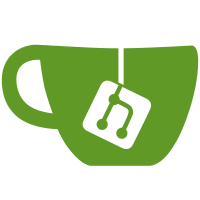
10 changed files with 335 additions and 17 deletions
@ -0,0 +1,136 @@ |
|||
/* XMRig
|
|||
* Copyright 2010 Jeff Garzik <jgarzik@pobox.com> |
|||
* Copyright 2012-2014 pooler <pooler@litecoinpool.org> |
|||
* Copyright 2014 Lucas Jones <https://github.com/lucasjones>
|
|||
* Copyright 2014-2016 Wolf9466 <https://github.com/OhGodAPet>
|
|||
* Copyright 2016 Jay D Dee <jayddee246@gmail.com> |
|||
* Copyright 2017-2018 XMR-Stak <https://github.com/fireice-uk>, <https://github.com/psychocrypt>
|
|||
* Copyright 2018-2019 SChernykh <https://github.com/SChernykh>
|
|||
* Copyright 2016-2019 XMRig <https://github.com/xmrig>, <support@xmrig.com>
|
|||
* |
|||
* This program is free software: you can redistribute it and/or modify |
|||
* it under the terms of the GNU General Public License as published by |
|||
* the Free Software Foundation, either version 3 of the License, or |
|||
* (at your option) any later version. |
|||
* |
|||
* This program is distributed in the hope that it will be useful, |
|||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|||
* GNU General Public License for more details. |
|||
* |
|||
* You should have received a copy of the GNU General Public License |
|||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
*/ |
|||
|
|||
|
|||
#include <stdexcept> |
|||
#include <uv.h> |
|||
|
|||
|
|||
#include "backend/cuda/wrappers/NvmlLib.h" |
|||
#include "backend/cuda/wrappers/nvml_lite.h" |
|||
#include "base/io/log/Log.h" |
|||
|
|||
|
|||
|
|||
namespace xmrig { |
|||
|
|||
|
|||
static uv_lib_t nvmlLib; |
|||
|
|||
|
|||
static const char *kNvmlInit = "nvmlInit_v2"; |
|||
static const char *kNvmlShutdown = "nvmlShutdown"; |
|||
static const char *kNvmlSystemGetDriverVersion = "nvmlSystemGetDriverVersion"; |
|||
static const char *kNvmlSystemGetNVMLVersion = "nvmlSystemGetNVMLVersion"; |
|||
static const char *kSymbolNotFound = "symbol not found"; |
|||
|
|||
|
|||
static nvmlReturn_t (*pNvmlInit)() = nullptr; |
|||
static nvmlReturn_t (*pNvmlShutdown)() = nullptr; |
|||
static nvmlReturn_t (*pNvmlSystemGetDriverVersion)(char *version, unsigned int length) = nullptr; |
|||
static nvmlReturn_t (*pNvmlSystemGetNVMLVersion)(char *version, unsigned int length) = nullptr; |
|||
|
|||
|
|||
#define DLSYM(x) if (uv_dlsym(&nvmlLib, k##x, reinterpret_cast<void**>(&p##x)) == -1) { throw std::runtime_error(kSymbolNotFound); } |
|||
|
|||
|
|||
bool NvmlLib::m_initialized = false; |
|||
bool NvmlLib::m_ready = false; |
|||
char NvmlLib::m_driverVersion[80] = { 0 }; |
|||
char NvmlLib::m_nvmlVersion[80] = { 0 }; |
|||
String NvmlLib::m_loader; |
|||
|
|||
|
|||
} // namespace xmrig
|
|||
|
|||
|
|||
bool xmrig::NvmlLib::init(const char *fileName) |
|||
{ |
|||
if (!m_initialized) { |
|||
m_loader = fileName; |
|||
m_ready = dlopen() && load(); |
|||
m_initialized = true; |
|||
} |
|||
|
|||
return m_ready; |
|||
} |
|||
|
|||
|
|||
const char *xmrig::NvmlLib::lastError() noexcept |
|||
{ |
|||
return uv_dlerror(&nvmlLib); |
|||
} |
|||
|
|||
|
|||
void xmrig::NvmlLib::close() |
|||
{ |
|||
if (m_ready) { |
|||
pNvmlShutdown(); |
|||
} |
|||
|
|||
uv_dlclose(&nvmlLib); |
|||
} |
|||
|
|||
|
|||
bool xmrig::NvmlLib::dlopen() |
|||
{ |
|||
if (!m_loader.isNull()) { |
|||
return uv_dlopen(m_loader, &nvmlLib) == 0; |
|||
} |
|||
|
|||
# ifdef _WIN32 |
|||
if (uv_dlopen("nvml.dll", &nvmlLib) == 0) { |
|||
return true; |
|||
} |
|||
|
|||
char path[MAX_PATH] = { 0 }; |
|||
ExpandEnvironmentStringsA("%PROGRAMFILES%\\NVIDIA Corporation\\NVSMI\\nvml.dll", path, sizeof(path)); |
|||
|
|||
return uv_dlopen(path, &nvmlLib) == 0; |
|||
# else |
|||
return uv_dlopen("libnvidia-ml.so", &nvmlLib) == 0; |
|||
# endif |
|||
} |
|||
|
|||
|
|||
bool xmrig::NvmlLib::load() |
|||
{ |
|||
try { |
|||
DLSYM(NvmlInit); |
|||
DLSYM(NvmlShutdown); |
|||
DLSYM(NvmlSystemGetDriverVersion); |
|||
DLSYM(NvmlSystemGetNVMLVersion); |
|||
} catch (std::exception &ex) { |
|||
return false; |
|||
} |
|||
|
|||
if (pNvmlInit() != NVML_SUCCESS) { |
|||
return false; |
|||
} |
|||
|
|||
pNvmlSystemGetDriverVersion(m_driverVersion, sizeof(m_driverVersion)); |
|||
pNvmlSystemGetNVMLVersion(m_nvmlVersion, sizeof(m_nvmlVersion)); |
|||
|
|||
return true; |
|||
} |
@ -0,0 +1,62 @@ |
|||
/* XMRig
|
|||
* Copyright 2010 Jeff Garzik <jgarzik@pobox.com> |
|||
* Copyright 2012-2014 pooler <pooler@litecoinpool.org> |
|||
* Copyright 2014 Lucas Jones <https://github.com/lucasjones>
|
|||
* Copyright 2014-2016 Wolf9466 <https://github.com/OhGodAPet>
|
|||
* Copyright 2016 Jay D Dee <jayddee246@gmail.com> |
|||
* Copyright 2017-2018 XMR-Stak <https://github.com/fireice-uk>, <https://github.com/psychocrypt>
|
|||
* Copyright 2018-2019 SChernykh <https://github.com/SChernykh>
|
|||
* Copyright 2016-2019 XMRig <https://github.com/xmrig>, <support@xmrig.com>
|
|||
* |
|||
* This program is free software: you can redistribute it and/or modify |
|||
* it under the terms of the GNU General Public License as published by |
|||
* the Free Software Foundation, either version 3 of the License, or |
|||
* (at your option) any later version. |
|||
* |
|||
* This program is distributed in the hope that it will be useful, |
|||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|||
* GNU General Public License for more details. |
|||
* |
|||
* You should have received a copy of the GNU General Public License |
|||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
*/ |
|||
|
|||
#ifndef XMRIG_NVMLLIB_H |
|||
#define XMRIG_NVMLLIB_H |
|||
|
|||
|
|||
#include "base/tools/String.h" |
|||
|
|||
|
|||
namespace xmrig { |
|||
|
|||
|
|||
class NvmlLib |
|||
{ |
|||
public: |
|||
static bool init(const char *fileName = nullptr); |
|||
static const char *lastError() noexcept; |
|||
static void close(); |
|||
|
|||
static inline bool isInitialized() noexcept { return m_initialized; } |
|||
static inline bool isReady() noexcept { return m_ready; } |
|||
static inline const char *driverVersion() noexcept { return m_driverVersion; } |
|||
static inline const char *version() noexcept { return m_nvmlVersion; } |
|||
|
|||
private: |
|||
static bool dlopen(); |
|||
static bool load(); |
|||
|
|||
static bool m_initialized; |
|||
static bool m_ready; |
|||
static char m_driverVersion[80]; |
|||
static char m_nvmlVersion[80]; |
|||
static String m_loader; |
|||
}; |
|||
|
|||
|
|||
} // namespace xmrig
|
|||
|
|||
|
|||
#endif /* XMRIG_NVMLLIB_H */ |
@ -0,0 +1,38 @@ |
|||
/* XMRig
|
|||
* Copyright 2010 Jeff Garzik <jgarzik@pobox.com> |
|||
* Copyright 2012-2014 pooler <pooler@litecoinpool.org> |
|||
* Copyright 2014 Lucas Jones <https://github.com/lucasjones>
|
|||
* Copyright 2014-2016 Wolf9466 <https://github.com/OhGodAPet>
|
|||
* Copyright 2016 Jay D Dee <jayddee246@gmail.com> |
|||
* Copyright 2017-2018 XMR-Stak <https://github.com/fireice-uk>, <https://github.com/psychocrypt>
|
|||
* Copyright 2018-2019 SChernykh <https://github.com/SChernykh>
|
|||
* Copyright 2016-2019 XMRig <https://github.com/xmrig>, <support@xmrig.com>
|
|||
* |
|||
* This program is free software: you can redistribute it and/or modify |
|||
* it under the terms of the GNU General Public License as published by |
|||
* the Free Software Foundation, either version 3 of the License, or |
|||
* (at your option) any later version. |
|||
* |
|||
* This program is distributed in the hope that it will be useful, |
|||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|||
* GNU General Public License for more details. |
|||
* |
|||
* You should have received a copy of the GNU General Public License |
|||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
*/ |
|||
|
|||
#ifndef XMRIG_NVML_LITE_H |
|||
#define XMRIG_NVML_LITE_H |
|||
|
|||
|
|||
#include <cstdint> |
|||
|
|||
|
|||
#define NVML_SUCCESS 0 |
|||
|
|||
|
|||
using nvmlReturn_t = uint32_t; |
|||
|
|||
|
|||
#endif /* XMRIG_NVML_LITE_H */ |
Loading…
Reference in new issue