Browse Source
class template base_uint had its own private lookup table. This is saving 256 bytes per instantiation. The result is not spectacular as bitcoin-qt has only shrinked of about 1Kb but it is still valid improvement. Also, I have replaced a for loop with a memset() call. Made CBigNum::SetHex() use the new HexDigit() function. Signed-off-by: Olivier Langlois <olivier@olivierlanglois.net>pull/145/head
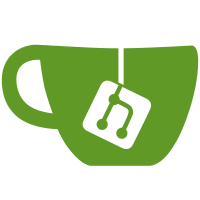
5 changed files with 34 additions and 14 deletions
Loading…
Reference in new issue