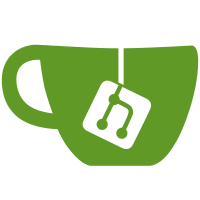
6 changed files with 4 additions and 202 deletions
@ -1,134 +0,0 @@ |
|||
// Copyright (c) 2016-2023 The Hush developers
|
|||
// Distributed under the GPLv3 software license, see the accompanying
|
|||
// file COPYING or https://www.gnu.org/licenses/gpl-3.0.en.html
|
|||
/******************************************************************************
|
|||
* Copyright © 2014-2019 The SuperNET Developers. * |
|||
* * |
|||
* See the AUTHORS, DEVELOPER-AGREEMENT and LICENSE files at * |
|||
* the top-level directory of this distribution for the individual copyright * |
|||
* holder information and the developer policies on copyright and licensing. * |
|||
* * |
|||
* Unless otherwise agreed in a custom licensing agreement, no part of the * |
|||
* SuperNET software, including this file may be copied, modified, propagated * |
|||
* or distributed except according to the terms contained in the LICENSE file * |
|||
* * |
|||
* Removal or modification of this copyright notice is prohibited. * |
|||
* * |
|||
******************************************************************************/ |
|||
|
|||
// encode decode tokens opret
|
|||
// make token cryptoconditions and vouts
|
|||
// This code was moved to a separate source file to enable linking libcommon.so (with importcoin.cpp which depends on some token functions)
|
|||
|
|||
#include "CCtokens.h" |
|||
|
|||
#ifndef IS_CHARINSTR |
|||
#define IS_CHARINSTR(c, str) (std::string(str).find((char)(c)) != std::string::npos) |
|||
#endif |
|||
|
|||
// NOTE: this inital tx won't be used by other contract
|
|||
// for tokens to be used there should be at least one 't' tx with other contract's custom opret
|
|||
CScript EncodeTokenCreateOpRet(uint8_t funcid, std::vector<uint8_t> origpubkey, std::string name, std::string description, vscript_t vopretNonfungible) |
|||
{ |
|||
return CScript(); |
|||
} |
|||
|
|||
CScript EncodeTokenCreateOpRet(uint8_t funcid, std::vector<uint8_t> origpubkey, std::string name, std::string description, std::vector<std::pair<uint8_t, vscript_t>> oprets) |
|||
{ |
|||
return CScript(); |
|||
} |
|||
|
|||
CScript EncodeTokenOpRet(uint256 tokenid, std::vector<CPubKey> voutPubkeys, std::pair<uint8_t, vscript_t> opretWithId) |
|||
{ |
|||
return CScript(); |
|||
} |
|||
|
|||
CScript EncodeTokenOpRet(uint256 tokenid, std::vector<CPubKey> voutPubkeys, std::vector<std::pair<uint8_t, vscript_t>> oprets) |
|||
{ |
|||
return CScript(); |
|||
} |
|||
|
|||
// overload for fungible tokens (no additional data in opret):
|
|||
uint8_t DecodeTokenCreateOpRet(const CScript &scriptPubKey, std::vector<uint8_t> &origpubkey, std::string &name, std::string &description) { |
|||
return 0; |
|||
} |
|||
|
|||
uint8_t DecodeTokenCreateOpRet(const CScript &scriptPubKey, std::vector<uint8_t> &origpubkey, std::string &name, std::string &description, std::vector<std::pair<uint8_t, vscript_t>> &oprets) |
|||
{ |
|||
return (uint8_t)0; |
|||
} |
|||
|
|||
// decode token opret:
|
|||
// for 't' returns all data from opret, vopretExtra contains other contract's data (currently only assets').
|
|||
// for 'c' returns only funcid. NOTE: nonfungible data is not returned
|
|||
uint8_t DecodeTokenOpRet(const CScript scriptPubKey, uint8_t &evalCodeTokens, uint256 &tokenid, std::vector<CPubKey> &voutPubkeys, std::vector<std::pair<uint8_t, vscript_t>> &oprets) |
|||
{ |
|||
return (uint8_t)0; |
|||
} |
|||
|
|||
|
|||
// make three-eval (token+evalcode+evalcode2) 1of2 cryptocondition:
|
|||
CC *MakeTokensCCcond1of2(uint8_t evalcode, uint8_t evalcode2, CPubKey pk1, CPubKey pk2) |
|||
{ |
|||
// make 1of2 sigs cond
|
|||
std::vector<CC*> pks; |
|||
pks.push_back(CCNewSecp256k1(pk1)); |
|||
pks.push_back(CCNewSecp256k1(pk2)); |
|||
|
|||
std::vector<CC*> thresholds; |
|||
thresholds.push_back(CCNewEval(E_MARSHAL(ss << evalcode))); |
|||
if (evalcode != EVAL_TOKENS) // if evalCode == EVAL_TOKENS, it is actually MakeCCcond1of2()!
|
|||
thresholds.push_back(CCNewEval(E_MARSHAL(ss << (uint8_t)EVAL_TOKENS))); // this is eval token cc
|
|||
if (evalcode2 != 0) |
|||
thresholds.push_back(CCNewEval(E_MARSHAL(ss << evalcode2))); // add optional additional evalcode
|
|||
thresholds.push_back(CCNewThreshold(1, pks)); // this is 1 of 2 sigs cc
|
|||
|
|||
return CCNewThreshold(thresholds.size(), thresholds); |
|||
} |
|||
// overload to make two-eval (token+evalcode) 1of2 cryptocondition:
|
|||
CC *MakeTokensCCcond1of2(uint8_t evalcode, CPubKey pk1, CPubKey pk2) { |
|||
return MakeTokensCCcond1of2(evalcode, 0, pk1, pk2); |
|||
} |
|||
|
|||
// make three-eval (token+evalcode+evalcode2) cryptocondition:
|
|||
CC *MakeTokensCCcond1(uint8_t evalcode, uint8_t evalcode2, CPubKey pk) |
|||
{ |
|||
std::vector<CC*> pks; |
|||
pks.push_back(CCNewSecp256k1(pk)); |
|||
|
|||
std::vector<CC*> thresholds; |
|||
thresholds.push_back(CCNewEval(E_MARSHAL(ss << evalcode))); |
|||
if (evalcode != EVAL_TOKENS) // if evalCode == EVAL_TOKENS, it is actually MakeCCcond1()!
|
|||
thresholds.push_back(CCNewEval(E_MARSHAL(ss << (uint8_t)EVAL_TOKENS))); // this is eval token cc
|
|||
if (evalcode2 != 0) |
|||
thresholds.push_back(CCNewEval(E_MARSHAL(ss << evalcode2))); // add optional additional evalcode
|
|||
thresholds.push_back(CCNewThreshold(1, pks)); // signature
|
|||
|
|||
return CCNewThreshold(thresholds.size(), thresholds); |
|||
} |
|||
// overload to make two-eval (token+evalcode) cryptocondition:
|
|||
CC *MakeTokensCCcond1(uint8_t evalcode, CPubKey pk) { |
|||
return MakeTokensCCcond1(evalcode, 0, pk); |
|||
} |
|||
|
|||
// make three-eval (token+evalcode+evalcode2) 1of2 cc vout:
|
|||
CTxOut MakeTokensCC1of2vout(uint8_t evalcode, uint8_t evalcode2, CAmount nValue, CPubKey pk1, CPubKey pk2) |
|||
{ |
|||
CTxOut vout; |
|||
return(vout); |
|||
} |
|||
// overload to make two-eval (token+evalcode) 1of2 cc vout:
|
|||
CTxOut MakeTokensCC1of2vout(uint8_t evalcode, CAmount nValue, CPubKey pk1, CPubKey pk2) { |
|||
return CTxOut(); |
|||
} |
|||
|
|||
// make three-eval (token+evalcode+evalcode2) cc vout:
|
|||
CTxOut MakeTokensCC1vout(uint8_t evalcode, uint8_t evalcode2, CAmount nValue, CPubKey pk) |
|||
{ |
|||
return CTxOut(); |
|||
} |
|||
// overload to make two-eval (token+evalcode) cc vout:
|
|||
CTxOut MakeTokensCC1vout(uint8_t evalcode, CAmount nValue, CPubKey pk) { |
|||
return CTxOut(); |
|||
} |
|||
|
Loading…
Reference in new issue