forked from hush/hush3
Browse Source
Refactor keytime: * Key metadata is kept in a CWallet::mapKeyMetadata (std::map<CKeyId,CKeyMetadata>). * When generating a new key, time is put in that map, and new key is written. * AddKeyPubKey and AddCryptedKey do not take a creation time argument, but instead pull it from that map, if it exists there. Bugfix: * AddKeyPubKey and AddCryptedKey in CWallet didn't override the CKeyStore definition anymore. This is fixed, as they no longed need the nCreationTime argument now. Also a few related other changes: * Metadata can be overwritten. * Only GenerateNewKey calls GetTime(), as it's the only place where we know for sure a key was not constructed earlier. * When the nTimeFirstKey is known to be inaccurate, it is set to the value 1 (instead of 0, which would mean unknown). * Use CPubKey instead of std::vector<unsigned char> where possible.metaverse
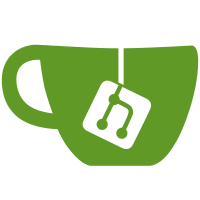
4 changed files with 36 additions and 28 deletions
Loading…
Reference in new issue