forked from hush/hush3
Browse Source
Port over https://github.com/chronokings/huntercoin/pull/19 from Huntercoin: This implements a new RPC command "getchaintips" that can be used to find all currently active chain heads. This is similar to the -printblocktree startup option, but it can be used without restarting just via the RPC interface on a running daemon.metaverse
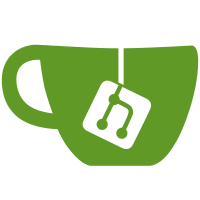
6 changed files with 100 additions and 4 deletions
@ -0,0 +1,24 @@ |
|||
#!/usr/bin/env python |
|||
# Copyright (c) 2014 The Bitcoin Core developers |
|||
# Distributed under the MIT/X11 software license, see the accompanying |
|||
# file COPYING or http://www.opensource.org/licenses/mit-license.php. |
|||
|
|||
# Exercise the getchaintips API. |
|||
|
|||
# Since the test framework does not generate orphan blocks, we can |
|||
# unfortunately not check for them! |
|||
|
|||
from test_framework import BitcoinTestFramework |
|||
from util import assert_equal |
|||
|
|||
class GetChainTipsTest (BitcoinTestFramework): |
|||
|
|||
def run_test (self, nodes): |
|||
res = nodes[0].getchaintips () |
|||
assert_equal (len (res), 1) |
|||
res = res[0] |
|||
assert_equal (res['branchlen'], 0) |
|||
assert_equal (res['height'], 200) |
|||
|
|||
if __name__ == '__main__': |
|||
GetChainTipsTest ().main () |
Loading…
Reference in new issue