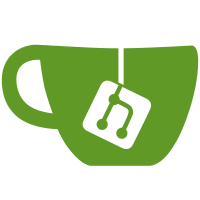
9 changed files with 0 additions and 491 deletions
@ -1,105 +0,0 @@ |
|||
#include "turnstile.h" |
|||
#include "mainwindow.h" |
|||
#include "balancestablemodel.h" |
|||
#include "controller.h" |
|||
#include "settings.h" |
|||
#include "ui_migration.h" |
|||
|
|||
|
|||
using json = nlohmann::json; |
|||
|
|||
// Need at least 0.0005 ZEC for this
|
|||
double Turnstile::minMigrationAmount = 0.0005; |
|||
|
|||
// Methods for zcashd native Migration
|
|||
void Turnstile::showZcashdMigration(MainWindow* parent) { |
|||
// If it is not enabled, don't show the dialog
|
|||
if (! parent->getRPC()->getMigrationStatus()->available) |
|||
return; |
|||
|
|||
Ui_MigrationDialog md; |
|||
QDialog d(parent); |
|||
md.setupUi(&d); |
|||
Settings::saveRestore(&d); |
|||
|
|||
MigrationTxns model(md.tblTxids, parent->getRPC()->getMigrationStatus()->txids); |
|||
md.tblTxids->setModel(&model); |
|||
|
|||
// Table right click
|
|||
md.tblTxids->setContextMenuPolicy(Qt::CustomContextMenu); |
|||
QObject::connect(md.tblTxids, &QTableView::customContextMenuRequested, [=, &model] (QPoint pos) { |
|||
QModelIndex index = md.tblTxids->indexAt(pos); |
|||
if (index.row() < 0) return; |
|||
|
|||
QMenu menu(parent); |
|||
QString txid = model.getTxid(index.row()); |
|||
|
|||
menu.addAction("Copy txid", [=]() { |
|||
QGuiApplication::clipboard()->setText(txid); |
|||
}); |
|||
|
|||
menu.addAction(QObject::tr("View on block explorer"), [=] () { |
|||
QString url; |
|||
if (Settings::getInstance()->isTestnet()) { |
|||
url = "https://explorer.testnet.z.cash/tx/" + txid; |
|||
} else { |
|||
url = "https://explorer.zcha.in/transactions/" + txid; |
|||
} |
|||
QDesktopServices::openUrl(QUrl(url)); |
|||
}); |
|||
|
|||
menu.exec(md.tblTxids->viewport()->mapToGlobal(pos)); |
|||
}); |
|||
|
|||
auto* status = parent->getRPC()->getMigrationStatus(); |
|||
|
|||
md.chkEnabled->setChecked(status->enabled); |
|||
md.lblSaplingAddress->setText(status->saplingAddress); |
|||
md.lblUnMigrated->setText(Settings::getZECDisplayFormat(status->unmigrated)); |
|||
md.lblMigrated->setText(Settings::getZECDisplayFormat(status->migrated)); |
|||
|
|||
if (d.exec() == QDialog::Accepted) { |
|||
// Update the migration status if it changed
|
|||
if (md.chkEnabled->isChecked() != status->enabled) { |
|||
parent->getRPC()->setMigrationStatus(md.chkEnabled->isChecked()); |
|||
} |
|||
} |
|||
} |
|||
|
|||
|
|||
MigrationTxns::MigrationTxns(QTableView *parent, QList<QString> txids) |
|||
: QAbstractTableModel(parent) { |
|||
headers << tr("Migration Txids"); |
|||
this->txids = txids; |
|||
} |
|||
|
|||
|
|||
int MigrationTxns::rowCount(const QModelIndex&) const { |
|||
return txids.size(); |
|||
} |
|||
|
|||
int MigrationTxns::columnCount(const QModelIndex&) const { |
|||
return headers.size(); |
|||
} |
|||
|
|||
QString MigrationTxns::getTxid(int row) const { |
|||
return txids.at(row); |
|||
} |
|||
|
|||
QVariant MigrationTxns::data(const QModelIndex &index, int role) const { |
|||
if (role == Qt::DisplayRole) { |
|||
switch(index.column()) { |
|||
case 0: return txids.at(index.row()); |
|||
} |
|||
} |
|||
return QVariant(); |
|||
} |
|||
|
|||
|
|||
QVariant MigrationTxns::headerData(int section, Qt::Orientation orientation, int role) const { |
|||
if (role == Qt::DisplayRole && orientation == Qt::Horizontal) { |
|||
return headers.at(section); |
|||
} |
|||
|
|||
return QVariant(); |
|||
} |
@ -1,37 +0,0 @@ |
|||
#ifndef TURNSTILE_H |
|||
#define TURNSTILE_H |
|||
|
|||
#include "precompiled.h" |
|||
|
|||
class MainWindow; |
|||
|
|||
class Turnstile |
|||
{ |
|||
public: |
|||
static void showZcashdMigration(MainWindow* parent); |
|||
|
|||
static double minMigrationAmount; |
|||
}; |
|||
|
|||
|
|||
// Classes for zcashd 2.0.5 native migration
|
|||
class MigrationTxns : public QAbstractTableModel { |
|||
|
|||
public: |
|||
MigrationTxns(QTableView* parent, QList<QString> txids); |
|||
~MigrationTxns() = default; |
|||
|
|||
int rowCount(const QModelIndex &parent) const; |
|||
int columnCount(const QModelIndex &parent) const; |
|||
QVariant data(const QModelIndex &index, int role) const; |
|||
QVariant headerData(int section, Qt::Orientation orientation, int role) const; |
|||
|
|||
QString getTxid(int row) const; |
|||
|
|||
private: |
|||
QList<QString> txids; |
|||
QStringList headers; |
|||
}; |
|||
|
|||
|
|||
#endif |
@ -1,235 +0,0 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<ui version="4.0"> |
|||
<class>Turnstile</class> |
|||
<widget class="QDialog" name="Turnstile"> |
|||
<property name="geometry"> |
|||
<rect> |
|||
<x>0</x> |
|||
<y>0</y> |
|||
<width>565</width> |
|||
<height>416</height> |
|||
</rect> |
|||
</property> |
|||
<property name="windowTitle"> |
|||
<string>Turnstile Migration</string> |
|||
</property> |
|||
<layout class="QVBoxLayout" name="verticalLayout"> |
|||
<item> |
|||
<widget class="QGroupBox" name="groupBox"> |
|||
<property name="title"> |
|||
<string>Turnstile Migration</string> |
|||
</property> |
|||
<layout class="QVBoxLayout" name="verticalLayout_2"> |
|||
<item> |
|||
<layout class="QGridLayout" name="gridLayout"> |
|||
<item row="0" column="0"> |
|||
<widget class="QLabel" name="msgIcon"> |
|||
<property name="text"> |
|||
<string/> |
|||
</property> |
|||
<property name="alignment"> |
|||
<set>Qt::AlignCenter</set> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="5" column="0"> |
|||
<widget class="QLabel" name="label_2"> |
|||
<property name="sizePolicy"> |
|||
<sizepolicy hsizetype="Minimum" vsizetype="Preferred"> |
|||
<horstretch>0</horstretch> |
|||
<verstretch>0</verstretch> |
|||
</sizepolicy> |
|||
</property> |
|||
<property name="text"> |
|||
<string>Migrate over</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="2" column="0"> |
|||
<widget class="QLabel" name="label"> |
|||
<property name="sizePolicy"> |
|||
<sizepolicy hsizetype="Minimum" vsizetype="Preferred"> |
|||
<horstretch>0</horstretch> |
|||
<verstretch>0</verstretch> |
|||
</sizepolicy> |
|||
</property> |
|||
<property name="text"> |
|||
<string>From</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="2" column="1" colspan="2"> |
|||
<widget class="AddressCombo" name="migrateZaddList"> |
|||
<property name="sizePolicy"> |
|||
<sizepolicy hsizetype="Expanding" vsizetype="Fixed"> |
|||
<horstretch>0</horstretch> |
|||
<verstretch>0</verstretch> |
|||
</sizepolicy> |
|||
</property> |
|||
<property name="editable"> |
|||
<bool>false</bool> |
|||
</property> |
|||
<property name="currentText"> |
|||
<string/> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="5" column="1" colspan="2"> |
|||
<widget class="QComboBox" name="privLevel"> |
|||
<property name="sizePolicy"> |
|||
<sizepolicy hsizetype="Expanding" vsizetype="Fixed"> |
|||
<horstretch>0</horstretch> |
|||
<verstretch>0</verstretch> |
|||
</sizepolicy> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="0" column="1" colspan="2"> |
|||
<widget class="QLabel" name="label_8"> |
|||
<property name="text"> |
|||
<string><html><head/><body><p>Funds from Sprout z-Addresses (which start with &quot;zc&quot;) need to be moved to the upgraded Sapling z-Addresses (which start with &quot;zs&quot;). The funds cannot be moved directly, but need to be sent through intermediate &quot;transparent&quot; addresses in privacy-preserving way.</p><p>This migration can be done automatically for you.</p></body></html></string> |
|||
</property> |
|||
<property name="wordWrap"> |
|||
<bool>true</bool> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="4" column="0"> |
|||
<widget class="QLabel" name="label_9"> |
|||
<property name="sizePolicy"> |
|||
<sizepolicy hsizetype="Minimum" vsizetype="Preferred"> |
|||
<horstretch>0</horstretch> |
|||
<verstretch>0</verstretch> |
|||
</sizepolicy> |
|||
</property> |
|||
<property name="text"> |
|||
<string>To</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="4" column="1" colspan="2"> |
|||
<widget class="AddressCombo" name="migrateTo"/> |
|||
</item> |
|||
<item row="1" column="0" colspan="3"> |
|||
<widget class="Line" name="line"> |
|||
<property name="orientation"> |
|||
<enum>Qt::Horizontal</enum> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="3" column="1"> |
|||
<widget class="QLabel" name="fromBalance"> |
|||
<property name="text"> |
|||
<string>Balance</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="7" column="0" rowspan="2" colspan="3"> |
|||
<spacer name="verticalSpacer"> |
|||
<property name="orientation"> |
|||
<enum>Qt::Vertical</enum> |
|||
</property> |
|||
<property name="sizeHint" stdset="0"> |
|||
<size> |
|||
<width>20</width> |
|||
<height>40</height> |
|||
</size> |
|||
</property> |
|||
</spacer> |
|||
</item> |
|||
<item row="6" column="0"> |
|||
<widget class="QLabel" name="label_5"> |
|||
<property name="sizePolicy"> |
|||
<sizepolicy hsizetype="Minimum" vsizetype="Preferred"> |
|||
<horstretch>0</horstretch> |
|||
<verstretch>0</verstretch> |
|||
</sizepolicy> |
|||
</property> |
|||
<property name="text"> |
|||
<string>Miner Fees</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="6" column="1" colspan="2"> |
|||
<widget class="QLabel" name="minerFee"> |
|||
<property name="sizePolicy"> |
|||
<sizepolicy hsizetype="Expanding" vsizetype="Preferred"> |
|||
<horstretch>0</horstretch> |
|||
<verstretch>0</verstretch> |
|||
</sizepolicy> |
|||
</property> |
|||
<property name="text"> |
|||
<string notr="true">0.0004 ZEC $0.04</string> |
|||
</property> |
|||
<property name="alignment"> |
|||
<set>Qt::AlignLeading|Qt::AlignLeft|Qt::AlignVCenter</set> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
<item row="3" column="0"> |
|||
<widget class="QLabel" name="label_3"> |
|||
<property name="text"> |
|||
<string>Total Balance</string> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
</layout> |
|||
</item> |
|||
</layout> |
|||
</widget> |
|||
</item> |
|||
<item> |
|||
<widget class="QDialogButtonBox" name="buttonBox"> |
|||
<property name="orientation"> |
|||
<enum>Qt::Horizontal</enum> |
|||
</property> |
|||
<property name="standardButtons"> |
|||
<set>QDialogButtonBox::Cancel|QDialogButtonBox::Ok</set> |
|||
</property> |
|||
</widget> |
|||
</item> |
|||
</layout> |
|||
</widget> |
|||
<customwidgets> |
|||
<customwidget> |
|||
<class>AddressCombo</class> |
|||
<extends>QComboBox</extends> |
|||
<header>addresscombo.h</header> |
|||
</customwidget> |
|||
</customwidgets> |
|||
<resources/> |
|||
<connections> |
|||
<connection> |
|||
<sender>buttonBox</sender> |
|||
<signal>accepted()</signal> |
|||
<receiver>Turnstile</receiver> |
|||
<slot>accept()</slot> |
|||
<hints> |
|||
<hint type="sourcelabel"> |
|||
<x>248</x> |
|||
<y>254</y> |
|||
</hint> |
|||
<hint type="destinationlabel"> |
|||
<x>157</x> |
|||
<y>274</y> |
|||
</hint> |
|||
</hints> |
|||
</connection> |
|||
<connection> |
|||
<sender>buttonBox</sender> |
|||
<signal>rejected()</signal> |
|||
<receiver>Turnstile</receiver> |
|||
<slot>reject()</slot> |
|||
<hints> |
|||
<hint type="sourcelabel"> |
|||
<x>316</x> |
|||
<y>260</y> |
|||
</hint> |
|||
<hint type="destinationlabel"> |
|||
<x>286</x> |
|||
<y>274</y> |
|||
</hint> |
|||
</hints> |
|||
</connection> |
|||
</connections> |
|||
</ui> |
Loading…
Reference in new issue