Browse Source
- remove btc address length from address validator - add an optional btc address check in validated line edit that defaults to off and is used in GUIUtil::setupAddressWidget() - an isAcceptable() check is added to validated line edit on focus out which only kicks in, when a validator is used with that widget - remove an isAcceptable() check from sendcoinsentry.cpp - remove obsolete attributes from ui files, which are set by calling GUIUtil::setupAddressWidget() - move some more things to GUIUtil::setupAddressWidget() and remove them from normal code e.g. placeholder textpull/145/head
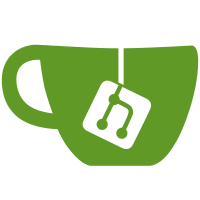
committed by
Wladimir J. van der Laan
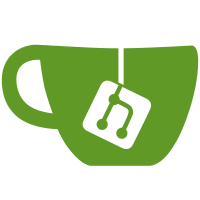
11 changed files with 140 additions and 55 deletions
Loading…
Reference in new issue