starting up, the alert will be broadcast. It is then |
|||
flooded through the network until the nRelayUntil time, and will be |
|||
active until nExpiration OR the alert is cancelled. |
|||
|
|||
If you make a mistake, send another alert with nCancel set to cancel |
|||
the bad alert. |
|||
|
|||
*/ |
|||
|
|||
// Copyright (c) 2016-2022 The Hush developers
|
|||
// Distributed under the GPLv3 software license, see the accompanying
|
|||
// file COPYING or https://www.gnu.org/licenses/gpl-3.0.en.html
|
|||
#include "main.h" |
|||
#include "net.h" |
|||
#include "alert.h" |
|||
#include "init.h" |
|||
#include "util.h" |
|||
#include "utiltime.h" |
|||
#include "key.h" |
|||
#include "clientversion.h" |
|||
#include "chainparams.h" |
|||
#include "alertkeys.h" |
|||
|
|||
static const int64_t DAYS = 24 * 60 * 60; |
|||
|
|||
void ThreadSendAlert() |
|||
{ |
|||
if (!mapArgs.count("-sendalert") && !mapArgs.count("-printalert")) |
|||
return; |
|||
|
|||
fprintf(stderr,"Sending alerts not supported!\n"); |
|||
return; |
|||
|
|||
} |
Loading…
Reference in new issue