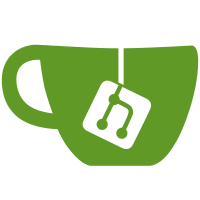
15 changed files with 211 additions and 123 deletions
@ -0,0 +1,64 @@ |
|||
#include "datamodel.h" |
|||
|
|||
DataModel::DataModel() { |
|||
lock = new QReadWriteLock(); |
|||
|
|||
// Write lock because we're creating everything
|
|||
QWriteLocker locker(lock); |
|||
|
|||
utxos = new QList<UnspentOutput>(); |
|||
balances = new QMap<QString, double>(); |
|||
usedAddresses = new QMap<QString, bool>(); |
|||
zaddresses = new QList<QString>(); |
|||
taddresses = new QList<QString>(); |
|||
} |
|||
|
|||
DataModel::~DataModel() { |
|||
delete lock; |
|||
|
|||
delete utxos; |
|||
delete balances; |
|||
delete usedAddresses; |
|||
delete zaddresses; |
|||
delete taddresses; |
|||
} |
|||
|
|||
void DataModel::replaceZaddresses(QList<QString>* newZ) { |
|||
QWriteLocker locker(lock); |
|||
Q_ASSERT(newZ); |
|||
|
|||
delete zaddresses; |
|||
zaddresses = newZ; |
|||
} |
|||
|
|||
|
|||
void DataModel::replaceTaddresses(QList<QString>* newT) { |
|||
QWriteLocker locker(lock); |
|||
Q_ASSERT(newT); |
|||
|
|||
delete taddresses; |
|||
taddresses = newT; |
|||
} |
|||
|
|||
void DataModel::replaceBalances(QMap<QString, double>* newBalances) { |
|||
QWriteLocker locker(lock); |
|||
Q_ASSERT(newBalances); |
|||
|
|||
delete balances; |
|||
balances = newBalances; |
|||
} |
|||
|
|||
|
|||
void DataModel::replaceUTXOs(QList<UnspentOutput>* newutxos) { |
|||
QWriteLocker locker(lock); |
|||
Q_ASSERT(newutxos); |
|||
|
|||
delete utxos; |
|||
utxos = newutxos; |
|||
} |
|||
|
|||
void DataModel::markAddressUsed(QString address) { |
|||
QWriteLocker locker(lock); |
|||
|
|||
usedAddresses->insert(address, true); |
|||
} |
@ -0,0 +1,48 @@ |
|||
#ifndef DATAMODEL_H |
|||
#define DATAMODEL_H |
|||
|
|||
#include "precompiled.h" |
|||
|
|||
|
|||
struct UnspentOutput { |
|||
QString address; |
|||
QString txid; |
|||
QString amount; |
|||
int confirmations; |
|||
bool spendable; |
|||
}; |
|||
|
|||
|
|||
// Data class that holds all the data about the wallet.
|
|||
class DataModel { |
|||
public: |
|||
void replaceZaddresses(QList<QString>* newZ); |
|||
void replaceTaddresses(QList<QString>* newZ); |
|||
void replaceBalances(QMap<QString, double>* newBalances); |
|||
void replaceUTXOs(QList<UnspentOutput>* utxos); |
|||
|
|||
void markAddressUsed(QString address); |
|||
|
|||
const QList<QString> getAllZAddresses() { QReadLocker locker(lock); return *zaddresses; } |
|||
const QList<QString> getAllTAddresses() { QReadLocker locker(lock); return *taddresses; } |
|||
const QList<UnspentOutput> getUTXOs() { QReadLocker locker(lock); return *utxos; } |
|||
const QMap<QString, double> getAllBalances() { QReadLocker locker(lock); return *balances; } |
|||
const QMap<QString, bool> getUsedAddresses() { QReadLocker locker(lock); return *usedAddresses; } |
|||
|
|||
|
|||
DataModel(); |
|||
~DataModel(); |
|||
private: |
|||
|
|||
|
|||
QList<UnspentOutput>* utxos = nullptr; |
|||
QMap<QString, double>* balances = nullptr; |
|||
QMap<QString, bool>* usedAddresses = nullptr; |
|||
QList<QString>* zaddresses = nullptr; |
|||
QList<QString>* taddresses = nullptr; |
|||
|
|||
QReadWriteLock* lock; |
|||
|
|||
}; |
|||
|
|||
#endif // DATAMODEL_H
|
Loading…
Reference in new issue