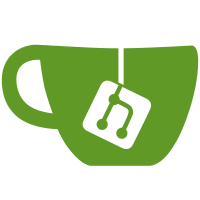
14 changed files with 565 additions and 6 deletions
@ -0,0 +1,53 @@ |
|||
/* XMRig
|
|||
* Copyright 2010 Jeff Garzik <jgarzik@pobox.com> |
|||
* Copyright 2012-2014 pooler <pooler@litecoinpool.org> |
|||
* Copyright 2014 Lucas Jones <https://github.com/lucasjones>
|
|||
* Copyright 2014-2016 Wolf9466 <https://github.com/OhGodAPet>
|
|||
* Copyright 2016 Jay D Dee <jayddee246@gmail.com> |
|||
* Copyright 2017-2018 XMR-Stak <https://github.com/fireice-uk>, <https://github.com/psychocrypt>
|
|||
* Copyright 2018-2019 SChernykh <https://github.com/SChernykh>
|
|||
* Copyright 2016-2019 XMRig <https://github.com/xmrig>, <support@xmrig.com>
|
|||
* |
|||
* This program is free software: you can redistribute it and/or modify |
|||
* it under the terms of the GNU General Public License as published by |
|||
* the Free Software Foundation, either version 3 of the License, or |
|||
* (at your option) any later version. |
|||
* |
|||
* This program is distributed in the hope that it will be useful, |
|||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|||
* GNU General Public License for more details. |
|||
* |
|||
* You should have received a copy of the GNU General Public License |
|||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
*/ |
|||
|
|||
#ifndef XMRIG_IOCLRUNNER_H |
|||
#define XMRIG_IOCLRUNNER_H |
|||
|
|||
|
|||
#include <stdint.h> |
|||
|
|||
|
|||
namespace xmrig { |
|||
|
|||
|
|||
class Job; |
|||
|
|||
|
|||
class IOclRunner |
|||
{ |
|||
public: |
|||
virtual ~IOclRunner() = default; |
|||
|
|||
virtual bool selfTest() const = 0; |
|||
virtual const char *buildOptions() const = 0; |
|||
virtual void run(uint32_t *hashOutput) = 0; |
|||
virtual void set(const Job &job) = 0; |
|||
}; |
|||
|
|||
|
|||
} /* namespace xmrig */ |
|||
|
|||
|
|||
#endif // XMRIG_IOCLRUNNER_H
|
@ -0,0 +1,78 @@ |
|||
/* XMRig
|
|||
* Copyright 2010 Jeff Garzik <jgarzik@pobox.com> |
|||
* Copyright 2012-2014 pooler <pooler@litecoinpool.org> |
|||
* Copyright 2014 Lucas Jones <https://github.com/lucasjones>
|
|||
* Copyright 2014-2016 Wolf9466 <https://github.com/OhGodAPet>
|
|||
* Copyright 2016 Jay D Dee <jayddee246@gmail.com> |
|||
* Copyright 2017-2018 XMR-Stak <https://github.com/fireice-uk>, <https://github.com/psychocrypt>
|
|||
* Copyright 2018-2019 SChernykh <https://github.com/SChernykh>
|
|||
* Copyright 2016-2019 XMRig <https://github.com/xmrig>, <support@xmrig.com>
|
|||
* |
|||
* This program is free software: you can redistribute it and/or modify |
|||
* it under the terms of the GNU General Public License as published by |
|||
* the Free Software Foundation, either version 3 of the License, or |
|||
* (at your option) any later version. |
|||
* |
|||
* This program is distributed in the hope that it will be useful, |
|||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|||
* GNU General Public License for more details. |
|||
* |
|||
* You should have received a copy of the GNU General Public License |
|||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
*/ |
|||
|
|||
|
|||
#include "backend/opencl/OclLaunchData.h" |
|||
#include "backend/opencl/runners/OclBaseRunner.h" |
|||
#include "backend/opencl/wrappers/OclLib.h" |
|||
#include "base/net/stratum/Job.h" |
|||
|
|||
|
|||
xmrig::OclBaseRunner::OclBaseRunner(size_t, const OclLaunchData &data) : |
|||
m_algorithm(data.algorithm), |
|||
m_ctx(data.ctx) |
|||
{ |
|||
cl_int ret; |
|||
m_queue = OclLib::createCommandQueue(m_ctx, data.device.id(), &ret); |
|||
if (ret != CL_SUCCESS) { |
|||
return; |
|||
} |
|||
|
|||
m_input = OclLib::createBuffer(m_ctx, CL_MEM_READ_ONLY, Job::kMaxBlobSize, nullptr, &ret); |
|||
m_output = OclLib::createBuffer(m_ctx, CL_MEM_READ_WRITE, sizeof(cl_uint) * 0x100, nullptr, &ret); |
|||
} |
|||
|
|||
|
|||
xmrig::OclBaseRunner::~OclBaseRunner() |
|||
{ |
|||
OclLib::releaseMemObject(m_input); |
|||
OclLib::releaseMemObject(m_output); |
|||
|
|||
OclLib::releaseCommandQueue(m_queue); |
|||
} |
|||
|
|||
|
|||
bool xmrig::OclBaseRunner::selfTest() const |
|||
{ |
|||
return m_queue != nullptr && m_input != nullptr && m_output != nullptr && !m_options.empty(); |
|||
} |
|||
|
|||
|
|||
|
|||
const char *xmrig::OclBaseRunner::buildOptions() const |
|||
{ |
|||
return m_options.c_str(); |
|||
} |
|||
|
|||
|
|||
void xmrig::OclBaseRunner::run(uint32_t *hashOutput) |
|||
{ |
|||
|
|||
} |
|||
|
|||
|
|||
void xmrig::OclBaseRunner::set(const Job &job) |
|||
{ |
|||
|
|||
} |
@ -0,0 +1,68 @@ |
|||
/* XMRig
|
|||
* Copyright 2010 Jeff Garzik <jgarzik@pobox.com> |
|||
* Copyright 2012-2014 pooler <pooler@litecoinpool.org> |
|||
* Copyright 2014 Lucas Jones <https://github.com/lucasjones>
|
|||
* Copyright 2014-2016 Wolf9466 <https://github.com/OhGodAPet>
|
|||
* Copyright 2016 Jay D Dee <jayddee246@gmail.com> |
|||
* Copyright 2017-2018 XMR-Stak <https://github.com/fireice-uk>, <https://github.com/psychocrypt>
|
|||
* Copyright 2018-2019 SChernykh <https://github.com/SChernykh>
|
|||
* Copyright 2016-2019 XMRig <https://github.com/xmrig>, <support@xmrig.com>
|
|||
* |
|||
* This program is free software: you can redistribute it and/or modify |
|||
* it under the terms of the GNU General Public License as published by |
|||
* the Free Software Foundation, either version 3 of the License, or |
|||
* (at your option) any later version. |
|||
* |
|||
* This program is distributed in the hope that it will be useful, |
|||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|||
* GNU General Public License for more details. |
|||
* |
|||
* You should have received a copy of the GNU General Public License |
|||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
*/ |
|||
|
|||
#ifndef XMRIG_OCLBASERUNNER_H |
|||
#define XMRIG_OCLBASERUNNER_H |
|||
|
|||
|
|||
#include <string> |
|||
|
|||
|
|||
#include "3rdparty/cl.h" |
|||
#include "backend/opencl/interfaces/IOclRunner.h" |
|||
#include "crypto/common/Algorithm.h" |
|||
|
|||
|
|||
namespace xmrig { |
|||
|
|||
|
|||
class OclLaunchData; |
|||
|
|||
|
|||
class OclBaseRunner : public IOclRunner |
|||
{ |
|||
public: |
|||
OclBaseRunner(size_t index, const OclLaunchData &data); |
|||
~OclBaseRunner() override; |
|||
|
|||
protected: |
|||
bool selfTest() const override; |
|||
const char *buildOptions() const override; |
|||
void run(uint32_t *hashOutput) override; |
|||
void set(const Job &job) override; |
|||
|
|||
protected: |
|||
Algorithm m_algorithm; |
|||
cl_command_queue m_queue = nullptr; |
|||
cl_context m_ctx; |
|||
cl_mem m_input = nullptr; |
|||
cl_mem m_output = nullptr; |
|||
std::string m_options; |
|||
}; |
|||
|
|||
|
|||
} /* namespace xmrig */ |
|||
|
|||
|
|||
#endif // XMRIG_OCLBASERUNNER_H
|
@ -0,0 +1,99 @@ |
|||
/* XMRig
|
|||
* Copyright 2010 Jeff Garzik <jgarzik@pobox.com> |
|||
* Copyright 2012-2014 pooler <pooler@litecoinpool.org> |
|||
* Copyright 2014 Lucas Jones <https://github.com/lucasjones>
|
|||
* Copyright 2014-2016 Wolf9466 <https://github.com/OhGodAPet>
|
|||
* Copyright 2016 Jay D Dee <jayddee246@gmail.com> |
|||
* Copyright 2017-2018 XMR-Stak <https://github.com/fireice-uk>, <https://github.com/psychocrypt>
|
|||
* Copyright 2018-2019 SChernykh <https://github.com/SChernykh>
|
|||
* Copyright 2016-2019 XMRig <https://github.com/xmrig>, <support@xmrig.com>
|
|||
* |
|||
* This program is free software: you can redistribute it and/or modify |
|||
* it under the terms of the GNU General Public License as published by |
|||
* the Free Software Foundation, either version 3 of the License, or |
|||
* (at your option) any later version. |
|||
* |
|||
* This program is distributed in the hope that it will be useful, |
|||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|||
* GNU General Public License for more details. |
|||
* |
|||
* You should have received a copy of the GNU General Public License |
|||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
*/ |
|||
|
|||
|
|||
#include "backend/opencl/runners/OclCnRunner.h" |
|||
#include "backend/opencl/wrappers/OclLib.h" |
|||
#include "backend/opencl/OclLaunchData.h" |
|||
#include "crypto/cn/CnAlgo.h" |
|||
|
|||
|
|||
xmrig::OclCnRunner::OclCnRunner(size_t index, const OclLaunchData &data) : OclBaseRunner(index, data) |
|||
{ |
|||
if (m_queue == nullptr) { |
|||
return; |
|||
} |
|||
|
|||
const size_t g_thd = data.thread.intensity(); |
|||
|
|||
cl_int ret; |
|||
m_scratchpads = OclLib::createBuffer(m_ctx, CL_MEM_READ_WRITE, data.algorithm.l3() * g_thd, nullptr, &ret); |
|||
if (ret != CL_SUCCESS) { |
|||
return; |
|||
} |
|||
|
|||
m_states = OclLib::createBuffer(m_ctx, CL_MEM_READ_WRITE, 200 * g_thd, nullptr, &ret); |
|||
m_blake256 = OclLib::createBuffer(m_ctx, CL_MEM_READ_WRITE, sizeof(cl_uint) * (g_thd + 2), nullptr, &ret); |
|||
m_groestl256 = OclLib::createBuffer(m_ctx, CL_MEM_READ_WRITE, sizeof(cl_uint) * (g_thd + 2), nullptr, &ret); |
|||
m_jh256 = OclLib::createBuffer(m_ctx, CL_MEM_READ_WRITE, sizeof(cl_uint) * (g_thd + 2), nullptr, &ret); |
|||
m_skein512 = OclLib::createBuffer(m_ctx, CL_MEM_READ_WRITE, sizeof(cl_uint) * (g_thd + 2), nullptr, &ret); |
|||
|
|||
uint32_t stridedIndex = data.thread.stridedIndex(); |
|||
if (data.device.vendorId() == OCL_VENDOR_NVIDIA) { |
|||
stridedIndex = 0; |
|||
} |
|||
else if (stridedIndex == 1 && (m_algorithm.family() == Algorithm::CN_PICO || (m_algorithm.family() == Algorithm::CN && CnAlgo<>::base(m_algorithm) == Algorithm::CN_2))) { |
|||
stridedIndex = 2; |
|||
} |
|||
|
|||
m_options += " -DITERATIONS=" + std::to_string(CnAlgo<>::iterations(m_algorithm)) + "U"; |
|||
m_options += " -DMASK=" + std::to_string(CnAlgo<>::mask(m_algorithm)) + "U"; |
|||
m_options += " -DWORKSIZE=" + std::to_string(data.thread.worksize()) + "U"; |
|||
m_options += " -DSTRIDED_INDEX=" + std::to_string(stridedIndex) + "U"; |
|||
m_options += " -DMEM_CHUNK_EXPONENT=" + std::to_string(1u << data.thread.memChunk()) + "U"; |
|||
m_options += " -DCOMP_MODE=" + std::to_string(data.thread.isCompMode() && g_thd % data.thread.worksize() != 0 ? 1u : 0u) + "U"; |
|||
m_options += " -DMEMORY=" + std::to_string(m_algorithm.l3()) + "LU"; |
|||
m_options += " -DALGO=" + std::to_string(m_algorithm.id()); |
|||
m_options += " -DALGO_FAMILY=" + std::to_string(m_algorithm.family()); |
|||
m_options += " -DCN_UNROLL=" + std::to_string(data.thread.unrollFactor()); |
|||
|
|||
# ifdef XMRIG_ALGO_CN_GPU |
|||
if (data.algorithm == Algorithm::CN_GPU) { |
|||
m_options += " -cl-fp32-correctly-rounded-divide-sqrt"; |
|||
} |
|||
# endif |
|||
} |
|||
|
|||
|
|||
xmrig::OclCnRunner::~OclCnRunner() |
|||
{ |
|||
OclLib::releaseMemObject(m_scratchpads); |
|||
OclLib::releaseMemObject(m_states); |
|||
OclLib::releaseMemObject(m_blake256); |
|||
OclLib::releaseMemObject(m_groestl256); |
|||
OclLib::releaseMemObject(m_jh256); |
|||
OclLib::releaseMemObject(m_skein512); |
|||
} |
|||
|
|||
|
|||
bool xmrig::OclCnRunner::selfTest() const |
|||
{ |
|||
return OclBaseRunner::selfTest() && |
|||
m_scratchpads != nullptr && |
|||
m_states != nullptr && |
|||
m_blake256 != nullptr && |
|||
m_groestl256 != nullptr && |
|||
m_jh256 != nullptr && |
|||
m_skein512 != nullptr; |
|||
} |
@ -0,0 +1,57 @@ |
|||
/* XMRig
|
|||
* Copyright 2010 Jeff Garzik <jgarzik@pobox.com> |
|||
* Copyright 2012-2014 pooler <pooler@litecoinpool.org> |
|||
* Copyright 2014 Lucas Jones <https://github.com/lucasjones>
|
|||
* Copyright 2014-2016 Wolf9466 <https://github.com/OhGodAPet>
|
|||
* Copyright 2016 Jay D Dee <jayddee246@gmail.com> |
|||
* Copyright 2017-2018 XMR-Stak <https://github.com/fireice-uk>, <https://github.com/psychocrypt>
|
|||
* Copyright 2018-2019 SChernykh <https://github.com/SChernykh>
|
|||
* Copyright 2016-2019 XMRig <https://github.com/xmrig>, <support@xmrig.com>
|
|||
* |
|||
* This program is free software: you can redistribute it and/or modify |
|||
* it under the terms of the GNU General Public License as published by |
|||
* the Free Software Foundation, either version 3 of the License, or |
|||
* (at your option) any later version. |
|||
* |
|||
* This program is distributed in the hope that it will be useful, |
|||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|||
* GNU General Public License for more details. |
|||
* |
|||
* You should have received a copy of the GNU General Public License |
|||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
*/ |
|||
|
|||
#ifndef XMRIG_OCLCNRUNNER_H |
|||
#define XMRIG_OCLCNRUNNER_H |
|||
|
|||
|
|||
#include "backend/opencl/runners/OclBaseRunner.h" |
|||
|
|||
|
|||
namespace xmrig { |
|||
|
|||
|
|||
class OclCnRunner : public OclBaseRunner |
|||
{ |
|||
public: |
|||
OclCnRunner(size_t index, const OclLaunchData &data); |
|||
~OclCnRunner() override; |
|||
|
|||
protected: |
|||
bool selfTest() const override; |
|||
|
|||
private: |
|||
cl_mem m_blake256 = nullptr; |
|||
cl_mem m_groestl256 = nullptr; |
|||
cl_mem m_jh256 = nullptr; |
|||
cl_mem m_scratchpads = nullptr; |
|||
cl_mem m_skein512 = nullptr; |
|||
cl_mem m_states = nullptr; |
|||
}; |
|||
|
|||
|
|||
} /* namespace xmrig */ |
|||
|
|||
|
|||
#endif // XMRIG_OCLCNRUNNER_H
|
@ -0,0 +1,36 @@ |
|||
/* XMRig
|
|||
* Copyright 2010 Jeff Garzik <jgarzik@pobox.com> |
|||
* Copyright 2012-2014 pooler <pooler@litecoinpool.org> |
|||
* Copyright 2014 Lucas Jones <https://github.com/lucasjones>
|
|||
* Copyright 2014-2016 Wolf9466 <https://github.com/OhGodAPet>
|
|||
* Copyright 2016 Jay D Dee <jayddee246@gmail.com> |
|||
* Copyright 2017-2018 XMR-Stak <https://github.com/fireice-uk>, <https://github.com/psychocrypt>
|
|||
* Copyright 2018-2019 SChernykh <https://github.com/SChernykh>
|
|||
* Copyright 2016-2019 XMRig <https://github.com/xmrig>, <support@xmrig.com>
|
|||
* |
|||
* This program is free software: you can redistribute it and/or modify |
|||
* it under the terms of the GNU General Public License as published by |
|||
* the Free Software Foundation, either version 3 of the License, or |
|||
* (at your option) any later version. |
|||
* |
|||
* This program is distributed in the hope that it will be useful, |
|||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|||
* GNU General Public License for more details. |
|||
* |
|||
* You should have received a copy of the GNU General Public License |
|||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
*/ |
|||
|
|||
#include "backend/opencl/runners/OclRxRunner.h" |
|||
|
|||
|
|||
xmrig::OclRxRunner::OclRxRunner(size_t index, const OclLaunchData &data) : OclBaseRunner(index, data) |
|||
{ |
|||
} |
|||
|
|||
|
|||
bool xmrig::OclRxRunner::selfTest() const |
|||
{ |
|||
return false; // TODO
|
|||
} |
@ -0,0 +1,48 @@ |
|||
/* XMRig
|
|||
* Copyright 2010 Jeff Garzik <jgarzik@pobox.com> |
|||
* Copyright 2012-2014 pooler <pooler@litecoinpool.org> |
|||
* Copyright 2014 Lucas Jones <https://github.com/lucasjones>
|
|||
* Copyright 2014-2016 Wolf9466 <https://github.com/OhGodAPet>
|
|||
* Copyright 2016 Jay D Dee <jayddee246@gmail.com> |
|||
* Copyright 2017-2018 XMR-Stak <https://github.com/fireice-uk>, <https://github.com/psychocrypt>
|
|||
* Copyright 2018-2019 SChernykh <https://github.com/SChernykh>
|
|||
* Copyright 2016-2019 XMRig <https://github.com/xmrig>, <support@xmrig.com>
|
|||
* |
|||
* This program is free software: you can redistribute it and/or modify |
|||
* it under the terms of the GNU General Public License as published by |
|||
* the Free Software Foundation, either version 3 of the License, or |
|||
* (at your option) any later version. |
|||
* |
|||
* This program is distributed in the hope that it will be useful, |
|||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|||
* GNU General Public License for more details. |
|||
* |
|||
* You should have received a copy of the GNU General Public License |
|||
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|||
*/ |
|||
|
|||
#ifndef XMRIG_OCLRXRUNNER_H |
|||
#define XMRIG_OCLRXRUNNER_H |
|||
|
|||
|
|||
#include "backend/opencl/runners/OclBaseRunner.h" |
|||
|
|||
|
|||
namespace xmrig { |
|||
|
|||
|
|||
class OclRxRunner : public OclBaseRunner |
|||
{ |
|||
public: |
|||
OclRxRunner(size_t index, const OclLaunchData &data); |
|||
|
|||
protected: |
|||
bool selfTest() const override; |
|||
}; |
|||
|
|||
|
|||
} /* namespace xmrig */ |
|||
|
|||
|
|||
#endif // XMRIG_OCLRXRUNNER_H
|
Loading…
Reference in new issue